Adaptability & Risk Management in JavaScript Projects
Navigating the dynamic world of JavaScript development requires proactive strategies for adapting to change and managing inherent risks effectively.
This overview introduces the concepts of adaptability and risk management specifically tailored for JavaScript projects, addressing challenges like framework churn, dependency issues, technical debt, and security vulnerabilities.
Key takeaways focus on practical approaches including Agile methodologies, robust testing, dependency auditing, CI/CD pipelines, and security best practices to build resilient and maintainable JavaScript applications.
1. The Need for Adaptability & Risk Management in the JS Ecosystem
This section sets the stage by explaining why adaptability and proactive risk management are particularly crucial when working with JavaScript and its rapidly evolving ecosystem.
Objectively, the JavaScript world (including Node.js, front-end frameworks like React/Vue/Angular, build tools, and the vast npm registry) is known for its extremely fast pace of change, innovation, and sometimes, instability ("JavaScript fatigue," "framework churn").
Delving deeper, this rapid evolution introduces specific risks: frameworks becoming obsolete, dependencies introducing breaking changes or vulnerabilities, build tooling complexities, and the constant need for developers to update their skills.
Further considerations highlight that projects must be built with adaptability in mind to respond to these changes effectively. Simultaneously, identifying and mitigating potential risks proactively is essential for project success, preventing delays, cost overruns, and quality issues commonly seen in software development.
The JavaScript ecosystem is one of the most dynamic and rapidly evolving areas in software development. New frameworks, libraries, tools, and language features emerge constantly. While this innovation drives progress, it also introduces significant challenges and risks for development teams:
- Framework & Library Churn: Popular tools can rise and fall in favor relatively quickly, potentially leading to difficult migration paths or reliance on unmaintained code.
- Dependency Complexity: Modern JS projects often rely on hundreds or thousands of external packages via npm or yarn. Managing these dependencies, their updates, and potential vulnerabilities is a major task.
- Rapid Tooling Evolution: Build tools (Webpack, Vite, esbuild), testing frameworks, and development practices are continually updated, requiring teams to stay current.
- Pervasiveness of JS: JavaScript runs everywhere – browsers, servers (Node.js), mobile apps, desktops – increasing the attack surface and the variety of risks to consider.
In this environment, simply following a rigid plan is often insufficient. Adaptability – the ability to respond effectively to changing requirements, technologies, and market conditions – becomes a key success factor. Equally important is Risk Management – the proactive process of identifying potential problems (risks) that could negatively impact the project (schedule, budget, quality, security) and implementing strategies to mitigate them.
This guide explores practical strategies for enhancing adaptability and managing common risks within JavaScript development projects.
The Fast-Paced JS Ecosystem (Conceptual)
(Placeholder: Graphic showing a whirlwind or fast-moving river representing JS frameworks, libraries, tools)
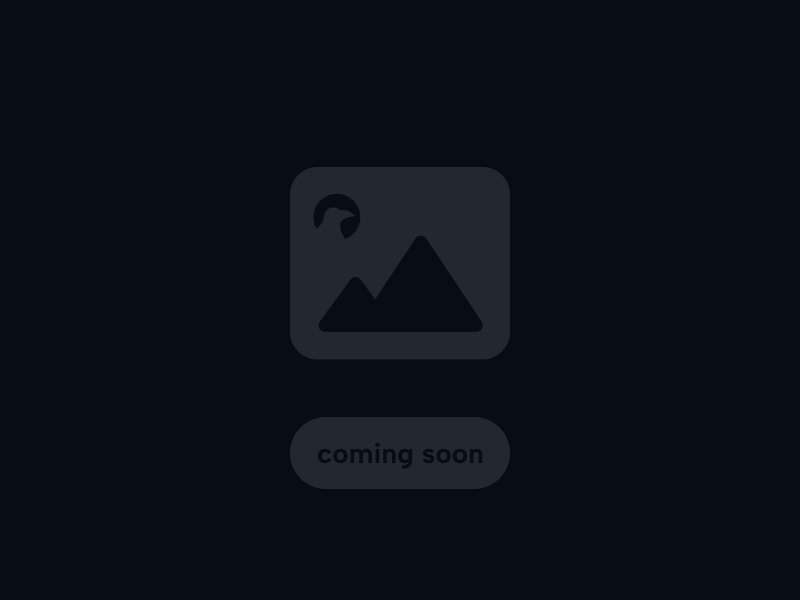
2. Identifying Key Risks in JavaScript Projects
This section outlines common risks that specifically affect projects utilizing JavaScript technologies, spanning technical, project management, and external factors.
Objectively, recognizing potential pitfalls early allows teams to plan mitigation strategies.
Delving deeper into key risk areas: * Technical Risks: * *Technical Debt:* Accumulation of suboptimal code (e.g., quick fixes, outdated patterns, lack of tests) hindering future development. Using deprecated JS features or legacy frameworks (like older Angular versions without upgrading). * *Dependency Risks:* Relying on external npm/yarn packages introduces risks of vulnerabilities (security holes in dependencies), breaking changes in updates, or packages becoming unmaintained ("Dependency Hell"). * *Performance Issues:* Inefficient client-side or server-side (Node.js) code leading to slow load times, poor responsiveness, or high server costs. * *Integration Issues:* Difficulties integrating with third-party APIs or internal legacy systems. * *Poor Code Quality:* Inconsistent coding styles, lack of documentation, complex logic making code hard to understand and maintain. * Project Management Risks: * *Scope Creep:* Uncontrolled changes or additions to project requirements. * *Unrealistic Deadlines/Estimates:* Poor planning leading to rushed work and compromised quality. * *Team Issues:* Lack of skills, poor communication, team member turnover. * Ecosystem Risks: * *Framework/Library Churn:* The chosen framework becomes outdated or less supported, necessitating costly migrations. * *Tooling Complexity:* Overly complex build processes or development environments. * Security Risks: * *Common Vulnerabilities:* Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), insecure direct object references, component vulnerabilities (via dependencies). * *Data Breaches:* Improper handling of sensitive data.
Further considerations involve classifying these risks based on likelihood and potential impact to prioritize mitigation efforts.
JavaScript projects face a unique set of potential risks alongside general software development challenges:
- Technical Debt Accumulation:
- Choosing quick-and-dirty solutions over robust design.
- Failing to refactor legacy JavaScript code (e.g., old jQuery-based logic mixed with modern frameworks).
- Not keeping up with language improvements (e.g., sticking to pre-ES6 patterns).
- Insufficient automated test coverage makes refactoring risky.
- Dependency Management ("Dependency Hell"):
- Vulnerabilities: npm packages can have known security holes (`npm audit` often reveals many).
- Breaking Changes: Updating dependencies can break your application if the package introduces incompatible API changes (semantic versioning helps but isn't foolproof).
- Unmaintained Packages: Relying on packages that are no longer actively developed or supported.
- Transitive Dependencies: Vulnerabilities or issues in dependencies *of your dependencies*.
- Rapid Ecosystem Changes & Framework Churn:
- The framework chosen (React, Vue, Angular, Svelte, etc.) might undergo major breaking changes or fall out of favor, requiring significant refactoring or migration efforts.
- Build tools and best practices evolve quickly.
- Performance Bottlenecks:
- Large JavaScript bundle sizes impacting front-end load times.
- Inefficient algorithms or excessive DOM manipulation slowing down the UI.
- Blocking operations or memory leaks in Node.js applications.
- Security Vulnerabilities:
- Client-Side: Cross-Site Scripting (XSS) remains a major threat if user input isn't properly sanitized before rendering.
- Server-Side (Node.js): Input validation failures leading to injection attacks (SQL, NoSQL, Command), insecure API endpoints, improper error handling revealing sensitive info.
- Dependency Vulnerabilities: Exploitable code in third-party npm packages.
- Cross-Browser/Environment Compatibility: Ensuring code works consistently across different browsers and versions, or different Node.js versions.
- Project Management Risks: Scope creep, inaccurate estimations, communication breakdowns, resource constraints – these apply broadly but can be exacerbated by the pace of JS development.
Identifying which of these risks are most relevant to *your* project is the first step in managing them.
Common JS Project Risk Areas (Conceptual)
(Placeholder: Mind map or list showing categories: Technical Debt, Dependencies, Ecosystem, Security, Performance, Project Mgmt)
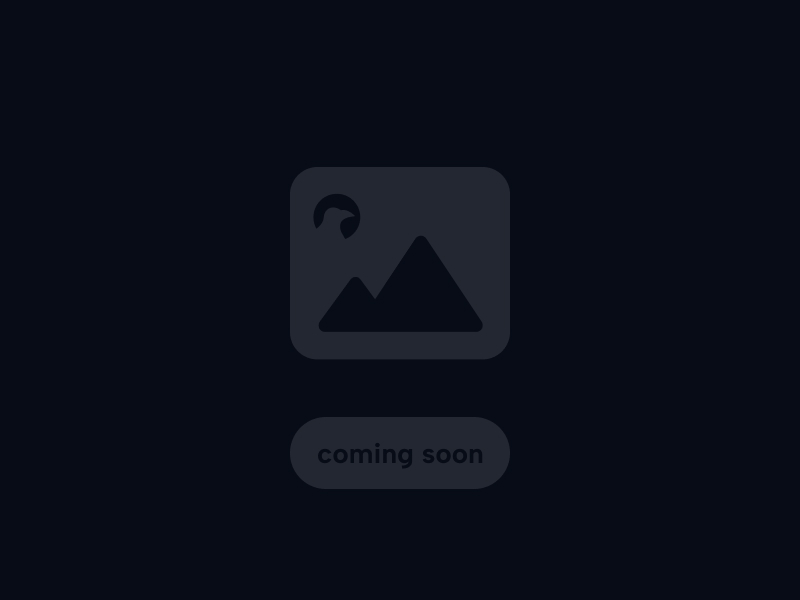
3. Strategies for Building Adaptability into JS Projects
This section focuses on proactive strategies and development practices that enhance a JavaScript project's ability to adapt to change.
Objectively, building adaptability requires conscious effort in architecture, process, and team culture.
Delving deeper into strategies: * Agile Methodologies (Scrum, Kanban): Embracing iterative development, frequent feedback loops, and flexible planning allows teams to respond to changing requirements and priorities more effectively than rigid waterfall approaches. Prioritize working software and collaboration. * Modular Architecture: Designing the application as a set of loosely coupled, independent modules or services. * *Back-end:* Microservices architecture allows individual services (often Node.js) to be updated or replaced independently. * *Front-end:* Component-based design (standard in React, Vue, Angular) promotes reusability. Micro-frontends take this further, allowing independent deployment of UI sections. * Feature Flags (Feature Toggles): Allows deploying new features to production disabled, then enabling them gradually or for specific user groups. This decouples deployment from release and reduces risk. * Continuous Learning Culture: Encouraging developers to stay updated with the JS ecosystem, explore new tools pragmatically, and share knowledge within the team. * Regular Refactoring: Making refactoring (improving code structure without changing external behavior) an ongoing part of the development process, not just a separate phase, keeps the codebase healthy and easier to change (the "Boy Scout Rule").
Further considerations involve using abstraction layers to isolate dependencies (especially external APIs or volatile libraries) and investing in good documentation.
To thrive in the dynamic JavaScript world, projects need to be designed and managed for adaptability:
- Embrace Agile Methodologies: Frameworks like Scrum or Kanban promote:
- Iterative Development: Building and delivering working software in short cycles (sprints).
- Frequent Feedback: Regularly demonstrating progress to stakeholders and incorporating their feedback.
- Adaptive Planning: Recognizing that requirements will change and having processes to accommodate adjustments.
- Collaboration: Prioritizing communication within the team and with stakeholders.
- Adopt Modular Architecture: Avoid building monolithic applications where changes in one part ripple unexpectedly through the entire system.
- Component-Based UI (React, Vue, Angular): Build interfaces from reusable, self-contained components.
- Microservices (Backend): Break down large Node.js backends into smaller, independent services that communicate via APIs. Changes to one service have less impact on others.
- Micro-frontends: Apply similar principles to the front-end, allowing different teams to work on parts of the UI independently using potentially different technologies.
- Clear API Contracts: Define stable interfaces between modules/services.
- Utilize Feature Flags (Toggles): Implement mechanisms to turn features on or off in production without redeploying code. This allows:
- Testing features with a subset of users (Canary Releases, A/B testing).
- Quickly disabling problematic features without rollbacks.
- Decoupling feature releases from code deployments.
- Foster a Culture of Continuous Learning: Encourage developers to:
- Keep up-to-date with relevant JS ecosystem trends (without chasing every new hype).
- Experiment with new tools and techniques.
- Share knowledge through code reviews, presentations, or pair programming.
- Practice Continuous Refactoring: Regularly improve the internal structure of the code without changing its external behavior. Follow the "Boy Scout Rule": leave the code cleaner than you found it. This prevents technical debt from accumulating and keeps the codebase malleable.
Adaptability Pillars (Conceptual)
(Placeholder: Icons/List: Agile Cycle, Modular Blocks, Feature Flag Switch, Learning Brain, Refactor Arrows)
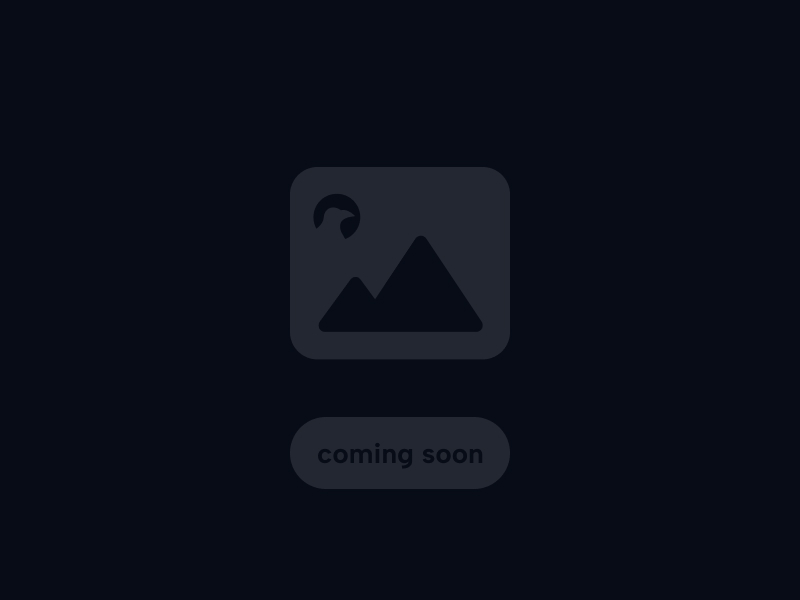
4. Managing Technical Debt in JavaScript
This section focuses specifically on identifying and managing technical debt – the implied cost of rework caused by choosing an easy (limited) solution now instead of using a better approach that would take longer.
Objectively, technical debt slows down future development, increases bug rates, and makes the codebase harder to understand and modify. It's a common risk in fast-moving JS projects.
Delving deeper into management strategies: * Identification: Recognize signs like complex/duplicated code, poor test coverage, outdated dependencies/frameworks, lack of documentation, frequent bugs in specific areas. Code analysis tools (linters, complexity checkers) can help. * Quantification & Prioritization: Assess the *impact* of specific debt items (e.g., does it slow down critical feature development? Does it pose a security risk?). Prioritize addressing high-impact debt. Communicate this impact in business terms (e.g., faster feature delivery, reduced bugs). * Integrate into Workflow: Don't treat debt reduction as a separate, massive project. Allocate a portion of each sprint or development cycle to addressing prioritized debt items (e.g., 10-20% capacity). Apply the "Boy Scout Rule." * Strategic Refactoring: Focus refactoring efforts where they provide the most value. Use techniques like Test-Driven Development (TDD) to refactor safely. Break down large refactoring tasks into smaller, manageable steps. * Automation & Standards: Use linters (ESLint, Prettier) to enforce coding standards automatically. Automate tests to ensure refactoring doesn't break existing functionality.
Further considerations involve fostering team ownership and making technical health a visible metric.
Technical debt refers to the long-term consequences of choosing expedient, easy solutions now instead of better, more sustainable approaches that might take longer to implement. In JavaScript projects, it can manifest as messy code, outdated libraries, poor architecture, or lack of tests.
Managing technical debt is crucial for maintaining development velocity and adaptability:
- Identify and Visualize Debt:
- Actively look for code smells (complexity, duplication, unclear logic).
- Track areas with high bug rates or frequent modification difficulties.
- Use static analysis tools and code complexity metrics.
- Maintain a technical debt backlog (e.g., specific tickets in Jira or your task tracker).
- Quantify and Prioritize:
- Not all debt is equal. Assess the *business impact* of each debt item. Does it slow down feature development? Increase operational risk? Hinder onboarding new developers?
- Estimate the effort required to fix it.
- Prioritize tackling debt with high negative impact and potentially reasonable fixing effort. Communicate this priority in terms non-technical stakeholders can understand (e.g., "Fixing this will let us deliver feature X 20% faster").
- Integrate Debt Management into Workflow:
- Allocate a consistent percentage of development time (e.g., 10-20% per sprint) specifically for addressing technical debt from the backlog.
- Encourage the "Boy Scout Rule": Leave the code better than you found it whenever working in an area.
- Link refactoring efforts to feature development where appropriate.
- Refactor Strategically and Safely:
- Ensure adequate test coverage *before* starting significant refactoring to verify behavior remains unchanged.
- Break down large refactoring tasks into smaller, incremental steps.
- Focus on improving clarity, reducing complexity, and adhering to established coding standards.
- Automate Quality Checks:
- Use linters (like ESLint) and code formatters (like Prettier) integrated into the development environment and CI pipeline to enforce standards and catch potential issues early.
Technical Debt Quadrant (Conceptual Prioritization)
(Placeholder: 2x2 Matrix showing Impact vs. Effort for prioritizing tech debt items)
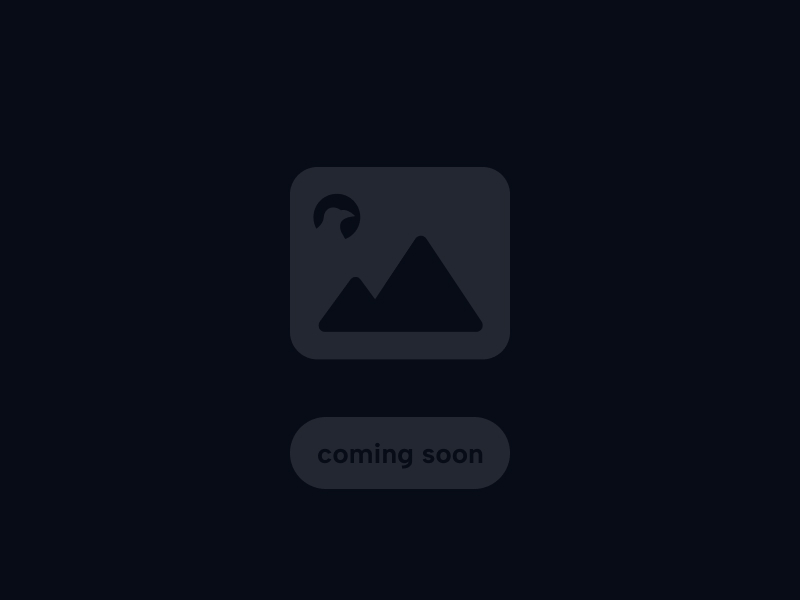
5. Dependency Management Risks & Mitigation (npm/yarn)
This section addresses the specific risks associated with managing external dependencies in JavaScript projects using package managers like npm and yarn, and outlines mitigation strategies.
Objectively, the ease of adding dependencies via `npm install` or `yarn add` is a double-edged sword, introducing potential security, stability, and maintenance risks.
Delving deeper into mitigation strategies: * Use Lockfiles: Always commit `package-lock.json` (npm) or `yarn.lock` (yarn) to version control. These files lock down the exact versions of all dependencies (including transitive ones), ensuring consistent installations across different environments (developer machines, CI, production). * Audit Dependencies: Regularly run `npm audit` or `yarn audit` to check for known security vulnerabilities in your dependencies and their dependencies. Address critical/high severity vulnerabilities promptly. * Automated Scanning: Integrate dependency scanning tools (like Snyk, Dependabot, Mend Renovate) into your CI/CD pipeline or repository settings to automatically detect vulnerabilities and even suggest/create pull requests for updates. * Update Dependencies Cautiously: Keep dependencies reasonably up-to-date to benefit from bug fixes and security patches, but do so carefully. Update incrementally, test thoroughly after updates (especially major version bumps which may contain breaking changes), and review changelogs. Tools like `npm-check-updates` or `yarn upgrade-interactive` can help manage this. * Choose Dependencies Wisely: Evaluate potential dependencies before adding them. Consider their popularity, maintenance activity (last commit/publish date), number of open issues, license compatibility, and whether a native browser/Node.js API could suffice. Prefer smaller, focused packages over large monolithic ones where possible.
Further considerations include understanding semantic versioning (SemVer) conventions used by most packages, but not relying on it absolutely to prevent breaking changes.
Modern JavaScript projects heavily rely on external packages managed by npm (Node Package Manager) or Yarn. While this accelerates development, it introduces significant risks:
- Security Vulnerabilities: Dependencies (or dependencies of dependencies) can contain known security flaws that attackers could exploit.
- Breaking Changes: Updating a dependency might introduce changes that break your application's functionality, especially during major version upgrades.
- Unmaintained Packages: A dependency might become abandoned by its maintainer, no longer receiving bug fixes or security patches.
- License Issues: Dependencies might have licenses incompatible with your project's requirements.
- Bloat: Too many dependencies can increase bundle size, installation times, and the overall complexity/attack surface.
Mitigation Strategies:
- Use and Commit Lockfiles: This is crucial. `package-lock.json` (for npm) or `yarn.lock` (for Yarn) records the *exact* versions of all installed packages, including indirect dependencies. Committing this file ensures everyone on the team and the CI/CD pipeline installs the exact same dependency tree, preventing "works on my machine" issues. Use `npm ci` or `yarn install --frozen-lockfile` in automated environments.
- Regularly Audit Dependencies:
- Run `npm audit` or `yarn audit` frequently to identify known vulnerabilities.
- Review the audit report and address high/critical severity issues by updating packages or finding alternatives.
- Automate Vulnerability Scanning: Integrate tools like GitHub Dependabot, Snyk, or Mend Renovate into your workflow. These tools automatically scan dependencies, alert you to vulnerabilities, and can often create pull requests to update vulnerable packages.
- Update Dependencies Strategically:
- Don't blindly update everything. Update dependencies incrementally and test thoroughly afterward.
- Pay close attention to major version updates (e.g., 1.x.x to 2.0.0), as these often contain breaking changes according to Semantic Versioning (SemVer). Read the release notes/changelogs.
- Tools like `npm-check-updates` (`ncu`) or `yarn upgrade-interactive --latest` can help identify available updates beyond the constraints in `package.json`.
- Vet Dependencies Before Adding:
- Is the package actively maintained? Check its GitHub repository activity, last publish date on npm.
- Is it widely used and well-regarded? Check download counts, GitHub stars, community feedback.
- Does it have known issues or vulnerabilities? Check issue trackers and audit results.
- Is the license compatible with your project?
- Could you achieve the same result with native JavaScript APIs or a smaller, existing dependency?
Dependency Management Workflow (Conceptual)
(Placeholder: Flowchart: Add Dep -> Commit Lockfile -> Audit Regularly -> Scan (CI) -> Update Cautiously -> Test)
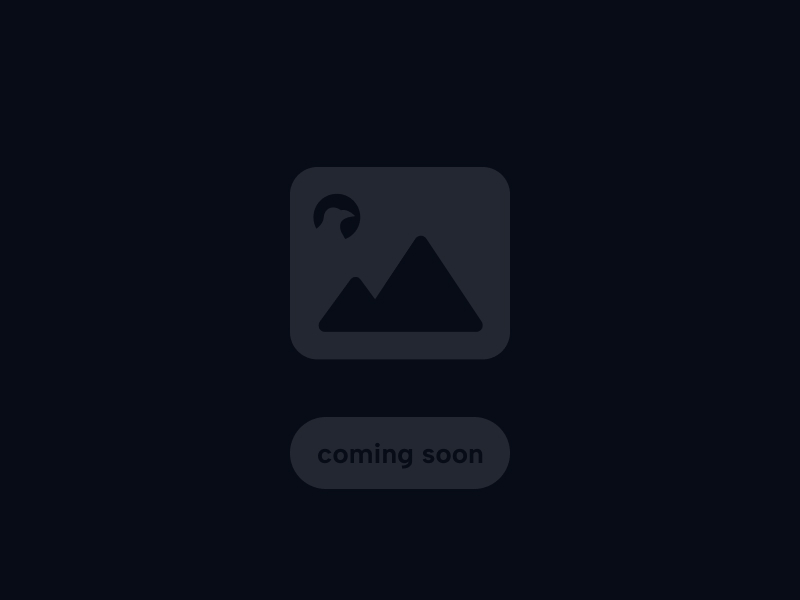
6. Mitigating Risks Through Comprehensive Testing
This section highlights the role of automated testing as a fundamental risk mitigation strategy in JavaScript development.
Objectively, a robust testing suite helps catch bugs early, verifies functionality, prevents regressions (old bugs reappearing), and provides confidence when refactoring code or updating dependencies.
Delving deeper into common testing types in JS: * Unit Testing: Testing small, isolated pieces of code (like individual functions or components) in isolation from their dependencies (using mocks/stubs). *Tools:* Jest, Mocha, Chai, Sinon. * Integration Testing: Testing the interaction between multiple units or modules to ensure they work together correctly (e.g., testing if a component correctly calls an API service mock and processes the response). *Tools:* Often uses same tools as unit testing, but with less mocking. React Testing Library encourages testing component interactions. * End-to-End (E2E) Testing: Simulating real user workflows through the entire application (front-end UI interacting with a real or test back-end) in a browser environment. Verifies critical user journeys. *Tools:* Cypress, Playwright, Selenium. * Other Types: Component testing (UI components in isolation), Performance testing, Security testing.
Further considerations include aiming for good test coverage (though 100% is often impractical), writing clear and maintainable tests, and integrating testing into the CI/CD pipeline.
Automated testing is one of the most effective ways to manage risks related to code quality, regressions, and unexpected behavior in JavaScript applications.
Different levels of testing address different risks:
- Unit Testing:
- Focus: Testing individual functions, modules, or components in isolation.
- Goal: Verify that each small piece of code works correctly according to its specification. Catch logic errors early.
- Technique: Dependencies are often "mocked" or "stubbed" to isolate the unit under test.
- JS Tools: Jest, Mocha (test runners), Chai (assertion library), Sinon (mocks/stubs).
- Benefit: Fast to run, easy to pinpoint failures. Forms the base of the testing pyramid.
- Integration Testing:
- Focus: Testing the interaction and communication between multiple units or modules.
- Goal: Verify that components work together as expected (e.g., does module A correctly pass data to module B? Does a UI component fetch data correctly from a service layer?).
- Technique: Involves testing integrated parts, potentially with some external dependencies (like a test database) or mocked interfaces.
- JS Tools: Can use unit testing frameworks; libraries like React Testing Library help test component integration from a user perspective. Supertest is common for testing Node.js API integrations.
- Benefit: Catches issues arising from component interactions.
- End-to-End (E2E) Testing:
- Focus: Testing complete user workflows through the entire application stack (UI, backend APIs, database).
- Goal: Simulate real user scenarios (e.g., logging in, adding item to cart, checking out) to ensure the whole system functions correctly from the user's perspective.
- Technique: Uses browser automation tools to interact with the UI like a user would.
- JS Tools: Cypress, Playwright, Selenium WebDriver (with JS bindings).
- Benefit: Provides highest confidence in overall application functionality but are slower and more brittle (prone to breaking due to UI changes) than unit/integration tests. Should focus on critical paths.
A balanced testing strategy (the "Testing Pyramid/Trophy") typically involves many fast unit tests, fewer integration tests, and even fewer E2E tests focusing on critical user flows. Integrating these tests into a CI/CD pipeline ensures they run automatically on every code change.
Testing Pyramid/Trophy (Conceptual)
(Placeholder: Diagram showing layers: Many Unit Tests at base, fewer Integration Tests, fewest E2E Tests at top)
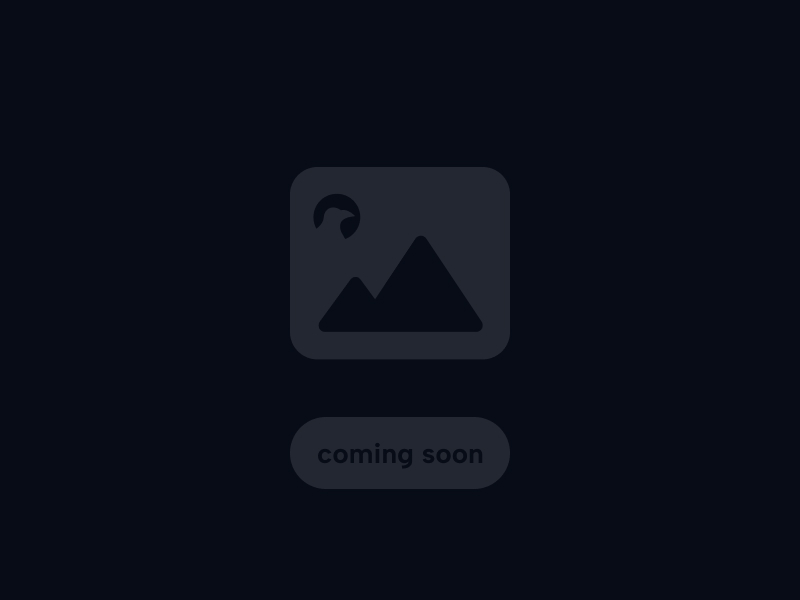
7. CI/CD Pipelines for Risk Reduction in JavaScript
This section explains how Continuous Integration (CI) and Continuous Deployment/Delivery (CD) pipelines help mitigate risks and improve adaptability in JavaScript projects.
Objectively, CI/CD pipelines automate the process of building, testing, and deploying code changes, providing faster feedback and more reliable releases.
Delving deeper into CI/CD benefits for JS: * Continuous Integration (CI): Developers merge code changes frequently (e.g., daily) into a central repository, after which automated builds and tests (unit, integration, linting, security scans) are run. * *Risk Mitigation:* Catches integration errors, bugs, style issues, and security vulnerabilities early and automatically. Reduces "merge hell." * Continuous Delivery (CD): Extends CI by automatically deploying every validated build to a testing/staging environment. * *Risk Mitigation:* Ensures code is always in a deployable state. Allows for thorough testing in a production-like environment before manual release. * Continuous Deployment (CD): Goes further by automatically deploying every validated build directly to production. * *Risk Mitigation:* Enables rapid delivery of small changes, reducing the risk associated with large, infrequent releases. Requires high confidence in automated testing and robust rollback strategies. * Tools: GitHub Actions, GitLab CI/CD, Jenkins, CircleCI, Buddy.Works, Azure DevOps Pipelines, AWS CodePipeline.
Further considerations involve configuring pipelines specifically for JS projects (e.g., running `npm install`, build steps like Webpack/Vite, executing Jest/Cypress tests, deploying to cloud services).
Continuous Integration (CI) and Continuous Deployment/Delivery (CD) are crucial DevOps practices that significantly reduce risks and enhance adaptability in JavaScript development.
- Continuous Integration (CI):
- Process: Developers frequently integrate their code changes into a shared repository (e.g., Git). Each integration triggers an automated process that builds the application and runs automated tests (unit tests, integration tests, linters, security scans).
- Benefits for JS:
- Early Bug Detection: Catches integration issues and bugs quickly after code changes.
- Improved Code Quality: Enforces code style (linting) and testing standards automatically.
- Reduced Merge Conflicts: Frequent integration minimizes large, complex merges.
- Faster Feedback: Developers get immediate feedback on whether their changes broke the build or tests.
- Continuous Delivery (CD):
- Process: Extends CI. Every code change that passes the automated CI tests is automatically prepared and deployed to a pre-production environment (e.g., staging, QA). Deployment to the *production* environment is typically a manual trigger (button click).
- Benefits: Ensures that every validated build is potentially shippable. Reduces manual deployment effort and errors for pre-prod environments. Allows for thorough testing before final release.
- Continuous Deployment (CD):
- Process: The most advanced stage. Every code change that passes all automated CI and potentially staging tests is *automatically* deployed to production without manual intervention.
- Benefits: Fastest delivery cycle. Releases small changes frequently, reducing the risk associated with large deployments.
- Requires: High confidence in automated testing, robust monitoring, and effective rollback mechanisms.
Implementing CI/CD pipelines for JavaScript projects typically involves:
- Using a CI/CD platform (e.g., GitHub Actions, GitLab CI, Jenkins, CircleCI, Azure DevOps).
- Configuring the pipeline steps: checkout code, install dependencies (`npm ci` or `yarn install --frozen-lockfile`), lint (`npm run lint`), build (`npm run build`), run tests (`npm test`), potentially security scans, and deploy (to static hosting, PaaS, containers, serverless functions).
CI/CD automates crucial quality gates, reduces manual errors, and enables faster, more reliable delivery of JavaScript applications.
Simple CI/CD Pipeline Flow (Conceptual)
(Placeholder: Flowchart: Code Commit -> CI Server (Build -> Lint -> Test) -> Deploy to Staging -> (Manual Trigger) -> Deploy to Production)
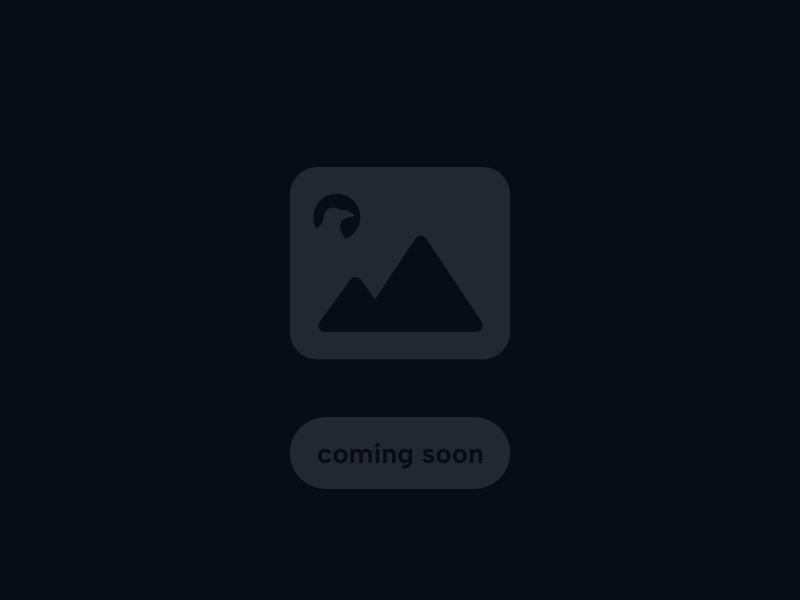
8. Handling Framework & Ecosystem Changes
This section addresses the challenge of "framework churn" and rapid evolution within the JavaScript ecosystem and suggests strategies for managing it.
Objectively, the constant influx of new libraries, frameworks, and updates requires a pragmatic approach to technology adoption and maintenance.
Delving deeper into strategies: * Stay Informed, Not Overwhelmed: Keep abreast of major trends and updates relevant to your stack (e.g., new versions of your framework, Node.js LTS releases, significant new browser APIs) through reputable blogs, newsletters, and conference talks. Avoid chasing every new minor library ("shiny object syndrome"). * Pragmatic Evaluation: When considering adopting a new framework, library, or major version upgrade, evaluate its stability, community support, documentation quality, learning curve, and actual benefits *for your specific project* versus the migration cost and risks. Don't adopt just because it's new. * Build Stable Cores: Where possible, encapsulate core business logic in framework-agnostic modules or layers. This makes it potentially easier to migrate the UI or specific infrastructure parts later without rewriting everything. * Plan for Upgrades: Don't let your core frameworks or Node.js versions become excessively outdated. Plan regular, incremental upgrades (e.g., staying within Long-Term Support (LTS) versions) rather than facing massive, risky jumps later. Allocate time for this maintenance. * Abstraction Layers: Introduce abstraction layers around particularly volatile third-party dependencies or browser APIs if their interfaces are likely to change frequently. * Leverage Standards: Prefer solutions based on web standards (like native Web Components, standard JS features) where they meet requirements, as these tend to have longer-term stability than proprietary framework features.
Further considerations involve fostering a team culture that values stability and maintainability alongside innovation.
The JavaScript ecosystem's rapid evolution ("churn") presents both opportunities and risks. Staying current is important, but constantly switching tools or chasing the latest hype can be counterproductive and destabilizing.
Strategies for managing ecosystem change:
- Be Informed, Selectively: Keep an eye on major developments (e.g., new ECMAScript features, major framework releases, Node.js LTS updates) through trusted sources (MDN, official blogs, key community figures). You don't need to know every new micro-library.
- Evaluate Pragmatically: Before adopting a new framework, major library version, or tool:
- Does it solve a real problem you have significantly better than current solutions?
- Is it stable and mature? Check version number (avoid pre-1.0 for critical production use unless necessary), issue tracker activity, community adoption.
- How good is the documentation and community support?
- What is the learning curve and migration cost for your team?
- What are the potential risks (e.g., becoming unmaintained)?
- Prioritize Stability for Core Dependencies: Be more conservative when choosing or updating critical dependencies like core frameworks, state management libraries, or data access layers.
- Isolate Volatility: If using a newer or less stable library, try to contain its usage within specific modules with clear interfaces, making it easier to replace later if needed (Abstraction Layers).
- Plan for Maintenance & Upgrades: Don't let your stack become ancient. Schedule regular time to update dependencies and Node.js/framework versions (especially LTS versions). Small, regular updates are less risky than huge jumps years later.
- Focus on Fundamentals: Strong understanding of core JavaScript, HTML, CSS, HTTP, and software design principles provides a stable foundation regardless of specific framework trends.
- Consider Framework-Agnostic Logic: For core business logic, especially in Node.js backends, try to write it in plain JavaScript/TypeScript, keeping it separate from framework-specific code where possible.
Finding a balance between leveraging new improvements and maintaining stability is key.
Balancing Innovation vs. Stability (Conceptual)
(Placeholder: Image of a scale balancing 'New Tech Adoption' and 'Project Stability/Maintenance')
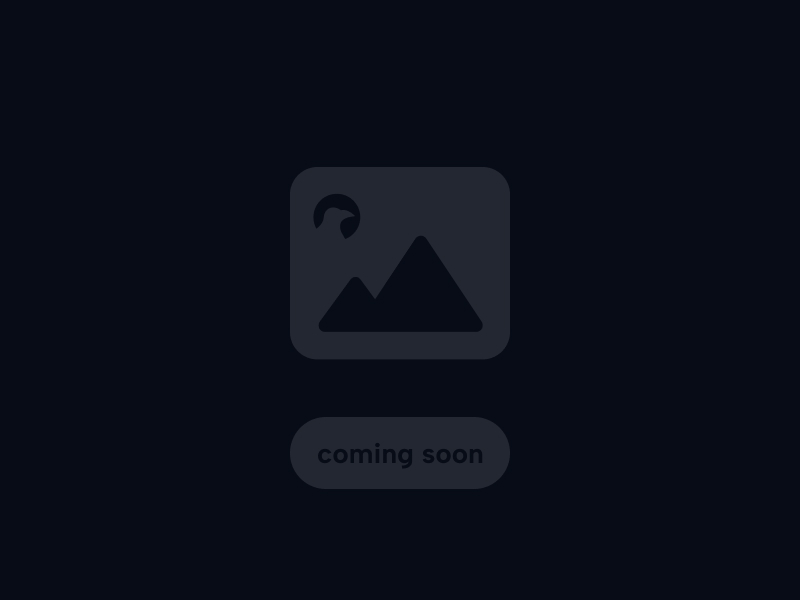
9. Security Risks & Best Practices in JavaScript Development
This section focuses on common security vulnerabilities relevant to JavaScript applications (both client-side and server-side with Node.js) and outlines essential best practices based on resources like OWASP.
Objectively, security must be a primary concern throughout the development lifecycle, as vulnerabilities can lead to data breaches, service disruption, and reputational damage.
Delving deeper into key areas: * Common Vulnerabilities: * *Cross-Site Scripting (XSS):* Injecting malicious scripts into websites viewed by other users. Mitigation: Proper output encoding/escaping (frameworks often help), Content Security Policy (CSP). * *Cross-Site Request Forgery (CSRF):* Tricking a user's browser into making unintended requests to a site where they are authenticated. Mitigation: Anti-CSRF tokens. * *Injection Attacks (SQL, NoSQL, Command - primarily Node.js):* Exploiting improperly validated user input to manipulate backend queries or execute commands. Mitigation: Parameterized queries/ORMs, input validation/sanitization. * *Insecure Direct Object References (IDOR):* Accessing unauthorized data by manipulating identifiers (e.g., changing a URL parameter). Mitigation: Proper authorization checks. * *Using Components with Known Vulnerabilities:* Relying on outdated or vulnerable npm packages. Mitigation: Dependency scanning (`npm audit`), regular updates. * Best Practices: * Input Validation: Validate and sanitize *all* input from users or external sources on the server-side. Use allow-lists where possible. Libraries like `validator.js` or framework built-ins help. * Output Encoding/Escaping: Properly encode data before rendering it in HTML to prevent XSS. Modern frameworks often handle this automatically, but be careful when directly manipulating the DOM or using `dangerouslySetInnerHTML`. * Secure Authentication & Session Management: Use robust libraries/frameworks, enforce strong passwords, use HTTPS, secure cookies (HttpOnly, Secure flags). * Proper Authorization/Access Control: Ensure users can only access data and perform actions they are permitted to. * Dependency Management: Keep packages updated and audited (as per Section 5). * Security Headers: Implement HTTP headers like Content Security Policy (CSP), Strict-Transport-Security (HSTS), X-Frame-Options, X-Content-Type-Options. * Error Handling: Avoid leaking sensitive information in error messages, especially in production (`NODE_ENV=production`). * Rate Limiting: Protect APIs from abuse and brute-force attacks.
Further considerations include regular security testing (static analysis - SAST, dynamic analysis - DAST, penetration testing) and staying informed via resources like the OWASP Top Ten.
Security is non-negotiable. JavaScript applications, both client-side and server-side (Node.js), are susceptible to various attacks if not developed securely.
Key Security Risks & OWASP-Aligned Best Practices:
- Input Validation & Sanitization:
- Risk: Injection attacks (SQL, NoSQL, Command Injection), Cross-Site Scripting (XSS).
- Practice: Never trust user input. Validate all incoming data on the server-side against expected formats and constraints (use allow-lists preferably). Sanitize data before using it in database queries, file paths, or system commands. Use parameterized queries or ORMs/ODMs to prevent SQL/NoSQL injection. Libraries like `express-validator` (Node.js) or `validator.js` can help.
- Output Encoding & Escaping:
- Risk: Cross-Site Scripting (XSS).
- Practice: Encode data appropriately before rendering it in HTML contexts. Modern front-end frameworks (React, Vue, Angular) usually handle this by default for content rendering, but be cautious when directly manipulating the DOM or using features like `dangerouslySetInnerHTML`. Implement a strong Content Security Policy (CSP) HTTP header.
- Authentication & Session Management:
- Risk: Broken Authentication (hijacked accounts, weak passwords).
- Practice: Use standard, secure libraries/frameworks for authentication (e.g., Passport.js in Node). Implement multi-factor authentication (MFA). Enforce strong password policies. Securely manage sessions using HttpOnly, Secure cookies or robust token mechanisms (JWTs handled correctly). Protect against Cross-Site Request Forgery (CSRF) using anti-CSRF tokens.
- Authorization / Access Control:
- Risk: Broken Access Control (users accessing data/functions they shouldn't).
- Practice: Implement authorization checks on the server-side for every sensitive operation or data access request based on the authenticated user's role or permissions. Don't rely on hiding UI elements client-side for security.
- Dependency Security:
- Risk: Using Components with Known Vulnerabilities.
- Practice: Regularly run `npm audit` or use automated tools (Snyk, Dependabot) to scan for vulnerabilities in dependencies. Update vulnerable packages promptly.
- Secure Headers & Configuration:
- Risk: Various attacks like clickjacking, MIME sniffing.
- Practice: Implement security-related HTTP headers: `Strict-Transport-Security` (HSTS), `X-Frame-Options`, `X-Content-Type-Options`, `Content-Security-Policy` (CSP). Configure servers securely (e.g., disable unnecessary information disclosure in headers). Set `NODE_ENV=production` in Node.js production environments to suppress detailed error messages.
- Logging & Monitoring: Implement sufficient logging to detect suspicious activity, but avoid logging sensitive data (passwords, tokens).
Referencing the OWASP Top Ten project provides guidance on the most critical web application security risks.
Key JS Security Pillars (Conceptual)
(Placeholder: Icons: Input Validation (Filter), Output Encoding (Shield), AuthN/AuthZ (Lock/Key), Dependency Scan (Bug), Secure Headers (Helmet))
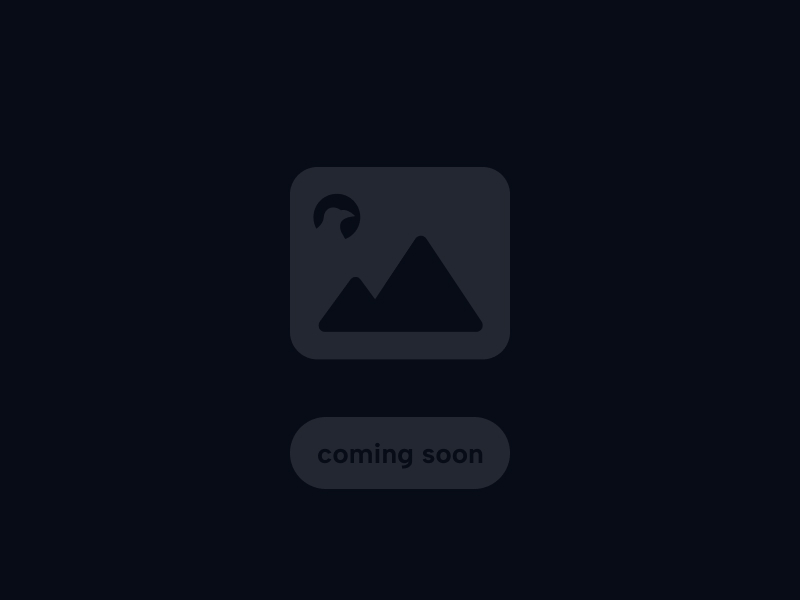
10. Conclusion: Cultivating Adaptability & Risk Awareness
This concluding section summarizes the importance of integrating adaptability and risk management into the culture and processes of JavaScript development teams.
Objectively, succeeding in the fast-paced JavaScript ecosystem requires more than just technical skill; it demands a proactive mindset towards change and potential threats.
Delving deeper, building adaptable systems (through modularity, agile practices, continuous learning) and managing risks systematically (identifying, prioritizing, mitigating via testing, CI/CD, dependency management, security practices) are intertwined and essential for delivering value reliably and sustainably.
Further considerations emphasize that these practices are not one-off tasks but require ongoing effort and a supportive team culture where discussing risks, addressing tech debt, and adapting to new information is encouraged.
Conclusion: Building Resilient JavaScript Applications
The JavaScript ecosystem offers incredible power and flexibility, but its rapid pace demands a development approach grounded in adaptability and proactive risk management. Ignoring these can lead to brittle applications, mounting technical debt, security breaches, and ultimately, project failure.
By embracing agile principles, designing modular systems, managing dependencies diligently, implementing comprehensive testing strategies, leveraging CI/CD pipelines, addressing technical debt systematically, staying vigilant about security, and pragmatically handling ecosystem changes, JavaScript teams can build applications that are not only functional but also resilient, maintainable, and capable of evolving with the landscape. Cultivating a team culture that values these practices is fundamental to long-term success.
Resources for Adaptability & Risk Management
Agile & Process:
- Agile Manifesto: agilemanifesto.org
- Scrum Guide: scrumguides.org
- Kanban Resources (e.g., Kanban University)
Technical Debt & Refactoring:
- Martin Fowler's Blog/Books (Refactoring): martinfowler.com (Technical Debt Tag)
- Books: "Refactoring: Improving the Design of Existing Code" by Martin Fowler, "Working Effectively with Legacy Code" by Michael Feathers.
Dependency Management & Security:
- npm Docs: docs.npmjs.com (See `npm audit`, `npm ci`)
- Yarn Docs: yarnpkg.com/cli (See `yarn audit`, `install --frozen-lockfile`)
- OWASP Top Ten Project: owasp.org/www-project-top-ten/
- OWASP Cheat Sheet Series (Node.js, Input Validation, etc.): cheatsheetseries.owasp.org
- Snyk / Dependabot / Mend Renovate websites.
Testing & CI/CD:
- Jest Docs: jestjs.io
- Cypress Docs: docs.cypress.io
- Playwright Docs: playwright.dev/docs
- Documentation for CI/CD platforms (GitHub Actions, GitLab CI, Jenkins, CircleCI, etc.)
References (Placeholder)
References to specific risk management frameworks (like ISO 31000), software engineering texts, or key blog posts could be added.
- (Placeholder for specific articles on managing JS framework churn)
- (Placeholder for risk management standards or software engineering texts)
Adaptability & Risk Mgmt Overview
(Placeholder: Simple graphic summarizing key areas: Agile, Tech Debt, Dependencies, Testing, CI/CD, Security, Change Mgmt)
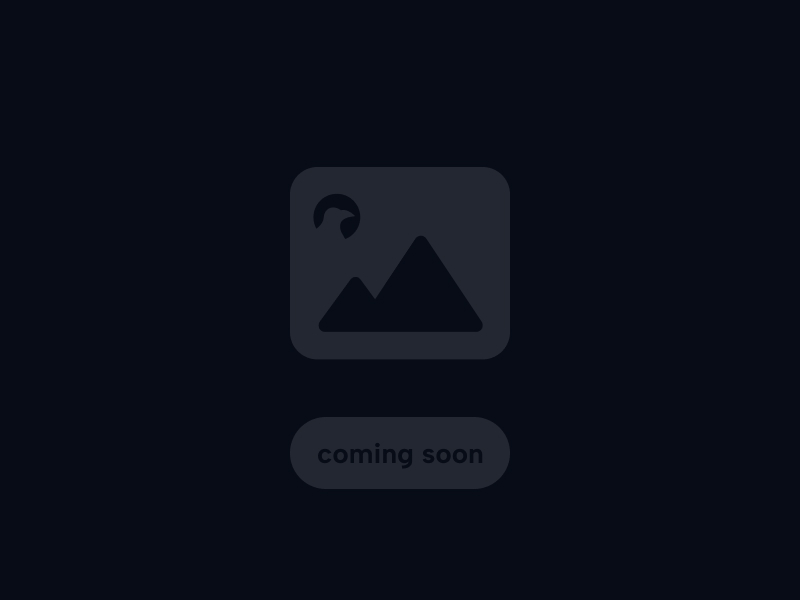