Understanding APIs: A JavaScript Developer's Guide
Demystify Application Programming Interfaces (APIs) and learn how they empower JavaScript developers to build dynamic, data-driven applications and interact with countless services.
This overview introduces APIs as crucial intermediaries enabling communication between different software components. We'll explore how JavaScript leverages APIs to fetch data, manipulate the browser, interact with servers, and utilize third-party functionalities.
Key takeaways focus on understanding common API types like REST and GraphQL, using the `Workspace` API in JavaScript, and recognizing the importance of APIs in the modern web development ecosystem.
1. What is an API? The Core Concept & Analogy
This section explains the fundamental concept of an API (Application Programming Interface) using a common analogy to make it accessible.
Objectively, an API is a set of definitions, protocols, and tools that allow different software applications or components to communicate and interact with each other. It defines the methods and data formats applications can use to request and exchange information.
Delving deeper with an analogy: Think of an API like a menu and a waiter in a restaurant. * The menu (API documentation) lists the available dishes (operations/data) you can order. * You (the client application, e.g., your JavaScript code) decide what you want from the menu. * The waiter (the API itself) takes your order (request), communicates it to the kitchen (the server or service providing the data/functionality), and brings back your dish (the response).
Further considerations emphasize that the API acts as an intermediary, hiding the complex internal workings of the "kitchen" (server/service) and providing a standardized way for the "customer" (client application) to get what it needs.
API stands for Application Programming Interface. At its core, an API is a contract or set of rules that defines how two pieces of software can talk to each other. It specifies what requests can be made, how to make them, the data formats that should be used, and what responses to expect.
Consider the popular restaurant analogy:
- You (the client software, e.g., your JavaScript web app) want some data or functionality.
- The menu (the API documentation) tells you what operations the kitchen (the server/service) can perform (e.g., "get weather data," "post a comment," "find user details").
- You give your order (an API request) to the waiter (the API endpoint/interface), specifying what you want based on the menu (e.g., "get weather for Mirabel").
- The waiter takes the request to the kitchen (the server), which processes it based on its internal logic and data.
- The waiter brings back your food (the API response), containing the requested information (e.g., the weather data for Mirabel).
The crucial point is that you, as the client, don't need to know *how* the kitchen prepares the food; you just need to know how to order from the menu via the waiter (API). APIs abstract away complexity, allowing different systems to work together seamlessly.
API Analogy: Restaurant Menu (Conceptual)
(Placeholder: Graphic showing Client -> Waiter (API) -> Kitchen (Server) interaction based on a Menu)
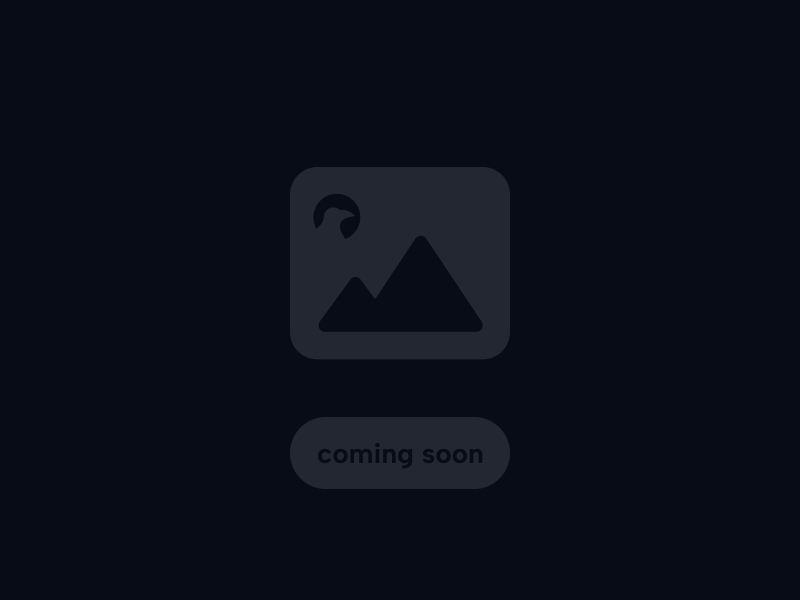
2. Why APIs Matter for JavaScript Developers
This section highlights the critical role APIs play in modern JavaScript development, enabling a wide range of functionalities.
Objectively, APIs allow JavaScript applications (running in browsers or on servers like Node.js) to interact with external data sources, services, and underlying platform capabilities without needing to know the intricate implementation details.
Delving deeper into key reasons: * Data Fetching: APIs are the standard way for front-end JavaScript (in the browser) to request data from back-end servers (e.g., user profiles, product lists, blog posts) to display dynamic content without full page reloads (AJAX technique). * Using Third-Party Services: Developers can integrate functionalities from external services like payment gateways (Stripe), mapping services (Google Maps, Mapbox), social media platforms (Twitter, Facebook), authentication services (Auth0), and more via their respective APIs. * Connecting Front-end and Back-end: APIs define the communication contract between separate front-end (React, Vue, Angular) and back-end (Node.js, Python, Java) applications. * Leveraging Platform Capabilities: JavaScript code uses built-in Browser APIs (like the DOM API to manipulate HTML, Fetch API for network requests, Geolocation API) and Node.js APIs (like the File System API, HTTP API) to interact with the runtime environment.
Further considerations emphasize that APIs promote modularity, enable faster development by reusing existing functionality, and fuel innovation by allowing developers to combine different services in novel ways.
APIs are fundamental tools for JavaScript developers, enabling them to build powerful and interactive applications:
- Fetching Dynamic Data: Front-end JavaScript code running in a browser uses APIs (typically REST or GraphQL) to communicate with back-end servers. This allows web pages to load data (like news feeds, product details, user comments) dynamically after the initial page load, creating richer user experiences (often referred to as AJAX - Asynchronous JavaScript and XML, though JSON is now standard).
- Integrating Third-Party Services: Modern applications rarely build everything from scratch. APIs allow developers to easily integrate external services:
- Payment processing (e.g., Stripe API, PayPal API)
- Mapping and location services (e.g., Google Maps API, Mapbox API)
- Social media integration (e.g., Twitter API, Facebook API)
- Weather data (e.g., OpenWeatherMap API)
- Authentication (e.g., Auth0 API, Firebase Authentication)
- And countless others...
- Decoupling Front-end and Back-end: In modern web architecture, the front-end (built with frameworks like React, Vue, Angular) is often separate from the back-end (built with Node.js, Python, Ruby, etc.). APIs define the clear contract for how these two parts communicate.
- Accessing Browser Features (Web APIs): JavaScript uses built-in Browser APIs to interact with the web browser itself, allowing manipulation of the web page (DOM API), making network requests (Fetch API), getting user location (Geolocation API), storing data locally (Web Storage API), and much more.
- Accessing Server/System Features (Node.js APIs): When using JavaScript on the server-side with Node.js, developers use built-in Node.js APIs to interact with the file system (`fs` module), create web servers (`http` module), handle network connections (`net` module), etc.
Essentially, APIs are the connective tissue that allows different software parts and services to work together, making complex applications possible.
API Use Cases in JavaScript (Conceptual)
(Placeholder: Diagram showing JS App connecting via APIs to Backend, 3rd Party Services, Browser Features, Node.js Features)
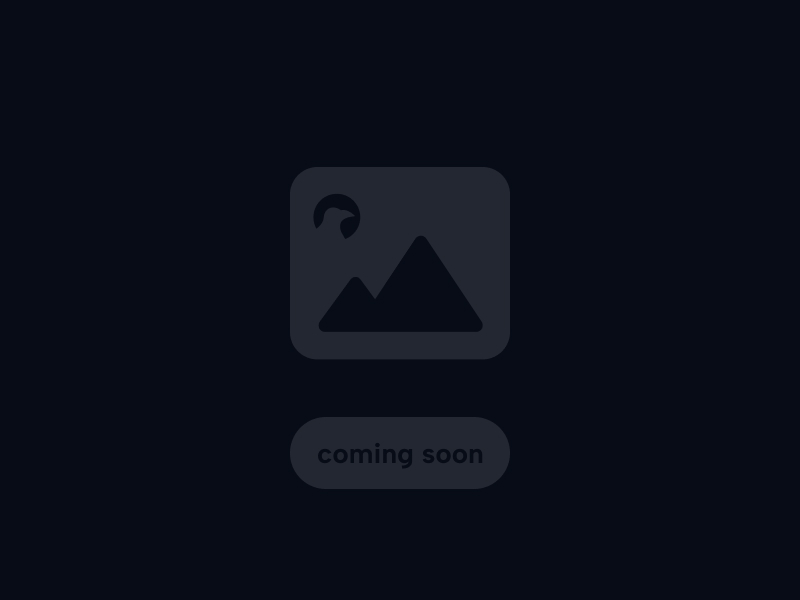
3. Types of APIs Used in JavaScript Development
This section categorizes the different kinds of APIs that JavaScript developers commonly encounter and utilize.
Objectively, APIs can be broadly classified based on their purpose, architecture, and the environment they operate in.
Delving deeper into key types relevant to JS: * Web APIs / Browser APIs: Interfaces built into web browsers, exposed via JavaScript objects (like `window`, `document`, `navigator`). They allow scripts to interact with the browser and operating system features. Examples: * DOM (Document Object Model) API: Manipulate HTML/CSS. * Fetch API / XMLHttpRequest: Make network requests. * Geolocation API: Access device location. * Web Storage API (localStorage, sessionStorage): Store data locally. * Console API (`console.log`, etc.). * Server-Side APIs (Web Service APIs): APIs exposed by web servers over the network (usually HTTP) to allow client applications (like browser JS or other servers) to interact with them. Common architectural styles include: * REST (Representational State Transfer): A widely used architectural style using standard HTTP methods (GET, POST, PUT, DELETE) to interact with resources identified by URLs. Typically uses JSON. * GraphQL: A query language and runtime for APIs, allowing clients to request exactly the data they need in a single request via a single endpoint. * Others (SOAP - older, gRPC - high performance). * Node.js APIs: Built-in modules provided by the Node.js runtime for server-side JavaScript development, allowing interaction with the operating system and network. Examples: * `fs` (File System) module: Read/write files. * `http` / `https` modules: Create HTTP(S) servers and clients. * `path` module: Handle file paths. * `os` module: Get operating system information.
Further considerations include Third-Party APIs, which can be any of the above types but are provided by external companies or services.
JavaScript developers work with various types of APIs depending on the context (browser or server) and the task at hand:
- Web APIs / Browser APIs:
- Definition: These are APIs built directly into the web browser, providing JavaScript access to browser features and the user's environment. They are not part of the core JavaScript language itself but are provided by the browser runtime.
- Examples:
- DOM API: Allows manipulation of the HTML document structure, style, and content (e.g., `document.getElementById()`, `element.innerHTML`, `element.style`).
- Fetch API / XMLHttpRequest: Used to make network requests to servers (fetch data, submit forms).
- Geolocation API: Accesses the user's geographical location (with permission).
- Web Storage API: Provides `localStorage` and `sessionStorage` for storing key-value data in the browser.
- Console API: Used for logging messages to the browser's developer console (e.g., `console.log()`).
- Canvas API, Web Audio API, WebGL API: For graphics and multimedia.
- Accessibility: Available directly in client-side JavaScript running in a browser.
- Server-Side APIs (Web Service APIs):
- Definition: These APIs are hosted on remote servers and accessed over the network (usually HTTP/HTTPS). They allow client applications (like your browser JavaScript, mobile apps, or other servers) to interact with the server's data and functionality.
- Common Architectural Styles:
- REST (Representational State Transfer): The most prevalent style. Uses standard HTTP methods (GET, POST, PUT, DELETE, etc.) and URLs to interact with resources. Typically exchanges data using JSON.
- GraphQL: A query language for APIs. Allows clients to request precisely the data they need from a single endpoint, reducing over-fetching. Uses POST requests typically.
- SOAP (Simple Object Access Protocol): An older protocol, often using XML. Less common for new web APIs.
- gRPC: A high-performance framework often used for microservices communication.
- Accessibility: Accessed from JavaScript using network request mechanisms like the Fetch API.
- Node.js APIs:
- Definition: Built-in modules provided by the Node.js runtime environment for server-side JavaScript. They allow interaction with the server's operating system, file system, network, etc.
- Examples:
- `fs` Module: For file system operations (reading/writing files).
- `http` / `https` Modules: For creating web servers and making HTTP requests from the server.
- `path` Module: For working with file and directory paths.
- `os` Module: Provides operating system-related utility methods.
- Accessibility: Available only when running JavaScript within the Node.js environment.
- Third-Party APIs: APIs provided by external companies (Google, Stripe, Twitter, etc.) that fall into the categories above (usually Server-Side APIs).
API Types in JavaScript Context (Conceptual)
(Placeholder: Diagram showing Browser JS using Browser/Web APIs & Server APIs, Node.js using Node APIs & Server APIs)
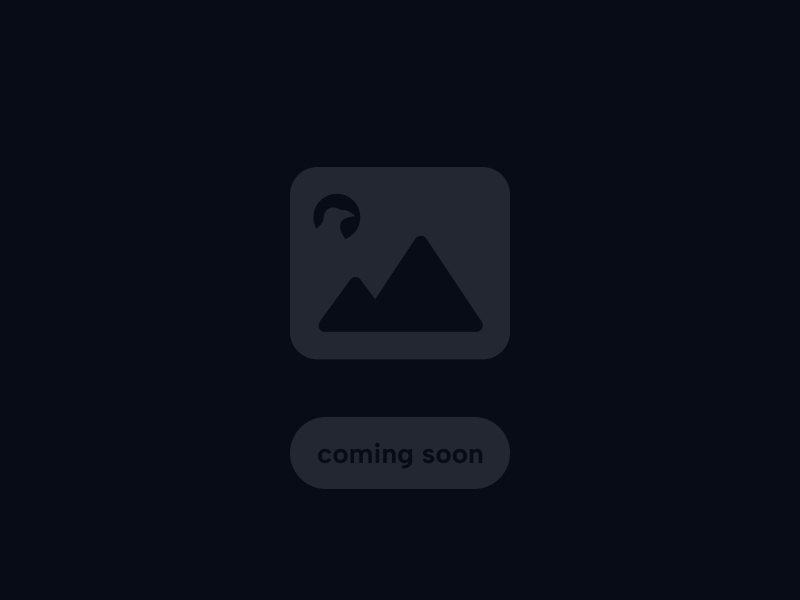
4. REST APIs Explained: Core Principles
This section focuses on REST (Representational State Transfer), the most common architectural style for building web service APIs used by JavaScript applications.
Objectively, REST is not a strict protocol but a set of architectural constraints or principles for designing networked applications, leveraging the existing HTTP protocol.
Delving deeper into key REST principles: * Client-Server Architecture: Separation of concerns between the client (requesting resources) and the server (managing resources). * Statelessness: Each request from a client to the server must contain all the information needed to understand and process the request. The server does not store any client context (session state) between requests. * Cacheability: Responses should be explicitly marked as cacheable or non-cacheable to improve performance and scalability. * Uniform Interface: A consistent way of interacting with resources, typically using: * Resource Identification via URIs: Resources (e.g., users, products) are identified by unique URLs (e.g., `/users`, `/products/123`). * Resource Manipulation Through Representations: Clients interact with representations of resources, commonly using JSON (JavaScript Object Notation) as the data format. * Standard HTTP Methods (Verbs): Using standard HTTP verbs for actions: * `GET`: Retrieve a resource. * `POST`: Create a new resource. * `PUT`: Update/replace an existing resource. * `DELETE`: Remove a resource. * `PATCH`: Partially update an existing resource. * Self-descriptive Messages & HATEOAS (optional): Responses ideally contain information on how to interact further (Hypermedia as the Engine of Application State).
Further considerations emphasize REST's simplicity, scalability, and reliance on established web standards (HTTP, URLs, JSON), making it widely adopted.
REST (Representational State Transfer) is an architectural style, not a strict protocol like SOAP. It defines a set of constraints for designing networked applications, particularly web services. APIs designed following these principles are called RESTful APIs.
Key principles of REST relevant to JavaScript developers consuming them:
- Client-Server Separation: The client (e.g., your JS application) handles the user interface and user experience, while the server handles data storage and logic. They communicate via HTTP requests/responses.
- Statelessness: Each request sent from the client to the server must contain all necessary information for the server to fulfill it. The server doesn't store any session state about the client between requests. If state is needed (like user login), it's typically managed by the client (e.g., sending an authentication token with each request).
- Uniform Interface: This is central to REST and typically involves:
- Resource-Based: APIs are designed around *resources* (e.g., users, products, articles). Each resource has a unique identifier, usually a URL (Uniform Resource Locator), like `/api/users` or `/api/products/42`.
- Standard HTTP Methods: Actions on resources are performed using standard HTTP verbs:
- `GET`: Retrieve data (e.g., `GET /api/users/123` to get user 123).
- `POST`: Create a new resource (e.g., `POST /api/users` with user data in the request body).
- `PUT`: Update or replace an existing resource completely (e.g., `PUT /api/users/123` with updated user data).
- `DELETE`: Remove a resource (e.g., `DELETE /api/users/123`).
- `PATCH`: Partially update an existing resource.
- Representations: Resources are exchanged using representations, most commonly JSON (JavaScript Object Notation) due to its simplicity and direct mapping to JavaScript objects. XML is another possibility.
- Cacheability: Responses from the server should indicate whether they can be cached by the client or intermediaries to improve performance.
- Layered System (Optional): Architecture can involve multiple layers (proxies, load balancers) without the client knowing.
REST's reliance on standard HTTP methods and easy-to-parse formats like JSON makes it relatively simple to consume from JavaScript.
REST API: Resource & HTTP Verb Interaction (Conceptual)
(Placeholder: Diagram showing URL `/users/123` being acted upon by GET, PUT, DELETE verbs)
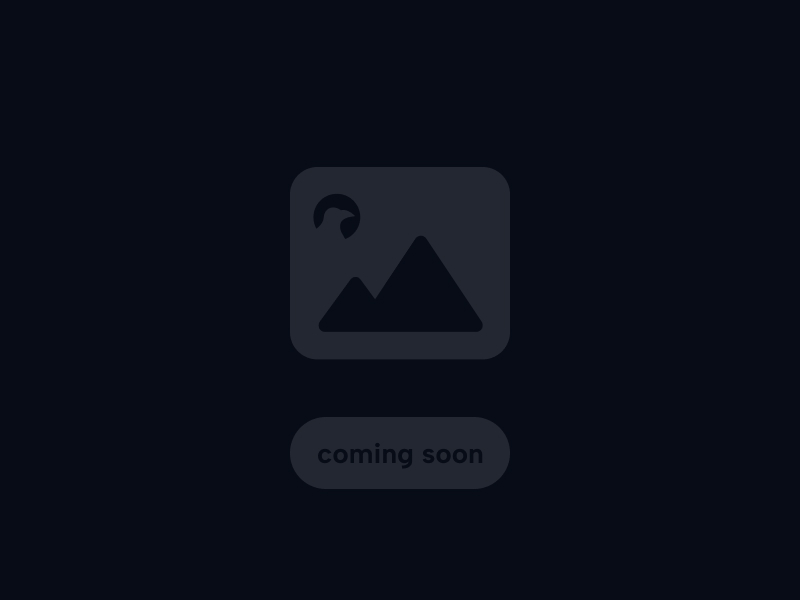
5. Consuming REST APIs with JavaScript (`Workspace`)
This section provides practical examples of how to make requests to REST APIs from JavaScript code running in the browser, primarily using the modern `Workspace` API.
Objectively, the `Workspace` API provides a flexible and Promise-based interface for making network requests (including to REST APIs).
Delving deeper into `Workspace` usage: * Basic GET Request: Calling `Workspace(url)` returns a Promise that resolves to a `Response` object. * Handling the Response: Check `response.ok` (or `response.status`) to see if the request was successful (e.g., status 200 OK). Use methods like `response.json()` (returns another Promise) to parse the response body as JSON, or `response.text()` for plain text. * Using Promises (`.then()`, `.catch()`): Chain `.then()` blocks to process the successful response and `.catch()` to handle errors (network errors or errors thrown during processing). * Using `async/await`: Provides a cleaner, more synchronous-looking syntax for working with the Promises returned by `Workspace` and `response.json()`. Use `try...catch` blocks for error handling. * Making Other Requests (POST, PUT, DELETE): Pass a second argument (an options object) to `Workspace` specifying the `method`, `headers` (e.g., `'Content-Type': 'application/json'`), and `body` (often using `JSON.stringify()` for sending JSON data).
Further considerations briefly mention the older `XMLHttpRequest` (XHR) object, which was used before `Workspace` but is more complex and less ergonomic.
The standard way to interact with REST APIs in modern browser-based JavaScript is using the `Workspace` API. It's built-in and uses Promises, making asynchronous requests easier to manage.
Basic GET Request with Promises:
const apiUrl = 'https://jsonplaceholder.typicode.com/posts/1'; // Example REST API endpoint
fetch(apiUrl)
.then(response => {
// Check if the request was successful (status code 200-299)
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
// Parse the response body as JSON (returns another promise)
return response.json();
})
.then(data => {
// Work with the JSON data
console.log('Data fetched:', data);
// Example: Display title on the page
// document.getElementById('title').textContent = data.title;
})
.catch(error => {
// Handle any errors that occurred during the fetch
console.error('Error fetching data:', error);
// Example: Display error message on the page
// document.getElementById('error').textContent = `Failed to load data: ${error}`;
});
Same GET Request with `async/await`:
`async/await` provides syntactic sugar over Promises, making asynchronous code look more like synchronous code.
const apiUrlAsync = 'https://jsonplaceholder.typicode.com/posts/2'; // Another example endpoint
async function fetchData() {
try {
const response = await fetch(apiUrlAsync);
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const data = await response.json(); // Wait for JSON parsing
console.log('Data fetched (async/await):', data);
// Process data here...
} catch (error) {
console.error('Error fetching data (async/await):', error);
// Handle errors here...
}
}
fetchData(); // Call the async function
Making a POST Request (Sending Data):
const postUrl = 'https://jsonplaceholder.typicode.com/posts'; // Endpoint for creating posts
const newData = {
title: 'foo',
body: 'bar',
userId: 1,
};
fetch(postUrl, {
method: 'POST', // Specify the method
headers: {
'Content-Type': 'application/json', // Indicate we're sending JSON
},
body: JSON.stringify(newData), // Convert the JS object to a JSON string
})
.then(response => {
if (!response.ok) {
// Check for server-side errors (e.g., 4xx, 5xx)
throw new Error(`HTTP error! status: ${response.status}`);
}
// Check if the response indicates success (e.g., status 201 Created)
console.log('POST Response Status:', response.status);
return response.json();
})
.then(data => {
console.log('Success:', data); // Log the response from the server (often the created resource)
})
.catch(error => {
console.error('Error during POST:', error);
});
Similar options objects can be used for `PUT`, `DELETE`, `PATCH` methods by changing the `method` property and including a `body` if necessary.
*(Note: `XMLHttpRequest` (XHR) is the older way to make requests. While still supported, `Workspace` is generally preferred for new development due to its cleaner Promise-based API).*
6. Introduction to GraphQL APIs
This section provides a brief introduction to GraphQL, an alternative approach to building and consuming APIs, contrasting it with REST.
Objectively, GraphQL is a query language for APIs and a runtime for fulfilling those queries with existing data. It allows clients to ask for exactly the data they need and nothing more.
Delving deeper into key GraphQL concepts: * Single Endpoint: Unlike REST's multiple URLs for different resources, GraphQL APIs typically expose a single endpoint (usually via HTTP POST). * Query Language: Clients send structured queries specifying the exact fields and relationships they need. * Schema & Types: GraphQL APIs are strongly typed. The server defines a schema describing the available data types and their relationships. * No Over/Under-fetching: Because clients specify required data precisely, they avoid receiving unnecessary data (over-fetching) or needing multiple requests to gather related data (under-fetching), common issues with REST. * Mutations & Subscriptions: GraphQL defines specific operations for modifying data (Mutations) and receiving real-time updates (Subscriptions).
Further considerations mention that consuming GraphQL in JavaScript often involves using dedicated client libraries (like Apollo Client, Relay, or urql) that simplify query construction, caching, and state management, although basic requests can be made with `Workspace`.
GraphQL is an increasingly popular alternative to REST for API development. Developed by Facebook and now open-source, it offers a different approach focused on client data needs.
Key differences and concepts compared to REST:
- Query Language: GraphQL *is* a query language. Clients send a query specifying the exact data structure and fields they require.
- Single Endpoint: Typically, all GraphQL requests (queries for data, mutations for changes) are sent to a *single* URL endpoint (usually using HTTP POST).
- Strongly Typed Schema: GraphQL APIs are defined by a schema that describes the types of data available, their fields, and relationships. This enables powerful developer tools and validation.
- No Over-fetching or Under-fetching: The client dictates the response shape. You only get the data you explicitly ask for in the query, solving REST's common problem of endpoints returning too much or too little data for a specific UI component.
- Operations:
- Query: Used for fetching data (read operations).
- Mutation: Used for modifying data (create, update, delete operations).
- Subscription: Used for real-time updates pushed from the server to the client over a persistent connection (e.g., WebSockets).
While you can technically send GraphQL queries using `Workspace` (as POST requests with the query in the body), JavaScript developers typically use specialized GraphQL client libraries (like Apollo Client, urql, Relay) which provide features like caching, state management, code generation based on the schema, and simplified handling of queries, mutations, and subscriptions.
# Example GraphQL Query (Sent in request body, often as JSON)
query GetUserDetails {
user(id: "123") {
id
name
email
posts { # Get related posts
title
}
}
}
# Example GraphQL Mutation
mutation CreatePost {
createPost(title: "New Post Title", content: "...") {
id # Return the ID of the newly created post
title
}
}
REST vs. GraphQL: Data Fetching (Conceptual)
(Placeholder: Diagram showing REST potentially needing multiple requests or getting extra data, vs GraphQL getting exact data in one request)
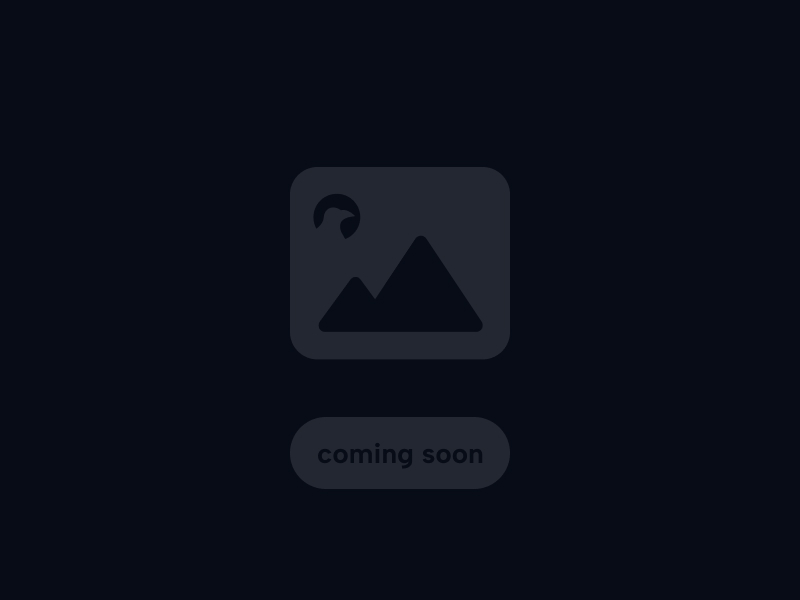
7. Browser APIs: Interacting with the Browser Environment
This section revisits Browser APIs (also called Web APIs), focusing on how JavaScript uses them to interact with the user's browser and device capabilities.
Objectively, these APIs are provided by the browser itself, not the JavaScript language core, and expose functionalities related to the document, window, user hardware, and network communication.
Delving deeper with examples: * DOM API: Essential for web development. Allows JavaScript to read and modify the HTML structure and CSS styles of a web page dynamically (e.g., `document.getElementById()`, `element.createElement()`, `element.addEventListener()`, `element.style`). * Geolocation API: Used via `navigator.geolocation` to request the user's current physical location (requires user permission). Methods like `getCurrentPosition()`. * Web Storage API: Provides `localStorage` (persistent storage) and `sessionStorage` (session-only storage) for storing key-value pairs directly in the browser. Methods like `setItem()`, `getItem()`, `removeItem()`. * Fetch API: Already discussed, used for making network requests.
Further considerations highlight the vast number of available Browser APIs (Console, Canvas, Web Audio, Web Workers, etc.) listed on resources like MDN, enabling rich web application features.
Browser APIs (or Web APIs) are interfaces built into web browsers, providing JavaScript with access to features of the browser and the user's computer environment. These are crucial for creating interactive and dynamic web pages.
Key examples frequently used by JavaScript developers:
- DOM (Document Object Model) API:
- Allows you to access and manipulate the HTML and CSS of the current webpage.
- You can find elements (`document.getElementById`, `document.querySelector`), change their content (`element.textContent`, `element.innerHTML`), modify styles (`element.style.color`), add or remove elements, and respond to user events (`element.addEventListener`).
- Fundamental for making web pages interactive.
- Fetch API:
- Used to make asynchronous network requests to servers, typically to get data from or send data to REST or GraphQL APIs. (Covered in Section 5).
- Geolocation API:
- Allows web applications to request the user's geographical location (latitude and longitude), after obtaining explicit permission.
- Accessed via the `navigator.geolocation` object (e.g., `navigator.geolocation.getCurrentPosition(successCallback, errorCallback)`).
- Web Storage API:
- Provides mechanisms for storing key-value pairs locally in the user's browser.
- `localStorage`: Stores data persistently even after the browser is closed.
- `sessionStorage`: Stores data only for the duration of the browser session.
- Accessed via `localStorage.setItem('key', 'value')`, `localStorage.getItem('key')`, etc.
- Console API:
- Used for logging information to the browser's developer console for debugging purposes (e.g., `console.log()`, `console.error()`, `console.warn()`).
Many other powerful Browser APIs exist for handling audio/video, graphics (Canvas, WebGL), background tasks (Web Workers), real-time communication (WebSockets), device hardware access (with permissions), and more. MDN Web Docs is the best resource for exploring these.
// Example: DOM API Manipulation
const myButton = document.getElementById('myButton');
const messageDiv = document.getElementById('message');
if (myButton && messageDiv) {
myButton.addEventListener('click', () => {
messageDiv.textContent = 'Button was clicked!';
messageDiv.style.color = 'blue';
});
}
// Example: Using localStorage
localStorage.setItem('username', 'QwireyUser');
const storedName = localStorage.getItem('username');
console.log(`Stored username: ${storedName}`); // Output: Stored username: QwireyUser
8. Node.js APIs: Server-Side JavaScript Capabilities
This section introduces the concept of built-in APIs provided by the Node.js runtime environment, enabling JavaScript to perform server-side tasks.
Objectively, Node.js allows JavaScript execution outside the browser and provides a rich set of modules (APIs) for interacting with the server's operating system, file system, network, and more, focusing on asynchronous, non-blocking I/O.
Delving deeper with examples: * `fs` (File System) Module: Provides functions for interacting with the file system, such as reading files (`fs.readFile`), writing files (`fs.writeFile`), checking file existence, creating directories, etc. Often has both synchronous and asynchronous (callback or Promise-based) versions. * `http` Module: Allows creating HTTP servers to handle incoming requests and send responses, forming the basis for web applications and APIs built with Node.js. Can also be used to make HTTP requests from the server. * `path` Module: Provides utilities for working with file and directory paths in a cross-platform way. * `os` Module: Offers methods to retrieve information about the operating system (CPU architecture, platform, free memory).
Further considerations highlight that Node.js APIs are essential for back-end JavaScript development, enabling tasks not possible within the security sandbox of a web browser.
When JavaScript runs on the server using the Node.js runtime environment, it gains access to a different set of powerful APIs designed for back-end tasks. These APIs allow JavaScript to interact directly with the server's operating system, file system, and network capabilities.
Key built-in Node.js modules (APIs):
- `fs` (File System):
- Provides functions to interact with the file system.
- Common methods: `fs.readFile()` (asynchronously read a file), `fs.writeFile()` (asynchronously write to a file), `fs.readFileSync()` (synchronous read), `fs.stat()` (get file stats), `fs.mkdir()` (create directory).
- Modern versions often provide Promise-based alternatives (e.g., `require('fs').promises`).
- `http` / `https`:
- Core modules for networking.
- `http.createServer()`: Used to create an HTTP server that listens for incoming requests and sends responses. This is fundamental for building web servers and APIs with Node.js (often used via frameworks like Express.js).
- `http.request()` / `https.request()`: Used to make HTTP(S) requests *from* the Node.js server to other servers or APIs.
- `path`:
- Provides utilities for working with file and directory paths reliably across different operating systems (Windows, macOS, Linux).
- Methods like `path.join()`, `path.resolve()`, `path.basename()`.
- `os`:
- Provides methods to retrieve information about the host operating system, such as `os.platform()`, `os.arch()`, `os.cpus()`, `os.freemem()`.
These APIs, combined with the vast ecosystem of third-party packages available via npm (Node Package Manager), make Node.js a powerful platform for building scalable server-side applications, APIs, command-line tools, and more with JavaScript.
// Example: Reading a file using Node.js fs module (async with callback)
const fs = require('fs'); // Import the built-in module
fs.readFile('./myFile.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File content:', data);
});
// Example: Basic HTTP server using Node.js http module
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello from Node.js Server!\n');
});
const PORT = 3000;
// server.listen(PORT, () => { // Uncomment to run locally
// console.log(`Server running at http://localhost:${PORT}/`);
// });
9. API Keys, Authentication & Security Basics
This section briefly touches upon the crucial aspect of security when working with APIs, particularly third-party or server-side APIs.
Objectively, many APIs, especially those providing access to sensitive data or paid services, require authentication and authorization mechanisms to control access and track usage.
Delving deeper into common security concepts:
* API Keys: Simple tokens provided by the API provider that developers include in their requests to identify their application and track usage. Often used for public data APIs or basic rate limiting. *Should generally be kept secret and not exposed in client-side code.*
* Authentication: Verifying the identity of the client making the request. Common methods include:
* Tokens (e.g., JWT - JSON Web Tokens, OAuth Tokens): After logging in or authorizing, the client receives a token to include in subsequent requests (often in the `Authorization` header, e.g., `Authorization: Bearer
Further considerations emphasize the importance of following the API provider's security guidelines, protecting keys and tokens, and being mindful of security best practices when building or consuming APIs.
When consuming APIs, especially third-party or private ones, security is paramount. APIs often control access to valuable data or functionality, so mechanisms are needed to identify and authorize users/applications.
Common security concepts JavaScript developers encounter:
- API Keys:
- A unique string provided by the API service to identify your application.
- Usually sent with each request (e.g., as a query parameter `?apiKey=YOUR_KEY` or in an HTTP header like `X-API-Key`).
- Mainly used for identification, usage tracking, and basic rate limiting.
- Crucial: API keys often need to be kept secret. Exposing them in client-side JavaScript source code is generally insecure. They should ideally be used from a server-side application or via a proxy server that adds the key securely.
- Authentication: Verifying who the user or application is.
- Token-Based Authentication (Common): After initial login or authorization (often via OAuth 2.0 flow), the client receives a temporary access token (like a JWT or opaque token). This token is sent with subsequent API requests, typically in the `Authorization` HTTP header (e.g., `Authorization: Bearer YOUR_ACCESS_TOKEN`). The server validates the token to authenticate the request.
- OAuth 2.0: A standard framework for delegated authorization. Allows users to grant limited access to their data on one site (e.g., Google, Facebook) to another application without sharing their password. Often used to get access tokens.
- Other methods: Basic Authentication (less secure), session cookies, etc.
- Authorization: Determining what an authenticated user/application is *allowed* to do (permissions). This is often handled server-side based on the authenticated identity or token scopes.
- HTTPS: Always interact with APIs over HTTPS to encrypt the communication channel, protecting API keys, tokens, and data from eavesdropping.
Always consult the specific API's documentation for its required authentication and security procedures and handle credentials securely.
API Security Concepts (Conceptual Icons)
(Placeholder: Icons: API Key (Key), Token (Ticket/Badge), HTTPS (Lock))
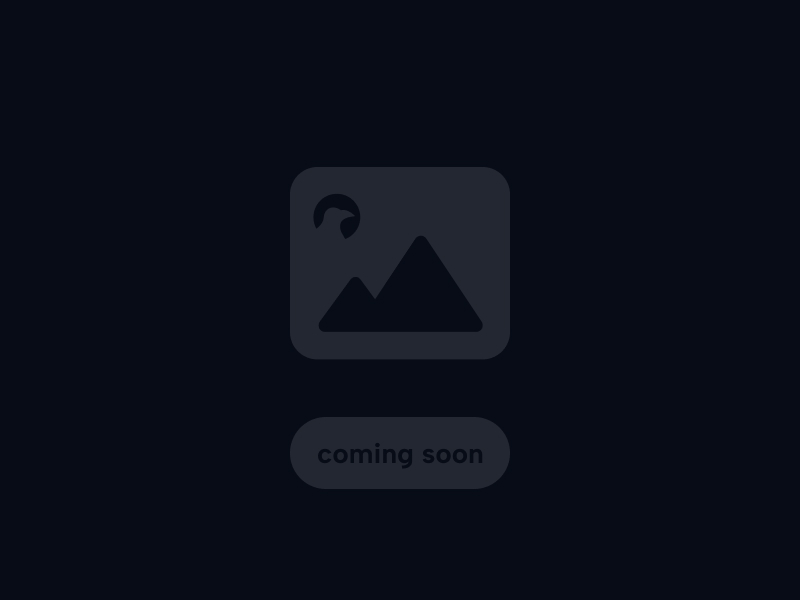
10. Conclusion & Resources
This concluding section summarizes the role of APIs in JavaScript development and provides pointers for further exploration.
Objectively, APIs are fundamental building blocks in modern web and server-side development with JavaScript. They enable interaction between disparate systems, access to vast amounts of data and functionality, and the creation of rich, dynamic applications.
Delving deeper, mastering how to consume various types of APIs (especially REST and increasingly GraphQL) using tools like the `Workspace` API and understanding asynchronous concepts (Promises, async/await) are essential skills for JavaScript developers.
Further considerations encourage developers to explore specific Browser APIs and Node.js APIs relevant to their projects, practice consuming public APIs, and consult authoritative documentation like MDN Web Docs.
Conclusion: APIs - The Bridge for Modern JavaScript Applications
Application Programming Interfaces are the essential bridges that connect JavaScript applications to data, services, and platform capabilities. Whether you're building interactive front-ends that fetch dynamic content, integrating third-party functionalities, manipulating the browser environment, or creating robust back-ends with Node.js, understanding and utilizing APIs is a core competency.
From the ubiquitous REST APIs consumed with `Workspace` or `async/await`, to the flexible querying of GraphQL, to the power of built-in Browser and Node.js APIs, these interfaces unlock immense potential. As a JavaScript developer, becoming proficient in finding, understanding, and consuming APIs securely is key to building modern, feature-rich applications.
Key API & JavaScript Resources
Core JavaScript & Web APIs:
- MDN Web Docs - Web APIs: The comprehensive reference for Browser APIs. MDN Web APIs
- MDN Web Docs - Fetch API: Detailed guide and reference. MDN Fetch API
- MDN Web Docs - Introduction to Web APIs: A good starting point. MDN Intro to Web APIs
REST & GraphQL:
- REST API Tutorials: Many available online (search for specific frameworks or general concepts).
- GraphQL Official Website: Learn about GraphQL concepts and ecosystem. graphql.org
- HowToGraphQL: Tutorials for learning GraphQL. howtographql.com
Node.js:
- Node.js Official Documentation: API reference for built-in modules. nodejs.org/api/
Public APIs:
- Public APIs Repository (GitHub): A curated list of free APIs for practice and projects. GitHub Public APIs List
References (Placeholder)
Links to specific RFCs (like HTTP spec) or foundational papers could be added.
- Fielding, Roy T. (2000). Architectural Styles and the Design of Network-based Software Architectures (Dissertation defining REST).
- MDN Web Docs (Specific pages for DOM, Geolocation, etc.)
- Node.js API Documentation (Specific module pages like `fs`, `http`).
API Guide Overview
(Placeholder: Simple graphic summarizing key guide sections - What, Why, Types, REST, Fetch, GraphQL, Browser/Node)
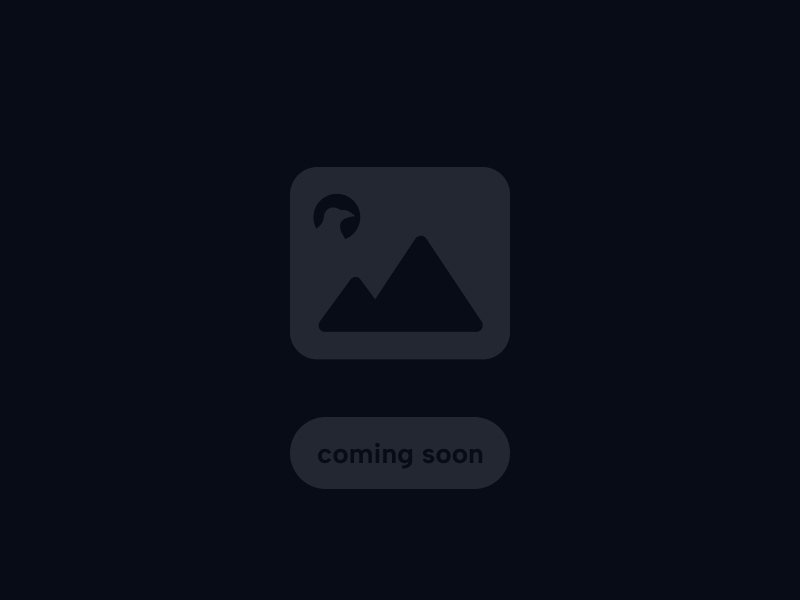