Node.js Basics: Your First Steps into Server-Side JavaScript
A beginner-friendly guide to Node.js, the powerful JavaScript runtime environment that lets you build fast, scalable backend applications.
This overview introduces the core ideas behind Node.js: running JavaScript outside the browser, its event-driven architecture, non-blocking I/O, and the versatile Node Package Manager (NPM).
Key takeaways cover Node.js's suitability for building APIs, real-time apps, and microservices, emphasizing its asynchronous nature and vast ecosystem of modules.
1. What is Node.js? (Beyond the Browser)
This section defines Node.js and explains its primary purpose: enabling JavaScript execution on the server-side.
Objectively, Node.js is an open-source, cross-platform JavaScript runtime environment. It allows developers to run JavaScript code outside of a web browser, primarily for building backend services and applications.
Delving deeper, Node.js is built on Google's V8 JavaScript engine, the same high-performance engine used in the Chrome browser. This means it executes JavaScript code very quickly.
Further considerations highlight its key design philosophy: event-driven, non-blocking I/O. This makes Node.js particularly well-suited for building applications that need to handle many concurrent connections efficiently, such as web servers, APIs, and real-time applications.
For years, JavaScript was primarily confined to running within web browsers to manipulate web pages. Node.js changed that. Created by Ryan Dahl in 2009, Node.js is:
- A JavaScript Runtime Environment: It provides the necessary components to execute JavaScript code directly on your computer or server, without needing a browser.
- Built on Chrome's V8 Engine: It leverages the powerful and fast V8 engine developed by Google for Chrome, ensuring high performance for JavaScript execution.
- Server-Side Focused: While versatile, its most common use case is building the backend (server-side) logic for web applications, APIs, and other network services.
- Open-Source & Cross-Platform: It's free to use and runs on various operating systems (Windows, macOS, Linux).
Node.js allows developers to use a single language (JavaScript) for both front-end and back-end development, simplifying the development stack (known as full-stack JavaScript).
Node.js: JavaScript Outside the Browser (Conceptual)
(Placeholder: Diagram showing Browser JS vs. Server-Side Node.js)
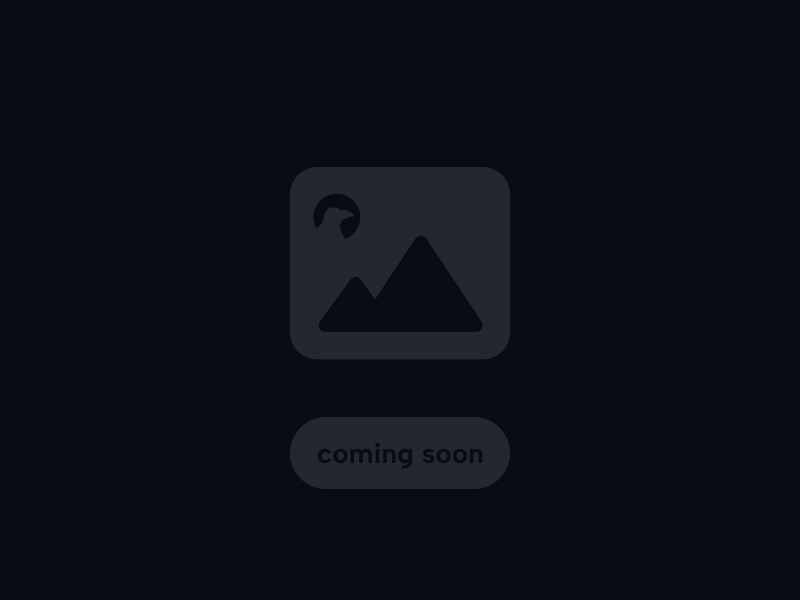
2. Core Concepts: Event Loop, Non-Blocking I/O
This section explains the fundamental architectural principles that make Node.js efficient for handling concurrent operations.
Objectively, Node.js operates on a single main thread but achieves concurrency through an event-driven model and non-blocking Input/Output (I/O) operations.
Delving deeper: * Event Loop: Node.js uses an event loop that constantly checks a queue for pending events (like incoming requests or completed I/O operations). When an event occurs, its associated callback function is executed. * Non-Blocking I/O: Instead of waiting for slow I/O operations (like reading a file or querying a database) to complete and blocking the main thread, Node.js delegates these tasks to the underlying system. It registers a callback function to be executed *after* the I/O operation finishes, allowing the event loop to continue processing other events in the meantime. * Asynchronous Nature: This non-blocking approach makes Node.js inherently asynchronous. Operations don't necessarily complete in the order they were started; results are handled via callbacks, Promises, or async/await when they become available.
Further considerations highlight that this model allows a single Node.js process to handle thousands of concurrent connections efficiently without needing many threads, which consumes fewer resources compared to traditional multi-threaded server models.
Node.js's efficiency, especially for I/O-intensive applications, stems from its core architecture:
- Single-Threaded Event Loop: Unlike traditional servers that might create a new thread for each connection (which can be resource-intensive), Node.js typically runs your JavaScript code on a single main thread.
- Event-Driven Architecture: Node.js applications listen for events (like an incoming HTTP request, a timer expiring, or a file operation completing). When an event occurs, a corresponding callback function is triggered and added to an event queue.
- The Event Loop: This is the heart of Node.js. It's a constantly running process that checks the event queue. If the main thread's call stack is empty, the event loop takes the next event from the queue and executes its associated callback function.
- Non-Blocking I/O: This is crucial. When Node.js encounters an operation that might take time (like reading a file from disk, making a database query, or handling a network request), it doesn't wait for it to finish. Instead:
- It initiates the operation.
- It registers a callback function to be executed *when* the operation completes.
- It immediately moves on to the next task, keeping the event loop free to handle other events.
This non-blocking, asynchronous model allows Node.js to handle many connections concurrently with high throughput using relatively few resources, making it ideal for real-time applications and APIs.
Node.js Event Loop (Simplified Conceptual Diagram)
(Placeholder: Diagram showing Call Stack, Event Queue, Event Loop checking queue and pushing callbacks to stack)
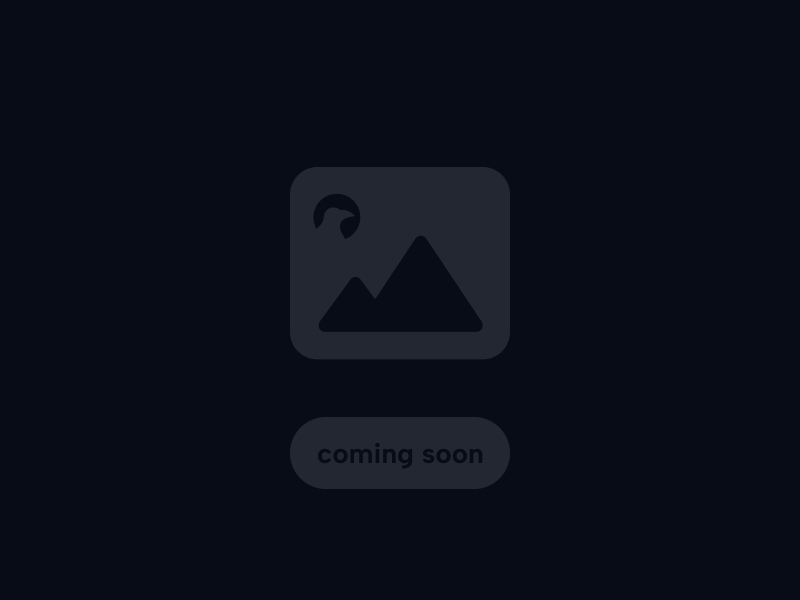
(Source: Conceptual Representation)
3. Setting Up Node.js & NPM Introduction
This section provides basic instructions on how to install Node.js and introduces its essential companion, NPM (Node Package Manager).
Objectively, installing Node.js is the first step. Official installers are available for Windows, macOS, and Linux from the nodejs.org website. Using a version manager like NVM (Node Version Manager) is often recommended for managing multiple Node.js versions.
Delving deeper: * Installation: Download the LTS (Long-Term Support) version from nodejs.org for stability, or the Current version for latest features. Follow the installer instructions. * Verification: Open your terminal or command prompt and type `node -v` and `npm -v` to verify installation and check the installed versions. * NPM (Node Package Manager): NPM is automatically installed with Node.js. It's the world's largest software registry and the command-line tool used to install and manage third-party packages (libraries/modules) for Node.js projects. * `npm init`: Running `npm init` in your project directory interactively creates a `package.json` file, which tracks project metadata and dependencies.
Further considerations include briefly mentioning the REPL (Read-Eval-Print Loop) accessed by simply typing `node` in the terminal for quick code testing.
Getting started with Node.js is easy:
- Download & Install Node.js:
- Go to the official Node.js website: https://nodejs.org/
- Download the installer for your operating system (Windows, macOS, Linux).
- It's generally recommended to download the LTS (Long-Term Support) version, which offers guaranteed stability and support for a longer period. The "Current" version has the latest features but might be less stable.
- Run the installer and follow the on-screen instructions.
- Verify Installation:
- Open your terminal (Command Prompt, PowerShell, Terminal).
- Type `node -v` and press Enter. You should see the installed Node.js version (e.g., `v18.18.0`).
- Type `npm -v` and press Enter. You should see the installed NPM version (e.g., `9.8.1`). NPM is bundled with Node.js.
Introducing NPM (Node Package Manager)
NPM is essential to the Node.js ecosystem. It serves two main purposes:
- It's an online registry hosting hundreds of thousands of reusable open-source code packages (libraries or modules) that you can use in your projects.
- It's a command-line tool for interacting with this registry, primarily for installing and managing dependencies (these external packages) for your project.
Initializing a Project (`npm init`)
When starting a new Node.js project, navigate to your project's directory in the terminal and run:
npm init
This command will prompt you for basic information about your project (name, version, description, entry point, etc.) and create a `package.json` file. This file is crucial for managing project metadata and dependencies installed via NPM.
You can also run `npm init -y` to accept all the defaults and create the `package.json` file immediately.
Node.js Installation Check (Terminal Output Concept)
(Placeholder: Image simulating terminal output showing `node -v` and `npm -v` commands with versions)
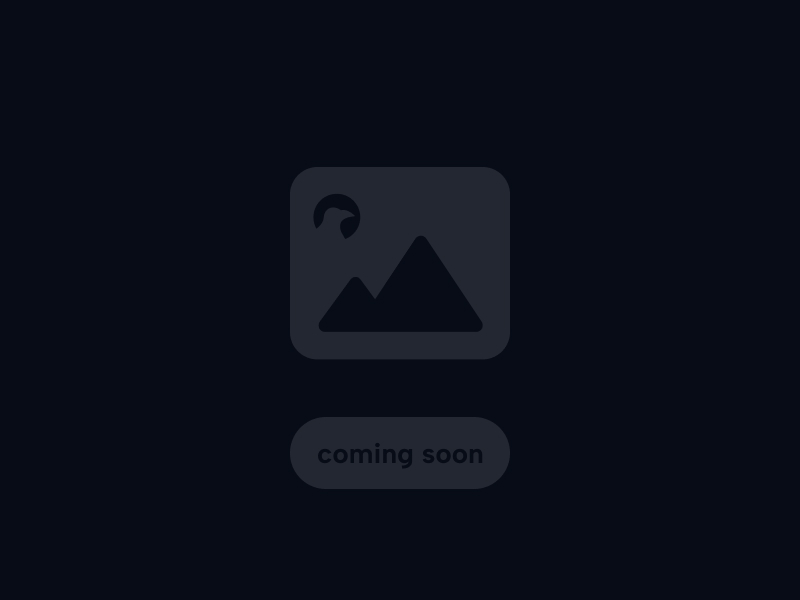
4. Node Modules: Core & NPM Packages
This section explains the module system in Node.js, covering both built-in core modules and third-party packages managed via NPM.
Objectively, Node.js uses a module system to organize code into reusable pieces. By default, it primarily uses the CommonJS module system (`require` and `module.exports`), although ES Modules (`import`/`export`) are increasingly supported.
Delving deeper:
* Core Modules: Node.js comes with built-in modules providing essential functionalities without needing installation (e.g., `http` for networking, `fs` for file system access, `path` for handling file paths). You load them using `require('module_name')`.
* NPM Packages (Third-Party Modules): The vast ecosystem of external libraries available through NPM. You install them using `npm install
Further considerations highlight how modules promote code reusability, organization, and leverage the vast open-source community via NPM.
Node.js applications are built using modules – reusable blocks of code. There are three main types:
- Core Modules: These are built directly into Node.js and provide fundamental functionalities. You don't need to install them separately. Examples include:
- `http`: For creating HTTP servers and clients.
- `https`: For creating HTTPS servers and clients.
- `fs` (File System): For interacting with the file system (reading/writing files).
- `path`: For handling and transforming file paths in a cross-platform way.
- `os`: For accessing operating system information.
- `events`: For working with the event emitter pattern.
- Local Modules: These are modules you create yourself within your project, typically as separate `.js` files. You export functionality using `module.exports` (CommonJS) or `export` (ES Modules) and import using `require('./myModule.js')` or `import ... from './myModule.js'`.
- Third-Party Modules (NPM Packages): These are modules created by the community and shared via the NPM registry. You install them into your project using the NPM command line tool:
- `npm install
` (e.g., `npm install express`) installs the package locally for your project. - `npm install -g
` installs the package globally (usually for command-line tools). - Installed packages are placed in a `node_modules` folder and usually added to your `package.json` dependencies.
- `npm install
`require` (CommonJS) vs. `import`/`export` (ES Modules)
Traditionally, Node.js used the CommonJS module system (`require`, `module.exports`). Modern JavaScript features ES Modules (`import`, `export`). Node.js now supports both, but you often need to configure your project (e.g., using `.mjs` file extension or setting `"type": "module"` in `package.json`) to use `import`/`export` syntax natively.
// Using a core module (CommonJS)
const fs = require('fs');
// Using a hypothetical local module (CommonJS)
// Assume myMath.js exists with: module.exports = { add: (a,b)=>a+b };
// const myMath = require('./myMath');
// console.log(myMath.add(2, 3)); // Output: 5
// Using an installed NPM package (CommonJS)
// Assume 'lodash' is installed: npm install lodash
// const _ = require('lodash');
// console.log(_.defaults({ 'a': 1 }, { 'a': 3, 'b': 2 })); // Output: { a: 1, b: 2 }
5. Creating a Simple HTTP Server
This section demonstrates one of Node.js's primary use cases: creating a basic web server using the built-in `http` module.
Objectively, the `http` core module provides functionality to create HTTP servers that can listen for incoming requests and send back responses.
Delving deeper into the process: 1. Require the `http` module: `const http = require('http');`. 2. Use `http.createServer()`: This method takes a callback function (request listener) that executes for each incoming request. This function receives two arguments: `request` (an object containing information about the incoming request) and `response` (an object used to send data back to the client). 3. Inside the callback: Set response headers (e.g., `response.writeHead(200, {'Content-Type': 'text/plain'});`) and send the response body (e.g., `response.end('Hello World\n');`). 4. Call `.listen()` on the created server object: Specify the port number and optional hostname to start listening for connections (e.g., `server.listen(3000, '127.0.0.1', () => { ... });`).
Further considerations mention that this is a very basic server; real-world applications typically use frameworks like Express.js built on top of the `http` module to simplify routing, middleware handling, and other common web server tasks.
One of the most common tasks for Node.js is building web servers or APIs. The built-in `http` module allows you to create a basic server relatively easily.
Here's a minimal example:
// 1. Import the http core module
const http = require('http');
// Define the hostname and port
const hostname = '127.0.0.1'; // Standard loopback address (localhost)
const port = 3000;
// 2. Create the server
// The function passed to createServer is the request listener.
// It runs every time a request hits the server.
const server = http.createServer((req, res) => {
// req: IncomingMessage object (request details like URL, method, headers)
// res: ServerResponse object (used to send response back)
// 3. Set the response status code and headers
res.statusCode = 200; // OK
res.setHeader('Content-Type', 'text/plain');
// 4. Write the response body and end the response
res.end('Hello World from Node.js!\n');
});
// 5. Start the server and make it listen on the specified port and hostname
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
To run this:
- Save the code in a file (e.g., `server.js`).
- Open your terminal in the same directory.
- Run the command: `node server.js`
- Open your web browser and navigate to `http://127.0.0.1:3000/` (or `http://localhost:3000/`).
You should see "Hello World from Node.js!" displayed. This demonstrates Node.js handling an HTTP request and sending a response.
While functional, building complex applications directly with the `http` module can be cumbersome. Frameworks like Express.js provide higher-level abstractions to simplify routing, middleware, request/response handling, and more.
6. Working with the File System (`fs` Module)
This section introduces Node.js's capabilities for interacting with the computer's file system using the built-in `fs` module.
Objectively, the `fs` module provides functions for performing various file system operations like reading files, writing files, checking file existence, creating directories, etc.
Delving deeper: * Asynchronous Operations: Most `fs` methods are asynchronous and non-blocking (e.g., `fs.readFile()`, `fs.writeFile()`). They accept a callback function that executes when the operation completes (either with data or an error). This is the preferred approach in Node.js to avoid blocking the event loop. * Synchronous Operations: For convenience in simple scripts or startup processes, synchronous versions are often available (e.g., `fs.readFileSync()`, `fs.writeFileSync()`). These block the event loop until the operation finishes and should generally be avoided in server applications handling concurrent requests. * Error Handling: Asynchronous methods typically pass an `error` object as the first argument to their callback function. Always check for errors.
Further considerations involve using the `path` module in conjunction with `fs` for constructing file paths reliably across different operating systems.
Node.js provides powerful tools for interacting with the file system through its core `fs` module. This allows your server-side JavaScript to read configuration files, write log files, serve static assets, and more.
Key characteristics of the `fs` module:
- Asynchronous by Default: Most file system operations can take time. To avoid blocking the single Node.js thread, the primary methods are asynchronous. They take a callback function as the last argument, which is executed once the operation completes. The first argument to the callback is typically an error object (or `null` if successful).
- Synchronous Alternatives: For many asynchronous methods, there's a synchronous counterpart (usually ending with `Sync`, e.g., `readFileSync`). These block execution until the operation completes. While sometimes useful for simple scripts or initial setup, avoid using synchronous methods in server applications handling multiple requests, as they will block the entire event loop.
- Error Handling: Always check for errors passed to the callback function in asynchronous operations.
const fs = require('fs');
const path = require('path'); // Core module for path manipulation
// --- Asynchronous File Reading ---
const filePathRead = path.join(__dirname, 'example.txt'); // Construct path safely
// Assume example.txt contains "Hello from file!"
fs.readFile(filePathRead, 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File content (async):', data);
// Output: File content (async): Hello from file!
});
console.log('Reading file asynchronously...'); // This logs first
// --- Asynchronous File Writing ---
const filePathWrite = path.join(__dirname, 'output.txt');
const contentToWrite = 'Writing this content!';
fs.writeFile(filePathWrite, contentToWrite, 'utf8', (err) => {
if (err) {
console.error('Error writing file:', err);
return;
}
console.log('File written successfully (async).');
});
console.log('Writing file asynchronously...'); // This logs while writing happens
// --- Synchronous File Reading (Use with caution!) ---
try {
const dataSync = fs.readFileSync(filePathRead, 'utf8');
console.log('File content (sync):', dataSync);
// Output: File content (sync): Hello from file!
} catch (err) {
console.error('Error reading file sync:', err);
}
console.log('After sync read attempt.'); // This logs only after sync read finishes
7. Understanding Asynchronous Programming in Node.js
This section revisits asynchronous programming patterns crucial for working effectively with Node.js's non-blocking nature.
Objectively, because Node.js relies on non-blocking I/O and the event loop, developers need ways to manage operations that don't complete immediately.
Delving deeper into common patterns: * Callbacks: The traditional Node.js approach where a function is passed as an argument to an asynchronous operation, to be executed upon completion. Can lead to nested code ("callback hell") for sequential operations. Error handling often follows the "error-first" convention (first callback argument is `err`). * Promises (ES6): Provide a cleaner way to handle asynchronous results using `.then()` for success and `.catch()` for errors, enabling better chaining for sequential tasks. Many modern Node.js APIs and libraries return Promises. * Async/Await (ES2017): Built on top of Promises, `async/await` offers syntactic sugar that allows writing asynchronous code that looks and behaves somewhat like synchronous code, making it much easier to read and reason about, especially for complex sequences. Use `async` before the function definition and `await` before a Promise-returning operation. Requires `try...catch` blocks for error handling.
Further considerations emphasize that mastering these patterns is essential for writing efficient, non-blocking, and maintainable Node.js applications.
Node.js's non-blocking, event-driven nature makes asynchronous programming fundamental. You need ways to handle operations that complete later without blocking the main thread.
- Callbacks:
- The original pattern used extensively in Node.js core modules (especially older ones).
- You pass a function (the callback) as an argument to an asynchronous function. This callback gets executed when the operation finishes.
- Often follows the "error-first" convention: `function(err, result) { ... }`. Check `err` first.
- Can lead to deeply nested code ("callback hell" or "pyramid of doom") when handling multiple sequential asynchronous operations.
// Callback Example (Conceptual - fs.readFile uses this) fs.readFile('file1.txt', 'utf8', (err1, data1) => { if (err1) return console.error(err1); console.log(data1); fs.readFile('file2.txt', 'utf8', (err2, data2) => { if (err2) return console.error(err2); console.log(data2); // ... potentially more nesting ... }); });
- Promises (ES6):
- An object representing the eventual result of an asynchronous operation.
- Use `.then()` to handle successful completion and `.catch()` to handle errors.
- Allows chaining operations more cleanly than nested callbacks.
- Many modern Node.js APIs (and libraries like `node-fetch` or promisified `fs` methods) return Promises.
// Promise Example (Conceptual using fs.promises) const fsPromises = require('fs').promises; fsPromises.readFile('file1.txt', 'utf8') .then(data1 => { console.log(data1); return fsPromises.readFile('file2.txt', 'utf8'); // Return next promise }) .then(data2 => { console.log(data2); }) .catch(err => { console.error('Error:', err); });
- Async/Await (ES2017):
- Syntactic sugar built on top of Promises. Makes asynchronous code look more like synchronous code.
- Use the `async` keyword before a function definition to indicate it contains asynchronous operations.
- Use the `await` keyword before a function call that returns a Promise. This pauses the `async` function's execution until the Promise settles (resolves or rejects).
- Use standard `try...catch` blocks for error handling with `await`.
- Generally the preferred method for modern asynchronous JavaScript/Node.js.
// Async/Await Example (Conceptual using fs.promises) const fsPromises = require('fs').promises; async function readFiles() { try { const data1 = await fsPromises.readFile('file1.txt', 'utf8'); console.log(data1); const data2 = await fsPromises.readFile('file2.txt', 'utf8'); console.log(data2); console.log("Both files read."); } catch (err) { console.error('Error:', err); } } readFiles();
Choosing the right pattern (preferably Promises or Async/Await for newer code) is key to effective Node.js development.
8. NPM Deep Dive: `package.json`, Dependencies & Scripts
This section takes a closer look at NPM (Node Package Manager) and the crucial `package.json` file for managing Node.js projects.
Objectively, `package.json` is the manifest file for any Node.js project. It contains metadata about the project and, importantly, lists its dependencies.
Delving deeper:
* `package.json`: Created by `npm init`. Contains project name, version, description, entry point (`main`), scripts, author, license, etc.
* Dependencies (`dependencies`): Packages required for the application to run in production (e.g., Express framework, database drivers). Installed using `npm install
Further considerations highlight how `package.json` and NPM facilitate reproducible builds and collaboration by clearly defining project requirements.
NPM is more than just an installer; it's central to managing your Node.js project's structure and dependencies via the `package.json` file.
- `package.json` - The Project Manifest:
- Created using `npm init`.
- Stores essential project metadata (name, version, description, license, author).
- Defines the main entry point file (often `index.js` or `server.js`).
- Lists project dependencies and devDependencies.
- Contains scripts for automating common tasks.
- Dependencies vs. DevDependencies:
- `dependencies`: Packages required for your application to run correctly in production (e.g., web framework like Express, database driver). Installed with `npm install
` or `npm i `. - `devDependencies`: Packages used only during development and testing (e.g., testing libraries like Jest or Mocha, linters like ESLint, bundlers like Webpack, utility tools like Nodemon). Installed with `npm install --save-dev
` or `npm i -D `. - Separating these helps optimize production deployments.
- `dependencies`: Packages required for your application to run correctly in production (e.g., web framework like Express, database driver). Installed with `npm install
- `node_modules` Folder:
- When you run `npm install`, NPM downloads the packages listed in `package.json` (and their dependencies) into this folder.
- Can become very large.
- Rule: Generally, you should add `node_modules/` to your `.gitignore` file and not commit it to version control. Other developers can simply run `npm install` to get the necessary dependencies based on `package.json` and `package-lock.json`.
- `package-lock.json`:
- Automatically generated or updated by `npm install`.
- Records the exact versions of every installed package and its dependencies that were used to create a working `node_modules` tree.
- Ensures that subsequent installs on different machines or at different times result in the exact same dependency tree, crucial for reproducible builds.
- Rule: You should commit `package-lock.json` to version control.
- NPM Scripts:
- The `"scripts"` section in `package.json` lets you define aliases for common command-line tasks.
- Example: `"scripts": { "start": "node server.js", "test": "jest" }`
- Run scripts using `npm run
` (e.g., `npm run start`). Common scripts like `start` and `test` can often be run as `npm start` and `npm test`.
`package.json` Structure (Conceptual)
(Placeholder: Text block showing key fields: name, version, main, scripts, dependencies, devDependencies)
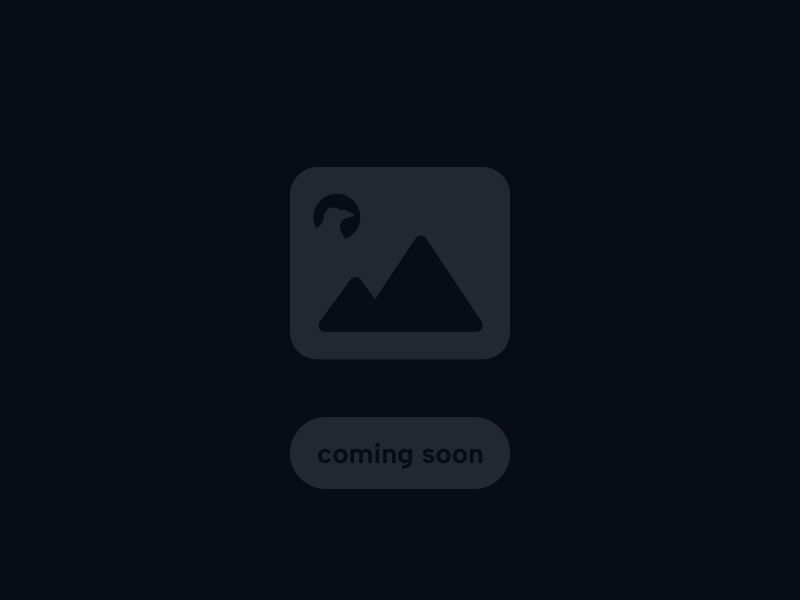
9. Common Use Cases for Node.js
This section highlights the types of applications and tasks where Node.js particularly excels due to its architecture.
Objectively, Node.js's event-driven, non-blocking I/O model makes it highly suitable for applications involving numerous concurrent connections and I/O operations.
Delving deeper into common use cases: * Web Servers & APIs: Building backend APIs (REST, GraphQL) for web and mobile applications. Frameworks like Express, Koa, Hapi, and NestJS are popular. * Real-time Applications: Chat applications, online gaming servers, collaborative tools, live dashboards – applications requiring persistent connections and real-time data flow, often using WebSockets (libraries like Socket.IO). * Microservices: Building small, independent backend services that communicate with each other, where Node.js's quick startup time and suitability for I/O-bound tasks are beneficial. * Build Tools & Development Workflow: Many popular web development tools (Webpack, Babel, ESLint, Prettier, task runners like Gulp) are built with Node.js. * Command-Line Interface (CLI) Tools: Creating custom command-line utilities for automation, development tasks, etc. * Data Streaming: Applications that process streams of data efficiently.
Further considerations mention that while Node.js can be used for CPU-intensive tasks (with worker threads), its primary strength lies in I/O-bound applications.
Thanks to its non-blocking I/O and event-driven nature, Node.js shines in several areas:
- Backend APIs (REST, GraphQL): This is arguably the most common use case. Node.js (often with frameworks like Express.js) is excellent for building fast, scalable APIs to serve data to web front-ends, mobile apps, or other services.
- Real-Time Applications: Its ability to handle many simultaneous connections efficiently makes it ideal for:
- Chat applications (using WebSockets via libraries like Socket.IO).
- Online multiplayer game backends.
- Live data dashboards and collaborative editing tools.
- Real-time tracking applications.
- Microservices: Node.js is a popular choice for building individual microservices within a larger distributed system due to its lightweight nature, fast startup times, and suitability for I/O-bound tasks.
- Single Page Application (SPA) Backends: Providing the server-side rendering (SSR) or API endpoints needed for modern front-end frameworks like React, Angular, and Vue.
- Streaming Applications: Efficiently handling streams of data, such as video streaming or real-time data processing pipelines.
- Command-Line Interface (CLI) Tools: Building cross-platform command-line tools for development workflows, automation, or system administration.
- Web Development Build Tools: Many essential tools used by front-end developers (like Webpack, Babel, ESLint, Gulp, Grunt) are built using Node.js and managed via NPM.
While Node.js can handle CPU-intensive tasks (especially with worker threads introduced in later versions), its core strength lies in efficiently managing numerous concurrent I/O operations.
Node.js Use Cases (Conceptual Icons)
(Placeholder: Icons: API, Chat Bubble (Real-time), Microservices (Puzzle), Tools (Wrench), CLI (Terminal))
10. Conclusion & Next Steps
This concluding section summarizes the fundamentals of Node.js and suggests resources for continued learning.
Objectively, Node.js is a powerful JavaScript runtime enabling server-side development. Its event-driven, non-blocking architecture, coupled with the vast NPM ecosystem, makes it a popular choice for building efficient and scalable network applications.
Delving deeper, mastering core concepts like the event loop, modules, asynchronous programming patterns (callbacks, Promises, async/await), and effectively using NPM are key to becoming proficient in Node.js development.
Further considerations encourage exploring popular Node.js frameworks like Express.js to simplify web application development and utilizing official documentation and community resources for ongoing learning and problem-solving.
Conclusion: Your Journey with Node.js Begins
Node.js has revolutionized backend development by bringing JavaScript to the server. Its unique event-driven, non-blocking I/O model makes it exceptionally well-suited for building fast, scalable network applications that handle many concurrent connections, such as APIs and real-time services. Understanding its core concepts, the module system, asynchronous programming patterns, and the indispensable NPM package manager are the foundational steps to leveraging its power.
This guide covered the basics, but the Node.js ecosystem is vast and constantly evolving. As you progress, exploring web frameworks, database integrations, testing strategies, and deployment techniques will be crucial next steps.
Node.js Learning Resources
Official Documentation:
- Node.js Official Website & Docs: nodejs.org/en/docs/ (Essential reference)
- NPM Official Website & Docs: docs.npmjs.com/ (For NPM commands and package info)
Tutorials & Learning Platforms:
- MDN Web Docs - Node.js Introduction: MDN Node.js Intro
- NodeSchool: nodeschool.io/ (Interactive command-line workshops)
- freeCodeCamp: Offers extensive backend development curriculum including Node.js.
- The Net Ninja, Traversy Media, Academind (Popular YouTube channels with Node.js tutorials).
Next Steps Considerations:
- Learn a Web Framework (like Express.js: expressjs.com)
- Database Integration (MongoDB, PostgreSQL, MySQL)
- Authentication & Authorization
- Testing Frameworks (Jest, Mocha)
- Deployment Strategies (Docker, Cloud Platforms like AWS, Azure, Heroku)
References (Placeholder)
Links to specific Node.js API docs could be included here.
- Node.js Documentation - HTTP Module
- Node.js Documentation - File System (fs) Module
- Node.js Documentation - Modules: CommonJS modules
- (Placeholder for articles on Event Loop, Non-Blocking I/O)
Node.js Basics Overview
(Placeholder: Simple graphic listing key topics: Runtime, Event Loop, NPM, Modules, HTTP, FS, Async)
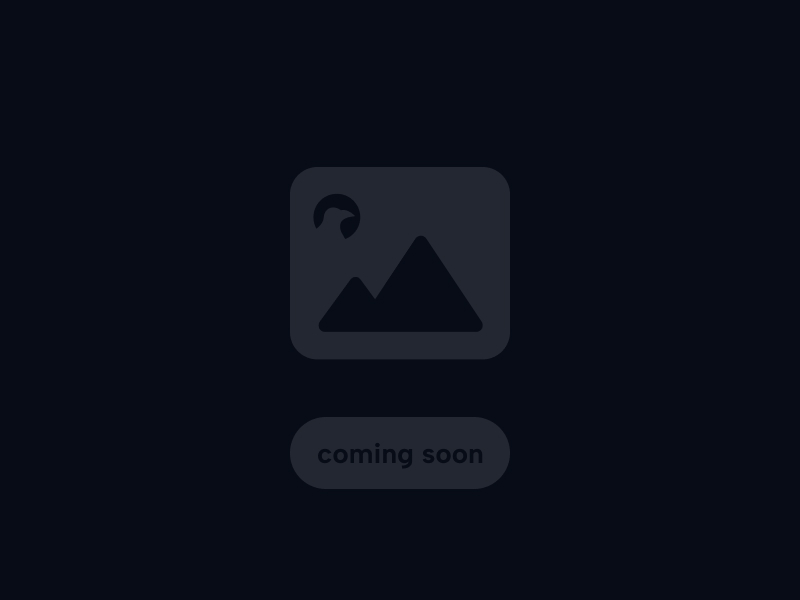