Connecting JavaScript to Databases: A Developer's Guide
Explore how JavaScript applications, both on the client and server (Node.js), interact with SQL and NoSQL databases to store, retrieve, and manage data effectively.
This overview covers the common patterns for database management in the JavaScript ecosystem, including direct connections via Node.js drivers, using ORMs/ODMs, client-side storage options, and interacting with databases through APIs.
Key takeaways focus on understanding different database types, choosing appropriate connection methods, leveraging tools like Sequelize and Mongoose, and prioritizing security in database operations.
1. Introduction: The Role of Databases & JavaScript's Interaction
This section introduces the fundamental need for databases in applications and outlines the different ways JavaScript code interacts with them.
Objectively, databases are organized collections of data, essential for storing, managing, and retrieving information efficiently in almost any application beyond the most trivial. JavaScript, being a dominant language in web development, frequently needs to interact with these databases.
Delving deeper, the interaction differs significantly based on context: * Client-Side JavaScript (in the Browser): Generally does not interact directly with server databases due to major security risks. Instead, it communicates with a server-side application (often via APIs) which then handles database operations. It *can* interact with browser-based storage mechanisms. * Server-Side JavaScript (e.g., Node.js): Can directly connect to and interact with databases using specific drivers or libraries. This is where most direct JavaScript-to-database management occurs.
Further considerations emphasize understanding this client-server separation and the security implications as crucial first steps before diving into specific database technologies.
Modern applications, from simple websites to complex enterprise systems, rely heavily on databases to persistently store and manage data (user information, product catalogs, posts, etc.). JavaScript, as the language of the web and a popular choice for server-side development (with Node.js), plays a key role in interacting with these databases.
However, how JavaScript interacts with a database depends heavily on where the JavaScript code is running:
- Client-Side JS (Running in the User's Browser): For security reasons, browser-based JavaScript almost never connects directly to a main application database (like PostgreSQL or MongoDB on a server). Imagine the chaos if any website visitor could directly access or modify your database! Instead, client-side JS typically:
- Sends requests to a server-side API (Application Programming Interface).
- Receives data *from* the API after the server has securely interacted with the database.
- Uses browser storage mechanisms (`localStorage`, `IndexedDB`) for small amounts of non-sensitive, temporary data.
- Server-Side JS (Running on a Server, e.g., using Node.js): This is where direct database interaction happens securely. Server-side JavaScript code can:
- Establish secure connections to various database systems (SQL and NoSQL).
- Execute queries to Create, Read, Update, and Delete (CRUD) data.
- Validate data and enforce business logic before interacting with the database.
- Expose data securely to the client-side via APIs.
This guide focuses primarily on how server-side JavaScript (Node.js) manages database interactions, while also touching upon client-side storage and the role of APIs.
Client-Server-Database Architecture (Conceptual)
(Placeholder: Diagram showing Browser(JS) -> API Request -> Server(Node.js) -> Database -> Server -> API Response -> Browser)
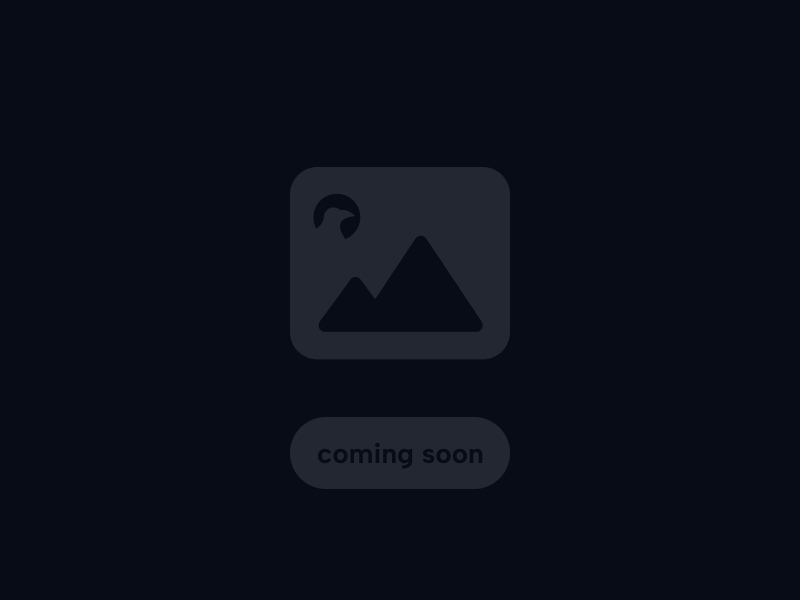
2. Database Types Overview (SQL vs. NoSQL)
This section provides a high-level overview of the two main categories of databases commonly used with JavaScript applications: SQL (Relational) and NoSQL.
Objectively, the choice between SQL and NoSQL depends on the application's data structure, scalability requirements, and consistency needs.
Delving deeper: * SQL (Relational Databases): * Structure: Data stored in tables with predefined schemas (rows and columns). Relationships defined between tables. * Language: Uses Structured Query Language (SQL) for querying. * Examples popular with JS/Node.js: PostgreSQL, MySQL, SQLite. * Strengths: Strong consistency (ACID properties), well-suited for structured data with complex relationships. * NoSQL (Non-Relational Databases): * Structure: More flexible data models. Common types include: * *Document:* Data stored in JSON-like documents (e.g., MongoDB). * *Key-Value:* Simple key-based lookups (e.g., Redis, Memcached). * *Column-Family:* Optimized for wide rows (e.g., Cassandra). * *Graph:* Focuses on relationships (e.g., Neo4j). * Language: Varies by database type; often have their own query APIs. * Examples popular with JS/Node.js: MongoDB (Document), Redis (Key-Value). * Strengths: High scalability, flexibility in schema, good for unstructured or semi-structured data.
Further considerations mention that JavaScript developers often work with both types, and the choice involves trade-offs.
JavaScript applications can work with various database systems, broadly categorized as SQL or NoSQL.
- SQL (Relational) Databases:
- Concept: Store data in structured tables with predefined columns and data types (like a spreadsheet). Relationships between tables are explicitly defined (e.g., a 'users' table linked to an 'orders' table).
- Query Language: Uses SQL (Structured Query Language) for defining schemas and manipulating data (e.g., `SELECT * FROM users WHERE country = 'Canada';`).
- Key Features: Emphasis on data integrity, consistency (ACID compliance), well-defined schemas.
- Popular Examples used with Node.js: PostgreSQL, MySQL, SQLite (for simpler/embedded use), Microsoft SQL Server.
- Best suited for: Applications requiring strong data consistency, complex queries involving multiple related tables, structured data.
- NoSQL (Non-Relational) Databases:
- Concept: Offer more flexible data models than traditional tables. They encompass various types:
- Document Databases: Store data in flexible, JSON-like documents (BSON in MongoDB's case). Schemas can vary between documents. (e.g., MongoDB)
- Key-Value Stores: Simple pairs of keys and values. Very fast for lookups. (e.g., Redis, Memcached)
- *(Others include Column-Family Stores and Graph Databases)*
- Query Language: Varies widely. Often use specific APIs or query methods provided by the database driver rather than standard SQL.
- Key Features: High scalability (horizontal scaling), schema flexibility, potentially higher performance for certain workloads (especially write-heavy or simple lookups). Consistency models can vary (often 'eventual consistency').
- Best suited for: Large datasets, applications needing high scalability and flexible schemas, unstructured or semi-structured data, real-time applications (like caching with Redis).
- Concept: Offer more flexible data models than traditional tables. They encompass various types:
The choice between SQL and NoSQL depends heavily on the specific needs of the application. JavaScript developers frequently encounter both, especially PostgreSQL and MySQL (SQL), and MongoDB (NoSQL Document DB).
3. Client-Side Storage with JavaScript
This section discusses storage mechanisms available directly within the user's web browser, manageable via client-side JavaScript, differentiating them from server-side databases.
Objectively, browsers provide APIs for storing data locally on the user's machine, useful for caching application state, user preferences, or offline data, but unsuitable for sensitive or large-scale persistent data.
Delving deeper into common browser storage options: * `localStorage`: Stores key-value pairs (strings only) persistently even after the browser is closed. Simple API, good for user preferences or small amounts of non-sensitive data. Limited storage size (typically 5-10MB). Synchronous access (can block main thread). * `sessionStorage`: Similar to `localStorage` (key-value strings) but data persists only for the duration of the browser *session* (until the tab/window is closed). Synchronous access. * `IndexedDB`: A more powerful, low-level asynchronous API for storing significant amounts of structured data client-side, including files/blobs. Acts like a client-side NoSQL database (object store). Can be complex to use directly, often used via wrapper libraries.
Further considerations emphasize the security risks (data is accessible/modifiable by the user), storage limitations, and the fact that this data is confined to the user's specific browser instance, unlike server databases accessible to all users.
While not traditional "database management," client-side JavaScript running in the browser has ways to store data locally on the user's computer. This is useful for things like remembering user preferences, storing application state, or enabling offline functionality, but it is not a replacement for a secure server-side database.
Key browser storage mechanisms:
- `localStorage`:
- What it stores: Key-value pairs, where both keys and values are strings. (You need `JSON.stringify()` to store objects and `JSON.parse()` to retrieve them).
- Persistence: Data persists even after the browser window is closed and reopened.
- Scope: Specific to the browser and the web origin (protocol, domain, port).
- Size Limit: Typically around 5-10MB per origin.
- API: Simple synchronous methods like `localStorage.setItem('key', 'value')`, `localStorage.getItem('key')`, `localStorage.removeItem('key')`. Synchronous nature can potentially block rendering if overused.
- Use Cases: Storing user preferences (e.g., theme choice), non-sensitive session info, simple application state.
- `sessionStorage`:
- What it stores: Same as `localStorage` (key-value strings).
- Persistence: Data persists only for the duration of the browser session. It's cleared when the tab or window is closed.
- Scope: Specific to the browser tab/window session and origin.
- Size Limit: Similar to `localStorage`.
- API: Same synchronous methods as `localStorage` (`sessionStorage.setItem()`, etc.).
- Use Cases: Storing temporary session data (e.g., user input in a multi-step form before submission).
- `IndexedDB`:
- What it stores: A much more powerful client-side database. Stores significant amounts of structured data, including complex JavaScript objects, files, and blobs. Uses object stores (like NoSQL tables) and indexes for efficient querying.
- Persistence: Persistent like `localStorage`.
- Scope: Specific to the origin.
- Size Limit: Much larger than localStorage/sessionStorage (can often be hundreds of MB or more, user permission might be required).
- API: Asynchronous API using events and requests. Can be complex to use directly; wrapper libraries often simplify it.
- Use Cases: Offline data storage for Progressive Web Apps (PWAs), caching large amounts of application data, complex client-side applications.
- *(Briefly: Cookies are another mechanism, primarily used for session management and tracking, sent with HTTP requests, not ideal for general client-side storage).*
Security Warning: Data stored client-side is accessible and potentially modifiable by the user via browser developer tools. Never store sensitive information (passwords, private keys) in client-side storage.
4. Server-Side JavaScript (Node.js) & Databases
This section explains why server-side JavaScript, particularly using the Node.js runtime environment, is the primary way JavaScript directly and securely interacts with databases.
Objectively, Node.js allows JavaScript code to run outside the browser, on a server, giving it access to the server's file system, network capabilities, and the ability to establish secure connections to database servers.
Delving deeper into the typical architecture: * Client Request: The user's browser (running client-side JS) sends an HTTP request to a server (e.g., asking for user data). * Server-Side Processing (Node.js): The Node.js application running on the server receives the request. * Database Interaction: The Node.js code uses appropriate database drivers or ORMs/ODMs to connect to the database server, execute queries (e.g., fetch user data based on request parameters), and receive results. * Server Response: The Node.js application processes the database results and sends an HTTP response (often containing data in JSON format) back to the client's browser. * Client Update: The client-side JS receives the response and updates the webpage accordingly.
Further considerations emphasize that this server layer acts as a secure intermediary, validating requests, enforcing business logic, and protecting database credentials, which should never be exposed to the client.
While client-side JavaScript handles user interface interactions, server-side JavaScript, most commonly powered by Node.js, is where the heavy lifting of interacting with databases securely occurs.
- Node.js Environment: Node.js is a runtime environment that allows you to execute JavaScript code on a server, outside the limitations of a web browser.
- Direct Database Access: Unlike browser JS, Node.js applications can directly install and use database drivers (specialized libraries) to connect to and communicate with various database systems (PostgreSQL, MongoDB, MySQL, etc.).
- Secure Connections: Node.js applications manage database connection credentials (usernames, passwords, connection strings) securely on the server, never exposing them to the client browser. Connections can be properly secured (e.g., using SSL/TLS).
- Typical Workflow (Web Application):
- User interacts with the webpage (Client-Side JS).
- Client-Side JS sends a request (e.g., using `Workspace`) to an API endpoint on the server.
- The server application (built with Node.js and frameworks like Express.js) receives the request.
- The Node.js code validates the request and performs necessary logic.
- Node.js code uses a database driver or ORM/ODM to connect to the database and execute a query (e.g., `SELECT user FROM users WHERE id = ?` or `db.collection('users').findOne({_id: ...})`).
- The database returns the results to the Node.js application.
- Node.js processes the results and sends a response (often JSON data) back to the client browser.
- Client-Side JS receives the response and updates the user interface.
Node.js provides the necessary environment and tools (like the Node Package Manager - npm - for installing database drivers) for JavaScript to become a powerful tool for full-stack development, including database management.
Node.js Interacting with Database (Conceptual)
(Placeholder: Diagram: Node.js App -> DB Driver/ORM -> Database Server)
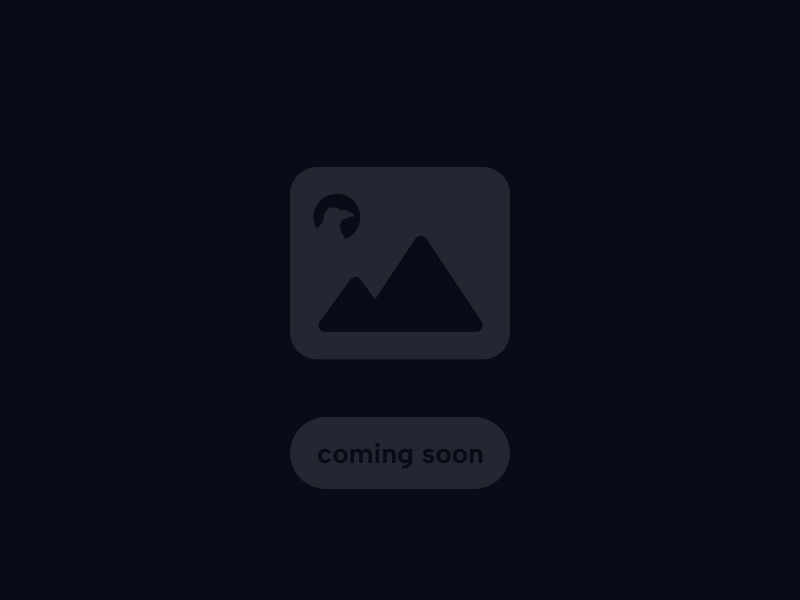
5. Connecting to SQL Databases with Node.js
This section focuses on the practical aspects of connecting server-side JavaScript (Node.js) applications to relational (SQL) databases like PostgreSQL or MySQL.
Objectively, this requires installing and using specific Node.js packages (database drivers) available via npm (Node Package Manager).
Delving deeper: * Install Driver: Use npm to install the appropriate driver, e.g., `npm install pg` for PostgreSQL or `npm install mysql2` for MySQL. * Establish Connection: Use the driver's API to create a connection or connection pool using database credentials (host, port, user, password, database name), typically stored securely in environment variables. * Execute Queries: Use methods provided by the driver to send SQL queries to the database (e.g., `client.query('SELECT * FROM users WHERE id = $1', [userId])`). * Parameterization: Crucially, use parameterized queries (placeholders like `$1`, `?`) instead of directly embedding user input into SQL strings to prevent SQL injection vulnerabilities. The driver handles safe substitution. * Handle Results: Process the results returned from the database (often involves asynchronous handling using Promises or async/await).
Further considerations mention connection pooling for managing multiple connections efficiently in web applications and the importance of closing connections properly.
To connect your Node.js application to a SQL database, you'll typically use a database driver specific to that database system, installed via npm.
General Steps (Conceptual - Syntax varies slightly between drivers):
- Install the Driver: Open your terminal in your Node.js project directory and run:
For PostgreSQL: `npm install pg`
For MySQL: `npm install mysql2` - Require the Library: In your JavaScript file, import the necessary library:
// For PostgreSQL const { Client, Pool } = require('pg'); // Or use import if using ES Modules // For MySQL // const mysql = require('mysql2/promise'); // Using the promise-based version
- Configure Connection: Set up connection details (host, user, password, database, port). Best practice: Store these securely using environment variables, not hardcoded in your source code.
// Example config for pg (using Pool for web apps) const pool = new Pool({ user: process.env.DB_USER, host: process.env.DB_HOST, database: process.env.DB_DATABASE, password: process.env.DB_PASSWORD, port: process.env.DB_PORT, });
- Connect & Execute Query: Use the driver's methods to connect (often handled by a pool) and run queries. Use Promises or `async/await` for asynchronous handling.
// Example with pg Pool using async/await async function getUser(userId) { let client; // Declare client outside try block try { client = await pool.connect(); // Get client from pool // Use Parameterized Query to prevent SQL injection! const queryText = 'SELECT name, email FROM users WHERE id = $1'; const result = await client.query(queryText, [userId]); return result.rows[0]; // Return the first user found } catch (err) { console.error('Database query error', err.stack); throw err; // Re-throw error } finally { if (client) { client.release(); // Release client back to the pool IMPORTANT! } } } // Example usage: // getUser(1).then(user => console.log(user)).catch(e => console.error(e));
Security is paramount: Always use parameterized queries (like `$1` in `pg` or `?` in `mysql2`) to safely insert variables into your SQL. Never concatenate user input directly into query strings, as this opens you up to SQL injection attacks.
6. Connecting to NoSQL Databases (MongoDB) with Node.js
This section focuses on connecting Node.js applications to a popular NoSQL document database, MongoDB.
Objectively, similar to SQL, connecting to MongoDB from Node.js requires a specific driver package installed via npm.
Delving deeper: * Install Driver: Use npm: `npm install mongodb`. * Establish Connection: Use the MongoDB driver's `MongoClient` to connect to the database instance using a connection string (URI), often stored in environment variables. * Select Database & Collection: Once connected, select the specific database and collection (similar to a table in SQL) you want to work with. * Perform CRUD Operations: Use driver methods like `insertOne()`, `find()`, `findOne()`, `updateOne()`, `deleteOne()` to interact with documents in the collection. These methods typically return Promises. * Querying: MongoDB uses a query object syntax (based on JSON) rather than SQL strings for finding documents.
Further considerations mention that while the connection mechanism is similar, the way data is structured (documents vs. tables) and queried differs significantly from SQL databases.
Connecting to NoSQL databases like MongoDB from Node.js also involves using a specific driver.
General Steps (Conceptual - using the official `mongodb` driver):
- Install the Driver: In your terminal: `npm install mongodb`
- Require the Library:
const { MongoClient } = require('mongodb'); // Or: import { MongoClient } from 'mongodb';
- Configure Connection (URI): Get your MongoDB connection string (Uniform Resource Identifier). Store securely using environment variables. It typically looks like `mongodb://username:password@host:port` or `mongodb+srv://...` for Atlas.
const uri = process.env.MONGODB_URI; const client = new MongoClient(uri); // Create a new MongoClient
- Connect & Perform Operations: Connect the client and use database/collection methods. Use `async/await`.
// Example using async/await async function findUserByEmail(email) { try { await client.connect(); // Connect the client to the server console.log("Connected successfully to MongoDB"); const database = client.db("myAppDatabase"); // Select database const usersCollection = database.collection("users"); // Select collection // Find one document that matches the query { email: email } const user = await usersCollection.findOne({ email: email }); return user; } catch (err) { console.error("MongoDB operation failed", err); throw err; } finally { // Ensures that the client will close when you finish/error await client.close(); console.log("MongoDB connection closed"); } } // Example usage: // findUserByEmail("user@example.com") // .then(user => console.log(user)) // .catch(e => console.error(e));
- CRUD Operations: The driver provides methods for:
- Create: `insertOne()`, `insertMany()`
- Read: `find()`, `findOne()`
- Update: `updateOne()`, `updateMany()`
- Delete: `deleteOne()`, `deleteMany()`
MongoDB uses object-based query filters instead of SQL strings. Security considerations like preventing NoSQL injection (though different from SQL injection) and securing connections are still important.
7. ORMs & ODMs: Simplifying Database Interactions
This section introduces Object-Relational Mappers (ORMs) and Object-Document Mappers (ODMs) – libraries that provide a higher level of abstraction for interacting with databases from JavaScript.
Objectively, ORMs/ODMs allow developers to work with database records using familiar JavaScript objects and methods, rather than writing raw SQL queries or database-specific commands.
Delving deeper: * ORM (Object-Relational Mapper): Used with SQL databases. Maps database tables/rows to JavaScript classes/objects. Provides methods for querying and manipulation (e.g., `User.findAll()`, `user.save()`). Popular example: Sequelize (for PostgreSQL, MySQL, etc.). * ODM (Object-Document Mapper): Used with NoSQL Document databases (like MongoDB). Maps database collections/documents to JavaScript classes/objects. Provides methods for schema definition, validation, querying, and manipulation. Popular example: Mongoose (for MongoDB). * Benefits: Increased developer productivity, more readable code (potentially), database abstraction (easier to switch DBs sometimes), built-in features like validation and relationship management.
Further considerations mention the potential drawbacks: performance overhead compared to raw queries, leaky abstractions (sometimes needing to understand underlying DB concepts anyway), and complexity in mastering the ORM/ODM itself.
Writing raw database queries (SQL or NoSQL commands) directly in your JavaScript code can become repetitive and complex, especially for intricate operations or when dealing with relationships between data.
ORMs (Object-Relational Mappers) and ODMs (Object-Document Mappers) are libraries that provide an abstraction layer, allowing you to interact with your database using JavaScript objects and methods instead.
- ORM (for SQL Databases):
- Concept: Maps relational database tables and rows to JavaScript classes and objects.
- How it works: You define "models" (JavaScript classes) that represent your database tables. The ORM provides methods like `Model.findAll()`, `Model.findOne()`, `instance.save()`, `instance.update()`, `instance.destroy()` to perform CRUD operations without writing SQL directly.
- Popular Example (Node.js): Sequelize (Supports PostgreSQL, MySQL, MariaDB, SQLite, Microsoft SQL Server).
- Benefits: Reduces boilerplate SQL, improves code readability (using JS methods), provides features like migrations, validations, and relationship handling (e.g., defining associations between models).
- ODM (for Document Databases, e.g., MongoDB):
- Concept: Maps database collections and documents to JavaScript classes and objects.
- How it works: You define "schemas" and "models" describing the structure of your documents. The ODM provides methods for querying, validation, middleware hooks, and population (linking related documents).
- Popular Example (Node.js for MongoDB): Mongoose.
- Benefits: Provides structure and validation for otherwise schema-less data, simplifies common operations, makes working with MongoDB data feel more object-oriented in JavaScript.
Trade-offs: While ORMs/ODMs boost productivity, they can introduce some performance overhead compared to highly optimized raw queries. They also add another layer of abstraction to learn, and sometimes you might still need to drop down to raw queries for very complex or specific database operations.
// --- Conceptual Example using an ORM (like Sequelize) ---
// Assume 'User' is a defined Sequelize model mapped to a 'users' table
async function getAllUsers() {
try {
const users = await User.findAll({
where: { status: 'active' },
attributes: ['id', 'name', 'email'] // Select specific columns
});
return users;
} catch (error) {
console.error("Error fetching users:", error);
}
}
// --- Conceptual Example using an ODM (like Mongoose) ---
// Assume 'Product' is a defined Mongoose model mapped to a 'products' collection
async function findProductById(productId) {
try {
// Mongoose methods often mirror MongoDB shell commands
const product = await Product.findById(productId).select('name price -_id'); // Find by ID, select fields
return product;
} catch (error) {
console.error("Error finding product:", error);
}
}
8. APIs as Intermediaries: The Common Pattern
This section describes the most common way client-side JavaScript interacts with databases – indirectly, by communicating with a server-side Application Programming Interface (API).
Objectively, for web applications, the browser (client-side JS) typically fetches data from or sends data to a back-end server via API calls (usually over HTTP/S). The server then handles the actual database interaction.
Delving deeper: * Why APIs? Security (database credentials aren't exposed), Abstraction (client doesn't need to know database details), Centralized Logic (validation and business rules enforced on the server), Scalability. * Client-Side Role: Use browser APIs like `Workspace` or libraries like `axios` to make HTTP requests (GET, POST, PUT, DELETE) to specific API endpoints (URLs) on the server. Handle the response data (often JSON) to update the UI. * Server-Side Role: Build API endpoints (using Node.js/Express, etc.) that receive client requests, interact with the database (using drivers or ORMs/ODMs as described earlier), process data, and send back appropriate HTTP responses.
Further considerations mention common API styles like REST (Representational State Transfer) and GraphQL, which define how client and server communicate.
As mentioned earlier, client-side JavaScript running in a browser should not connect directly to your main application database. The standard and secure approach is for the client to communicate with the database indirectly through a server-side API.
- API (Application Programming Interface): An interface defined by the server that specifies how clients can request data or perform actions. Think of it like a restaurant menu – the client orders from the menu (API endpoints), and the kitchen (server+database) prepares the food (data/action).
- Common Pattern (e.g., REST API):
- Server: A Node.js application (often using a framework like Express.js) defines various API endpoints (URLs), like `/api/users` or `/api/products/:id`. Each endpoint corresponds to specific actions (e.g., GET `/api/users` retrieves all users, POST `/api/users` creates a new user).
- Server Logic: When a request hits an endpoint, the server code validates it, interacts with the database (using drivers or ORM/ODM) to fetch or modify data, and then sends back an HTTP response, usually containing data in JSON (JavaScript Object Notation) format.
- Client: The client-side JavaScript uses the browser's `Workspace` API or a library like `axios` to make HTTP requests to these server endpoints.
- Client Logic: The client JS receives the JSON response from the server and uses that data to update the webpage (e.g., display user profiles, show product lists).
- Benefits: This separation provides security, allows the server to enforce rules, lets the client focus on presentation, and enables multiple clients (web, mobile) to use the same back-end.
// --- Conceptual Client-Side JS using fetch ---
async function displayUsers() {
try {
const response = await fetch('/api/users'); // Request data from server API
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const users = await response.json(); // Parse JSON response
// Code to display users on the webpage...
console.log(users);
} catch (error) {
console.error('Could not fetch users:', error);
}
}
// displayUsers(); // Call the function to fetch and display
This indirect interaction via APIs is the standard for most modern web applications involving JavaScript and databases.
9. Security Considerations for Database Interaction
This section highlights critical security practices that must be followed when allowing applications, especially those written in JavaScript (Node.js), to interact with databases.
Objectively, insecure database interactions can lead to data breaches, data corruption, and application downtime. Security must be a primary concern.
Delving deeper into key security points: * Preventing Injection Attacks: * *SQL Injection:* Use parameterized queries or ORMs that handle escaping properly. Never construct SQL queries by concatenating user input directly. * *NoSQL Injection:* While different from SQL injection, vulnerabilities can exist depending on the database and query methods. Sanitize and validate user input used in NoSQL queries. ODMs often provide some protection. * Secure Connection Credentials: Never hardcode database usernames, passwords, or connection strings in source code. Use environment variables or secure configuration management tools. * Principle of Least Privilege: Configure database users with only the minimum permissions necessary for the application to function. * Server-Side Validation: Always validate and sanitize data on the server-side before inserting or updating it in the database, even if client-side validation exists. Never trust client input. * Secure Transport: Use encrypted connections (SSL/TLS) between the application server and the database server. * Regular Updates: Keep database systems, drivers, ORMs/ODMs, and Node.js itself updated with security patches.
Further considerations reiterate why direct browser-to-database connections are insecure and emphasize the server's role as the security gatekeeper.
Security is paramount when dealing with databases. Failing to implement proper security can have severe consequences.
Key considerations for JavaScript (especially server-side Node.js) database interactions:
- Prevent Injection Attacks:
- SQL Injection: This occurs when malicious SQL code is inserted into input fields, potentially allowing attackers to read sensitive data, modify data, or even drop tables. Prevention: ALWAYS use parameterized queries (prepared statements) provided by your database driver or rely on an ORM (like Sequelize) that handles input sanitization correctly. NEVER build SQL queries by concatenating strings containing user input.
- NoSQL Injection: While the nature is different, NoSQL databases can also be vulnerable if user input is improperly handled in query construction (e.g., allowing users to inject operators into MongoDB queries). Prevention: Use ODMs (like Mongoose) that often provide schema validation and sanitization. Validate and sanitize all user input rigorously before using it in database queries.
- Secure Credentials Management:
- Never hardcode database usernames, passwords, or connection strings directly in your source code.
- Use environment variables (`process.env`) or secure secret management systems to store and access credentials.
- Ensure configuration files containing credentials are not committed to version control (e.g., Git).
- Principle of Least Privilege: Create dedicated database users for your application with only the permissions they absolutely need (e.g., only SELECT, INSERT, UPDATE on specific tables/collections, not full admin rights).
- Server-Side Validation: Always validate data thoroughly on the server-side before inserting or updating it in the database. Do not rely solely on client-side validation, as it can be easily bypassed.
- Secure Connections: Ensure the connection between your Node.js application server and the database server is encrypted using SSL/TLS, especially if they are on different machines or networks.
- Keep Software Updated: Regularly update Node.js, database drivers, ORMs/ODMs, and the database system itself to patch known security vulnerabilities.
- Why No Direct Browser Access: Reinforcing the point – exposing database credentials or allowing direct connections from the browser would make almost all the above protections impossible. The server acts as the essential security layer.
10. Conclusion & Resources
This concluding section summarizes JavaScript's role in database management and points towards further learning resources.
Objectively, JavaScript, particularly through server-side environments like Node.js, is a powerful tool for interacting with both SQL and NoSQL databases. Client-side JavaScript typically relies on APIs as intermediaries for security and abstraction.
Delving deeper, successful database management with JavaScript involves choosing appropriate database types, utilizing drivers or ORMs/ODMs effectively, understanding asynchronous operations (Promises, async/await), and prioritizing security through measures like parameterized queries and proper credential management.
Further considerations highlight emerging trends like serverless databases and edge computing databases, and emphasize the importance of consulting official documentation for specific databases, drivers, and ORMs/ODMs.
Conclusion: JavaScript as a Full-Stack Database Partner
JavaScript has evolved far beyond simple browser scripting. With Node.js, it has become a capable language for server-side development, including direct and secure management of database interactions. Understanding the distinction between client-side limitations (relying on APIs) and server-side capabilities (using drivers, ORMs/ODMs) is crucial.
Whether working with relational SQL databases like PostgreSQL or flexible NoSQL solutions like MongoDB, the JavaScript ecosystem offers robust tools (drivers, Sequelize, Mongoose) to connect, query, and manage data. However, this power comes with the responsibility of prioritizing security, understanding asynchronous patterns, and choosing the right tools for the job. By mastering these concepts, JavaScript developers can effectively build full-stack applications that leverage the power of modern databases.
Database & JavaScript Resources
Node.js & General JS:
- Node.js Official Documentation: nodejs.org
- MDN Web Docs (JavaScript): developer.mozilla.org (Promises, Fetch API, Async/Await)
Database Drivers & ORM/ODM Documentation:
- PostgreSQL Node.js Driver (`pg`): node-postgres.com
- MySQL Node.js Driver (`mysql2`): github.com/sidorares/node-mysql2
- MongoDB Node.js Driver: mongodb.github.io/node-mongodb-native/
- Sequelize ORM: sequelize.org
- Mongoose ODM: mongoosejs.com
Database Documentation:
- PostgreSQL: postgresql.org/docs/
- MySQL: dev.mysql.com/doc/
- MongoDB: docs.mongodb.com/
- Redis: redis.io/documentation
Security Resources:
- OWASP (Open Web Application Security Project): owasp.org (See SQL Injection, NoSQL Injection prevention cheatsheets)
References (Placeholder)
Links to specific tutorials or documentation pages could be added.
- (Placeholder for specific tutorials on Node.js + DB connections)
- (Placeholder for relevant security articles)
JS & DB Interaction Overview
(Placeholder: Graphic summarizing Client-Side (Storage/API) vs Server-Side (Node.js/Driver/ORM/DB) interaction)
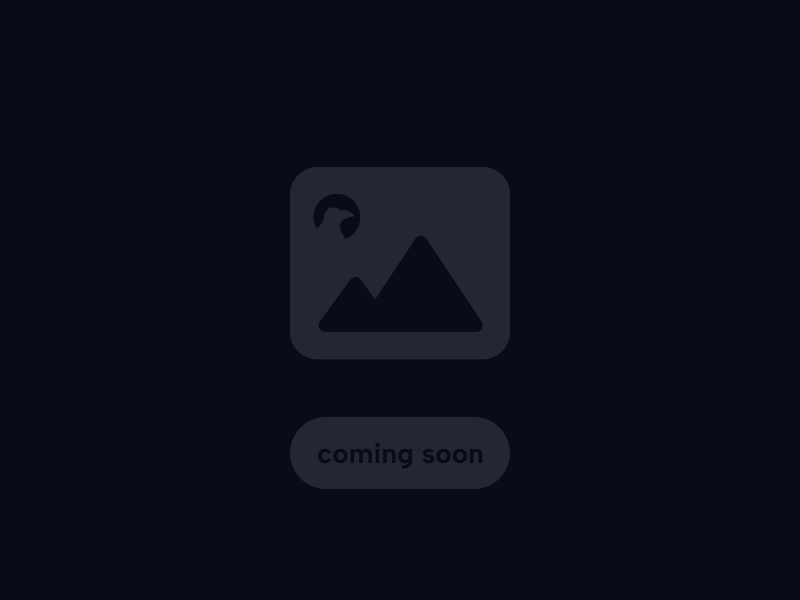