Command-Line Interface (CLI) Basics
Unlock the power of your computer with the command-line interface. This guide introduces the fundamental concepts and commands for beginners.
Learn what a CLI is, why it's essential for developers and power users, and how to perform basic tasks like navigating directories, managing files, and combining commands.
Key takeaways include understanding core commands like `ls`, `cd`, `mkdir`, `cp`, `mv`, `rm`, getting help with `man`, and using piping (`|`) and redirection (`>`).
1. What is a Command-Line Interface (CLI)?
A Command-Line Interface, or CLI, is a text-based way to interact with your computer's operating system or software applications.
Instead of clicking icons and menus in a Graphical User Interface (GUI), you type commands into a program called a terminal (or terminal emulator).
These commands are interpreted by another program called a shell (like Bash, Zsh, or PowerShell), which then executes the requested action.
- Terminal: The window or program where you type commands (e.g., Terminal on macOS/Linux, Windows Terminal, Command Prompt on Windows).
- Shell: The program running inside the terminal that interprets your commands and interacts with the operating system (e.g., Bash is the default shell on many Linux distributions and macOS).
- Command: A specific instruction given to the shell (e.g., `ls` to list files, `cd` to change directory).
- Prompt: The text displayed by the shell indicating it's ready for your next command (often ends with `$` or `>`).
Think of it as having a direct conversation with your computer using a specific language (the shell commands).
GUI vs. CLI (Conceptual)
(Placeholder: Simple graphic comparing a visual file explorer window with a text-based terminal window)

2. Why Use a Command-Line Interface?
While GUIs are often intuitive for basic tasks, CLIs offer significant advantages, especially for developers, system administrators, and power users:
- Speed & Efficiency: Typing commands can often be much faster than navigating through multiple menus and dialog boxes for experienced users.
- Automation & Scripting: You can easily combine multiple commands into scripts (e.g., shell scripts) to automate repetitive tasks. This is fundamental for DevOps and system administration.
- Access to Powerful Tools: Many essential development tools primarily operate via the command line (e.g., Git for version control, Docker for containerization, npm/yarn for package management, most compilers and build tools).
- Remote Access: CLIs are the standard way to manage remote servers via protocols like SSH (Secure Shell), as transmitting text is much more efficient than transmitting graphics.
- Resource Efficiency: Terminals and shells generally consume fewer system resources (CPU, RAM) than graphical applications.
- Fine-grained Control: Commands often provide more options and precise control over actions compared to their GUI counterparts.
- Composability: The ability to chain commands together using piping and redirection (see Section 7) allows for complex operations to be built from simple building blocks.
Learning the CLI opens up a vast world of powerful tools and efficient workflows that are often hidden behind graphical interfaces.
3. Common Terminals & Shells
While the terms are often used interchangeably, the terminal is the window, and the shell is the command interpreter running inside it.
Common Shells:
- Bash (Bourne Again SHell): The most common default shell on Linux and macOS. Most examples in this guide use Bash-compatible syntax.
- Zsh (Z Shell): A popular alternative to Bash, often used with frameworks like "Oh My Zsh" for enhanced features (autocompletion, themes). Mostly compatible with Bash for basic commands. Became the default shell in recent macOS versions.
- PowerShell: The modern, object-oriented shell for Windows. Its commands (cmdlets) have a different `Verb-Noun` structure (e.g., `Get-ChildItem` instead of `ls`), but it can also run many traditional commands. Available cross-platform.
- Command Prompt (cmd.exe): The legacy command-line interpreter on Windows. Has a different command set (e.g., `dir` instead of `ls`, `copy` instead of `cp`). Less powerful than PowerShell or Bash/Zsh.
- Fish (Friendly Interactive SHell): Known for user-friendly features like extensive autocompletion and syntax highlighting out-of-the-box.
Common Terminal Emulators:
- Terminal.app (macOS): Built-in terminal.
- GNOME Terminal, Konsole (Linux): Common defaults on Linux desktops.
- Windows Terminal: Modern Microsoft terminal supporting Command Prompt, PowerShell, and WSL (Windows Subsystem for Linux) shells in tabs. Recommended for Windows users.
- iTerm2 (macOS): Popular third-party terminal with many features.
- Hyper, Alacritty: Cross-platform, often customizable terminals.
For this guide, we'll focus on Bash-like commands as they are widely applicable (Linux, macOS, WSL on Windows).
5. File & Directory Operations
Creating, copying, moving, renaming, and deleting files and directories are common tasks.
-
mkdir
(Make Directory): Creates a new directory.mkdir new_project ls # Output now includes: new_project
-
touch
(Update Timestamp / Create Empty File): Creates an empty file if it doesn't exist, or updates the modification timestamp if it does.touch notes.txt ls # Output now includes: notes.txt
-
cp
(Copy): Copies files or directories.cp source_file destination_file
: Copies a file.cp file.txt backup.txt
: Creates a copy named `backup.txt`.cp file.txt existing_directory/
: Copies `file.txt` into `existing_directory`.cp -r source_directory/ destination_directory/
: Copies a directory and its contents recursively (`-r` flag is important).
cp notes.txt notes_backup.txt cp notes.txt new_project/ cp -r new_project/ project_archive/ # Assumes project_archive exists or will be created
-
mv
(Move / Rename): Moves files/directories or renames them.mv old_filename new_filename
: Renames a file or directory.mv file_to_move target_directory/
: Moves a file into a directory.mv directory_to_move target_directory/
: Moves a directory into another directory.
mv notes_backup.txt old_notes.txt # Rename mv old_notes.txt new_project/ # Move
-
rm
(Remove): Deletes files. **Use with caution! There is no undelete/trash bin by default.**rm filename.txt
: Deletes a file.rm -i filename.txt
: Interactive mode, prompts before deleting.rm -r directory_name/
: Deletes a directory and its contents recursively. **Very dangerous if used incorrectly.**rm -rf directory_name/
: Forcefully deletes a directory recursively without prompting. **EXTREMELY DANGEROUS.** Double-check before using.
touch temp_file.tmp rm temp_file.tmp mkdir temp_dir # rm temp_dir # This will usually error as rm deletes files # Use rmdir for empty dirs OR rm -r for non-empty
-
rmdir
(Remove Directory): Deletes an *empty* directory.mkdir empty_folder rmdir empty_folder
6. Getting Help
When you don't know how to use a command or forget its options, the CLI provides built-in help.
-
man
(Manual): Displays the manual page (detailed documentation) for a command.Navigate the `man` page using arrow keys, Page Up/Down. Press `q` to quit.
man ls # Shows the manual for the 'ls' command man cp # Shows the manual for the 'cp' command
-
--help
or-h
Flags: Many commands provide a brief summary of their usage and options when called with a help flag. The exact flag varies (`--help` is common, `-h` is a frequent short version).ls --help # Displays help for 'ls' mkdir --help # Displays help for 'mkdir' git --help # Displays help for the 'git' command
Using `man` pages and help flags is essential for learning new commands and understanding their capabilities without constantly searching the web.
7. Piping & Redirection
Two powerful concepts allow you to combine commands and manage their input/output:
-
Piping (
|
): Takes the standard output (stdout) of one command and sends it directly as the standard input (stdin) to another command. This lets you chain commands together.# List all files in long format, then filter for lines containing ".txt" ls -l | grep ".txt" # List running processes, then count how many lines there are ps aux | wc -l
-
Output Redirection (
>
and>>
): Sends the standard output (stdout) of a command to a file instead of the terminal screen.> filename
: Redirects output to `filename`. **Overwrites** the file if it already exists.>> filename
: Redirects output to `filename`. **Appends** to the file if it exists, or creates it if it doesn't.
# Save the list of files to files.txt (overwrites if exists) ls > files.txt # Add "Hello World" to greeting.txt (appends if exists) echo "Hello World" >> greeting.txt # Save error messages (stderr, channel 2) to errors.log some_command_that_might_fail 2> errors.log
-
Input Redirection (
<
): Takes input for a command from a file instead of the keyboard (less common in basic interactive use but important for scripting).# Sort the contents of names.txt sort < names.txt
Piping and redirection are fundamental for automating tasks and manipulating data efficiently on the command line.
8. Useful Utilities (Examples)
Beyond basic navigation and file operations, countless command-line utilities exist. Here are a couple of very common and useful examples:
-
grep
(Global Regular Expression Print): Searches for patterns (text or regular expressions) within files or input streams. Often used with piping.# Search for the word "error" in server.log grep "error" server.log # List files, then find lines containing "config" (case-insensitive) ls -l | grep -i "config"
-
find
: Searches for files and directories within a directory hierarchy based on various criteria (name, size, modification time, type, etc.).# Find all files named "README.md" starting from the current directory (.) find . -name "README.md" # Find all directories (-type d) modified in the last 2 days (-mtime -2) find . -type d -mtime -2 # Find all files larger than 1MB (+1M) and execute 'ls -lh' on them find . -type f -size +1M -exec ls -lh {} \;
-
echo
: Displays text or variables to the standard output. Useful for simple messages or creating file content via redirection.echo "Starting script..." echo "My home directory is: $HOME" # $HOME is an environment variable
-
cat
(Concatenate): Displays the contents of files. Can also combine files.cat file1.txt cat file1.txt file2.txt > combined.txt # Combine files
-
less
/more
: View the contents of large files one screen at a time. `less` is generally more powerful (allows backward scrolling). Use arrow keys/Page Up/Down to navigate, `q` to quit.less very_long_log_file.log
Exploring and learning common utilities like these significantly increases your command-line proficiency.
9. Conclusion & Next Steps
This guide has covered the absolute basics of the command-line interface. You've learned what a CLI is, why it's useful, how to navigate the file system, manage files and directories, get help, and use fundamental tools like piping, redirection, `grep`, and `find`.
The CLI is a vast and powerful tool. These fundamental commands are the building blocks for more complex operations and automation.
Next Steps:
- Practice Regularly: The best way to become comfortable is to use the CLI for everyday tasks (navigating, creating files, running development tools).
- Explore More Commands: Use `man` or search online for commands related to tasks you want to perform (e.g., text manipulation with `sed`/`awk`, process management with `ps`/`kill`, networking with `ping`/`curl`).
- Learn Basic Shell Scripting: Combine commands into simple `.sh` scripts to automate workflows.
- Customize Your Shell: Explore configuration options for Bash or Zsh (e.g., aliases, custom prompts, plugins via Oh My Zsh).
- Tool-Specific CLIs: Dive deeper into the CLIs for tools you use frequently, like Git (`git`), Docker (`docker`), and Node.js package managers (`npm`, `yarn`).
Key Takeaway:
Don't be intimidated! Start with the basics, practice consistently, and gradually explore more advanced features. The command line is an indispensable skill for efficient software development and system interaction.
Further Resources (Placeholder)
- LinuxCommand.org
- explainshell.com (Breaks down commands)
- Tutorials on specific shells (Bash, Zsh, PowerShell)
- Online courses on Linux/Unix fundamentals
Keep Learning!
(Placeholder: Simple graphic like an upward arrow or open book)
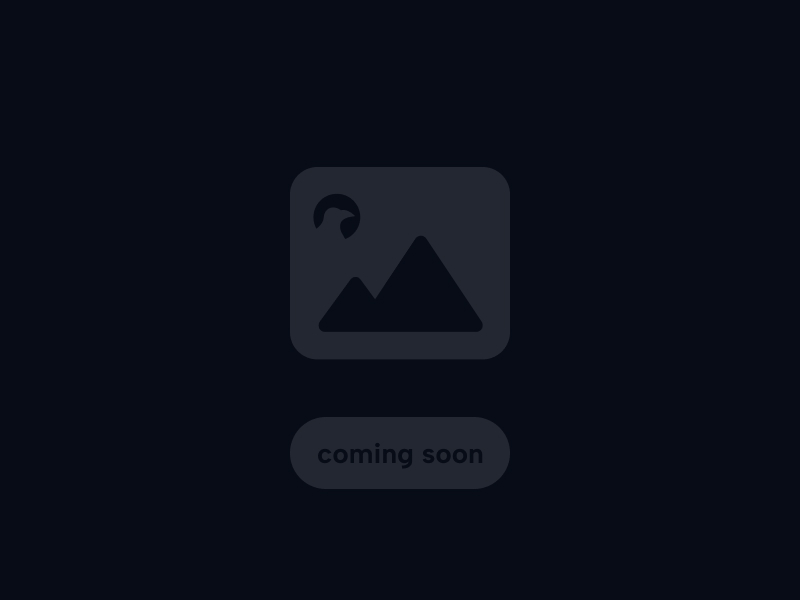