JavaScript Fundamentals: Your First Steps in Web Programming
Welcome to JavaScript! This guide breaks down the essential building blocks of the language, helping you start your journey into web development and interactive websites.
Explore core concepts like variables, data types, operators, control flow (if/else, loops), and functions in simple terms with clear examples.
Key takeaways focus on understanding how JavaScript brings websites to life, learning the basic syntax, and building a foundation for more advanced topics.
1. What is JavaScript & Its Role in Web Development?
This section introduces JavaScript and explains its primary function in creating websites and web applications.
Objectively, JavaScript (often abbreviated as JS) is a high-level, interpreted programming language primarily used for creating interactive effects within web browsers. Alongside HTML (structure) and CSS (style), it forms the core technologies of the World Wide Web.
Delving deeper, while HTML defines the content (text, images) and CSS defines the layout and appearance (colors, fonts), JavaScript adds behavior and interactivity. Think of things like dropdown menus, image sliders, form validation, dynamic content updates without reloading the page, and much more.
Further considerations mention that JavaScript's role has expanded significantly beyond browsers. With environments like Node.js, it's now also widely used for server-side development, mobile apps, game development, and other areas, making it a versatile and valuable language to learn.
Welcome to the world of JavaScript! If you've ever interacted with a website – clicked a button that changed something, filled out a form that gave you instant feedback, or seen animations – you've likely encountered JavaScript in action.
Think of building a website like building a house:
- HTML (HyperText Markup Language): Provides the basic structure – the walls, rooms, doors (paragraphs, headings, images).
- CSS (Cascading Style Sheets): Adds the style and appearance – the paint color, furniture arrangement, decorations (layout, fonts, colors).
- JavaScript (JS): Makes the house interactive and functional – turning on lights, opening doors automatically, having appliances respond to you (making webpage elements interactive, validating forms, fetching data).
Initially created to run *inside* web browsers (client-side scripting), JavaScript's role has grown immensely. It's now a versatile language used for building complex web applications, server-side applications (with Node.js), mobile apps, and more. Learning the fundamentals is the first step towards mastering this powerful language.
The Web Trinity (Conceptual)
(Placeholder: Simple graphic showing HTML (Structure) + CSS (Style) + JS (Behavior) = Webpage)
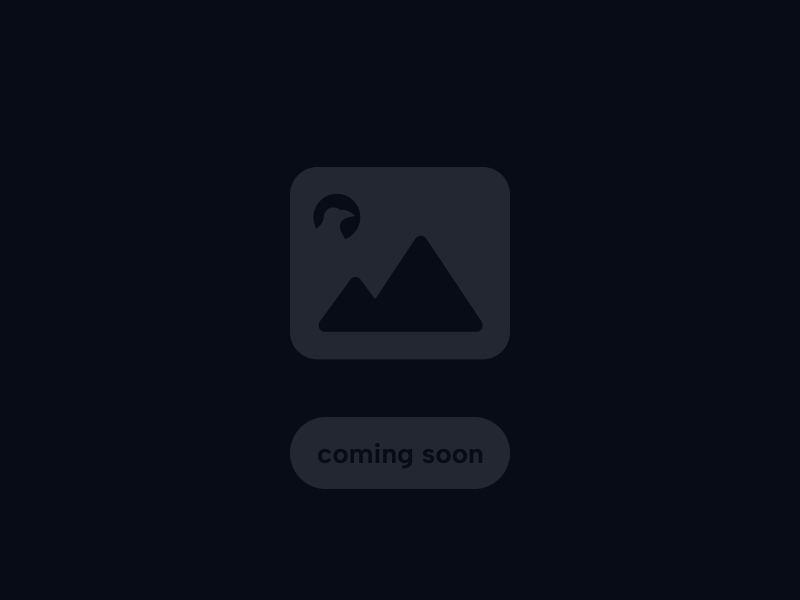
2. Running Your First JavaScript Code
This section explains the simplest ways for a beginner to start writing and executing JavaScript code without complex setup.
Objectively, JavaScript code can be executed directly within modern web browsers or through dedicated runtime environments like Node.js.
Delving deeper into beginner-friendly methods: * Browser Developer Console: Almost all web browsers (Chrome, Firefox, Edge, Safari) have built-in developer tools, including a "Console". You can type simple JavaScript commands directly into the console and see immediate results. This is great for quick experiments. (How to open: Usually F12 key or right-click -> Inspect -> Console tab). * HTML `` tags, or link to an external JavaScript file (`.js`) using ``. When you open the HTML file in a browser, the JavaScript code runs.
Further considerations mention online code editors (like CodePen, JSFiddle) as easy ways to experiment without local setup, and briefly touch upon Node.js for running JS outside the browser (more advanced).
The great thing about JavaScript is that you don't need fancy software to start running it! You likely already have everything you need:
- Using the Browser Developer Console:
- Open your web browser (like Chrome, Firefox, Edge).
- Right-click anywhere on a webpage and select "Inspect" or "Inspect Element".
- Find and click on the "Console" tab in the developer tools panel that appears.
- You can now type JavaScript commands directly here and press Enter to see the result! Try typing `console.log("Hello, World!");` or `2 + 2`.
- Using an HTML File:
- Create a simple text file and save it with an `.html` extension (e.g., `index.html`).
- Inside the file, you can write basic HTML structure and include JavaScript within ``. This is better for larger projects.
- Online Playgrounds: Websites like CodePen, JSFiddle, or Replit let you write and run HTML, CSS, and JS directly in your browser without any setup.
For now, using the browser console or simple HTML files is the easiest way to follow along with the examples.
3. Variables & Basic Data Types
This section introduces the fundamental concepts of variables for storing data and the basic types of data JavaScript can handle.
Objectively, variables are like named containers used to store information (values) that can be referenced and manipulated in a program. In modern JavaScript (ES6+), variables are typically declared using `let` or `const`.
Delving deeper into basic data types (Primitives): * String: Represents textual data, enclosed in quotes (e.g., `"Hello"`, `'World'`). * Number: Represents numerical data, including integers and decimals (e.g., `42`, `3.14`). * Boolean: Represents logical values, either `true` or `false`. * Undefined: Represents a variable that has been declared but not yet assigned a value. * Null: Represents the intentional absence of any object value. It's an assigned value meaning "no value". * (Briefly mention Symbol and BigInt as more advanced primitive types).
Further considerations explain that JavaScript is dynamically typed, meaning you don't explicitly declare the *type* of a variable; its type is determined automatically based on the value assigned to it.
In programming, we need ways to store and label pieces of information. We use variables for this. Think of a variable as a labeled box where you can put data.
In modern JavaScript, we primarily use `let` and `const` to declare variables:
- `let`: Use this when you expect the value stored in the variable might need to change later.
- `const`: Use this when the value should not change (it's constant) after you set it initially. It's generally good practice to use `const` by default unless you know you'll need to reassign the variable.
Variables can hold different types of data. The basic ("primitive") data types in JavaScript are:
- String: Textual data. Always wrap strings in single quotes (`'...'`), double quotes (`"..."`), or backticks (``` `...` ```).
Example: `let greeting = "Hello there!";` - Number: Any kind of number, including whole numbers (integers) and decimals (floating-point numbers).
Example: `let userAge = 30; const price = 99.95;` - Boolean: Represents a logical value - either `true` or `false`. Often used for making decisions in code.
Example: `const isLoggedIn = true; let hasPermission = false;` - Undefined: A variable that has been declared but hasn't been given a value yet automatically has the value `undefined`.
Example: `let userCity; console.log(userCity); // Output: undefined` - Null: Represents the intentional absence of a value. It's different from `undefined`; `null` is explicitly assigned to mean "no value".
Example: `let selectedProduct = null;` - *(Others: `Symbol` and `BigInt` are more advanced primitive types you'll encounter later.)*
JavaScript is dynamically typed, meaning you don't have to specify the data type when declaring a variable. The type is determined by the value you assign.
let message = "Learning JS"; // message is a String
const currentYear = 2025; // currentYear is a Number
let isComplete = false; // isComplete is a Boolean
console.log(typeof message); // Output: string
console.log(typeof currentYear); // Output: number
console.log(typeof isComplete); // Output: boolean
message = 100; // You can change the value AND type with 'let'
console.log(typeof message); // Output: number
4. Basic Operators in JavaScript
This section introduces operators – special symbols used to perform operations on values (operands).
Objectively, operators are fundamental for performing calculations, assignments, comparisons, and logical evaluations in code.
Delving deeper into common operator types: * Arithmetic Operators: Perform mathematical calculations: `+` (addition), `-` (subtraction), `*` (multiplication), `/` (division), `%` (modulo - remainder of division). * Assignment Operators: Assign values to variables: `=` (simple assignment), `+=`, `-=`, `*=`, `/=` (shorthand for performing an operation and assigning the result). * Comparison Operators: Compare two values and return a boolean (`true` or `false`): `==` (equal value), `===` (equal value AND type - preferred), `!=` (not equal value), `!==` (not equal value OR type - preferred), `>` (greater than), `<` (less than), `>=` (greater than or equal), `<=` (less than or equal). * Logical Operators: Combine boolean expressions: `&&` (logical AND - true if both sides are true), `||` (logical OR - true if at least one side is true), `!` (logical NOT - inverts boolean value).
Further considerations mention operator precedence (order of operations, like multiplication before addition) and associativity.
Operators are the symbols that perform actions on your data (variables and values, called operands).
- Arithmetic Operators: For doing math.
- `+` Addition (also used for string concatenation)
- `-` Subtraction
- `*` Multiplication
- `/` Division
- `%` Modulo (gives the remainder of a division, e.g., `10 % 3` is `1`)
- `` Exponentiation (ES2016+, e.g., `2 3` is `8`)
- Assignment Operators: For assigning values to variables.
- `=` Assign value (e.g., `let x = 10;`)
- `+=` Add and assign (e.g., `x += 5;` is shorthand for `x = x + 5;`)
- `-=` Subtract and assign
- `*=` Multiply and assign
- `/=` Divide and assign
- Comparison Operators: For comparing values, results in `true` or `false`.
- `==` Equal value (loose equality - tries type conversion, often avoid)
- `===` Equal value AND equal type (strict equality - use this usually!)
- `!=` Not equal value (loose)
- `!==` Not equal value OR not equal type (strict inequality - use this usually!)
- `>` Greater than
- `<` Less than
- `>=` Greater than or equal to
- `<=` Less than or equal to
- Logical Operators: For combining boolean (`true`/`false`) values.
- `&&` AND ( `true` only if both sides are `true` )
- `||` OR ( `true` if at least one side is `true` )
- `!` NOT ( flips `true` to `false`, and `false` to `true` )
let score = 100;
score += 50; // score is now 150
console.log(score); // Output: 150
let price = 20;
let quantity = 3;
let total = price * quantity; // total is 60
console.log(total); // Output: 60
let age = 18;
console.log(age >= 18); // Output: true (Comparison)
console.log(age === "18"); // Output: false (Strict equality checks type)
console.log(age == "18"); // Output: true (Loose equality converts string to number - avoid!)
let hasKey = true;
let doorLocked = false;
console.log(hasKey && !doorLocked); // Output: true (Has key AND door is NOT locked)
5. Control Flow: Conditional Statements (`if`/`else`)
This section introduces conditional statements, which allow programs to make decisions and execute different blocks of code based on whether certain conditions are true or false.
Objectively, the primary conditional structures in JavaScript are `if`, `else if`, and `else`.
Delving deeper: * `if` statement: Executes a block of code only if a specified condition evaluates to `true`. Syntax: `if (condition) { // code to run if true }` * `else` statement: Executes a block of code if the preceding `if` condition evaluates to `false`. Syntax: `if (condition) { ... } else { // code to run if false }` * `else if` statement: Allows checking multiple conditions in sequence. Executes the block corresponding to the *first* `else if` condition that evaluates to `true`. An optional final `else` block runs if none of the preceding `if` or `else if` conditions were true. Syntax: `if (cond1) { ... } else if (cond2) { ... } else { ... }`
Further considerations include mentioning the `switch` statement as an alternative for handling multiple discrete cases based on a single expression's value, though `if/else if/else` is often more flexible.
Often, you want your code to do different things based on certain conditions. This is called control flow, and the most common way to achieve it is using conditional statements.
- The `if` Statement: Executes a block of code *only if* a specified condition is `true`.
let temperature = 25; // degrees Celsius if (temperature > 20) { console.log("It's a warm day!"); } // If temperature was 15, nothing would be printed.
- The `if...else` Statement: Executes one block of code if the condition is `true`, and another block if the condition is `false`.
let hour = 14; // 2 PM if (hour < 12) { console.log("Good morning!"); } else { console.log("Good afternoon/evening!"); // This line runs }
- The `if...else if...else` Statement: Checks multiple conditions in order. The code block for the *first* condition that evaluates to `true` is executed. If none are true, the final `else` block (if present) runs.
let grade = 75; if (grade >= 90) { console.log("Grade: A"); } else if (grade >= 80) { console.log("Grade: B"); } else if (grade >= 70) { console.log("Grade: C"); // This line runs } else if (grade >= 60) { console.log("Grade: D"); } else { console.log("Grade: F"); }
- *(Briefly: The `switch` statement is another way to handle multiple specific conditions based on the value of a single variable, sometimes cleaner than many `else if` checks.)*
6. Control Flow: Loops (`for`, `while`)
This section introduces loops, control structures used to execute a block of code repeatedly as long as a certain condition remains true or for a specific number of iterations.
Objectively, loops are essential for automating repetitive tasks, such as processing items in a list (array) or performing an action multiple times.
Delving deeper into common loop types: * `for` loop: Typically used when you know in advance how many times you want the loop to run. It has three parts: initialization (runs once before the loop starts), condition (checked before each iteration), and final expression (runs after each iteration, often used to increment a counter). Syntax: `for (initialization; condition; finalExpression) { // code to repeat }` * `while` loop: Executes a block of code as long as a specified condition evaluates to `true`. The condition is checked *before* each iteration. Syntax: `while (condition) { // code to repeat; // IMPORTANT: update condition variable inside }` (Need to ensure the condition eventually becomes false to avoid infinite loops).
Further considerations include briefly mentioning `do...while` loops (condition checked *after* the block, guaranteeing at least one execution) and the `break` (exit loop early) and `continue` (skip current iteration) keywords.
Loops allow you to run a block of code multiple times without writing it out repeatedly. This is incredibly useful for tasks like processing lists of items or repeating an action.
- The `for` Loop: Best when you know roughly how many times you need to repeat the code. It has three parts separated by semicolons:
- Initialization: Runs once at the very beginning (e.g., creating a counter variable `let i = 0`).
- Condition: Checked *before* each loop run. If `true`, the loop body runs; if `false`, the loop stops. (e.g., `i < 5`).
- Final Expression: Runs *after* each loop body execution (e.g., incrementing the counter `i++`).
// Print numbers 0 to 4 for (let i = 0; i < 5; i++) { console.log("The number is " + i); } // Output: // The number is 0 // The number is 1 // The number is 2 // The number is 3 // The number is 4
- The `while` Loop: Best when you want to repeat code *as long as* a certain condition is true, but you might not know exactly how many times it will run beforehand. The condition is checked *before* each iteration.
Warning: Be careful with `while` loops! If the condition never becomes false, you'll create an infinite loop that can crash your browser or program.let count = 0; while (count < 3) { console.log("Count is " + count); count++; // Crucial: Must change the condition variable inside the loop! } // Output: // Count is 0 // Count is 1 // Count is 2
- *(Briefly: A `do...while` loop is similar to `while`, but the condition is checked *after* the code block runs, so the block always executes at least once.)*
- *(Briefly: `break` exits a loop immediately. `continue` skips the rest of the current loop iteration and proceeds to the next one.)*
7. Functions: Reusable Blocks of Code
This section introduces functions – fundamental building blocks in JavaScript used to encapsulate a block of code that performs a specific task, allowing it to be reused multiple times.
Objectively, functions help organize code, make it more readable, reduce repetition (DRY - Don't Repeat Yourself), and allow for modular programming.
Delving deeper into function basics: * Declaration: Defining a function using the `function` keyword followed by a name, parentheses for parameters `()`, and curly braces `{}` for the code block. Syntax: `function functionName(parameter1, parameter2) { // code to execute; return value; }` * Parameters: Variables listed inside the parentheses in the function definition, acting as placeholders for inputs. * Arguments: The actual values passed into the function when it is called (invoked). * Calling/Invoking: Executing the code inside a function by using its name followed by parentheses `()`, potentially passing arguments. Syntax: `functionName(argument1, argument2);` * `return` Statement: Used inside a function to send a value back to the part of the code that called the function. If omitted, the function implicitly returns `undefined`.
Further considerations mention function expressions (assigning a function to a variable) and arrow functions (ES6 concise syntax, briefly introduced previously) as alternative ways to define functions. Also touch upon the concept of function scope (variables declared inside a function are typically local to it).
Functions are like named recipes or mini-programs within your main program. You define a set of instructions (code) once inside a function, give it a name, and then you can "call" (or "invoke") that function by its name whenever you need to perform that specific task, potentially providing different inputs each time.
- Why Use Functions?
- Reusability: Write the code once, use it many times.
- Organization: Break down complex problems into smaller, manageable, named pieces.
- Readability: Makes code easier to understand by giving descriptive names to blocks of functionality.
- Defining a Function (Function Declaration):
function greet(name) { // 'name' is a parameter (input placeholder) console.log("Hello, " + name + "!"); }
- Calling a Function: Use the function name followed by parentheses `()`. Pass values (arguments) inside the parentheses if the function expects parameters.
greet("Alice"); // "Alice" is the argument passed to the 'name' parameter // Output: Hello, Alice! greet("Bob"); // Output: Hello, Bob!
- Parameters and Arguments:
- Parameters: Variables listed in the function definition (`name` in the example above).
- Arguments: Actual values passed to the function when it's called (`"Alice"`, `"Bob"`).
- The `return` Statement: Functions can perform actions (like `console.log`) and also send a value back to the place where they were called using the `return` keyword.
If a function doesn't have a `return` statement, it implicitly returns `undefined`.function add(num1, num2) { let sum = num1 + num2; return sum; // Sends the calculated value back } let result = add(5, 3); // Call the function, store the returned value console.log(result); // Output: 8
- *(Briefly: Functions can also be defined as expressions (`const myFunction = function() {...};`) or using the concise ES6 arrow function syntax (`const myFunction = () => {...};`).)*
- *(Briefly: Variables declared inside a function (`let`, `const`) are generally only accessible within that function - this is called function scope.)*
8. Introduction to Objects and Arrays
This section provides a basic introduction to two fundamental data structures in JavaScript used for storing collections of data: Objects and Arrays.
Objectively: * Arrays: Ordered lists of values, enclosed in square brackets `[]`. Elements are accessed by their numerical index (starting from 0). Good for storing sequences of items. * Objects: Unordered collections of key-value pairs, enclosed in curly braces `{}`. Values are accessed by their associated key (a string). Good for representing entities with named properties.
Delving deeper into basic usage: * Array Literals: `let myArray = [value1, value2, value3];` Accessing: `myArray[0]` (gets `value1`). Checking length: `myArray.length`. Adding items: `myArray.push(newValue)`. * Object Literals: `let myObject = { key1: value1, key2: value2 };` Accessing: `myObject.key1` (dot notation) or `myObject['key1']` (bracket notation). Adding/modifying properties: `myObject.newKey = newValue;`
Further considerations mention that both arrays and objects can contain values of any data type, including other arrays or objects, allowing for complex data structures.
While primitive types (String, Number, Boolean, etc.) store single values, we often need to store collections of related data. JavaScript provides two primary ways to do this: Arrays and Objects.
- Arrays:
- Used to store an ordered list of items.
- Created using square brackets `[]`.
- Items (elements) are separated by commas.
- Elements are accessed using their index (position), starting from zero (0).
// An array of strings let colors = ["red", "green", "blue"]; console.log(colors[0]); // Access the first element -> Output: red console.log(colors[1]); // Access the second element -> Output: green console.log(colors.length); // Get the number of items -> Output: 3 colors.push("yellow"); // Add an item to the end console.log(colors); // Output: [ 'red', 'green', 'blue', 'yellow' ]
- Used to store collections of key-value pairs. Think of it like a dictionary or a real-world object with properties.
- Created using curly braces `{}`.
- Each item consists of a `key` (usually a string) followed by a colon `:` and its corresponding `value`. Key-value pairs are separated by commas.
- Values are accessed using their key (property name), either with dot notation (`.`) or bracket notation (`[]`).
// An object representing a user
let user = {
firstName: "Alice",
lastName: "Wonder",
age: 30,
isActive: true
};
console.log(user.firstName); // Access using dot notation -> Output: Alice
console.log(user['age']); // Access using bracket notation -> Output: 30
user.city = "Mirabel"; // Add a new property
console.log(user.city); // Output: Mirabel
Arrays are great for ordered lists, while objects are perfect for representing things with named characteristics. Both can hold values of any data type, including other arrays or objects!
9. Basic DOM Interaction
This section provides a very basic introduction to the Document Object Model (DOM) and how JavaScript can interact with HTML elements on a webpage.
Objectively, the DOM is a programming interface for HTML documents. It represents the page structure as a tree of objects, allowing programs (like JavaScript) to access and modify the document's content, structure, and style.
Delving deeper into basic interactions: * Selecting Elements: Using methods like `document.getElementById('elementId')` or `document.querySelector('cssSelector')` to get a reference to an HTML element in the JavaScript code. * Modifying Content: Changing the text inside an element using properties like `.textContent` or `.innerHTML`. * Handling Events: Making elements interactive by attaching event listeners, such as responding to a button click using `.onclick` or `addEventListener('click', function)`. (Keep very simple).
Further considerations emphasize that this is the foundation of how JavaScript makes webpages dynamic and interactive, manipulating the structure defined by HTML and styled by CSS.
One of the most common uses of JavaScript is to make web pages interactive. To do this, JavaScript needs a way to "see" and "change" the HTML elements on the page. This is done through the Document Object Model (DOM).
Think of the DOM as a structured representation (like a family tree) of your HTML document that JavaScript can understand and manipulate.
Here are some very basic things you can do:
- Selecting an HTML Element: You first need to grab the element you want to work with.
// Assuming you have an HTML element like:
Some text
let paragraph = document.getElementById("myParagraph"); // Now, the 'paragraph' variable holds a reference to that HTML paragraph. // Another way using CSS selectors (more flexible): let firstButton = document.querySelector("button"); // Gets the first button element - Changing Element Content: Once you have selected an element, you can change what's inside it.
// Continuing from above... if (paragraph) { // Good practice to check if element was found paragraph.textContent = "The text has been changed by JavaScript!"; // The text inside the
tag on the webpage will update. // .innerHTML can also be used, but be careful with security if using user input. }
- Responding to Events (like Clicks): You can make things happen when a user interacts with an element.
// Assuming you have: let button = document.getElementById("myButton"); if (button) { button.onclick = function() { // Assign a function to run on click alert("Button was clicked!"); }; // Alternative using addEventListener (more modern): // button.addEventListener('click', function() { // alert("Button was clicked!"); // }); }
DOM manipulation is a vast topic, but these basics show how JavaScript connects to your HTML to create dynamic experiences.
10. Conclusion & Next Steps
This concluding section recaps the fundamental JavaScript concepts covered and suggests directions for further learning.
Objectively, this guide introduced the core building blocks of JavaScript: variables, data types, operators, control flow (conditionals and loops), functions, basic objects/arrays, and a glimpse into DOM interaction.
Delving deeper, mastering these fundamentals provides a solid foundation for tackling more complex JavaScript topics and building interactive web applications. Practice is key to solidifying understanding.
Further considerations recommend exploring modern JavaScript features (ES6+), diving deeper into DOM manipulation, learning about asynchronous JavaScript (Promises, async/await), and eventually exploring frameworks/libraries (like React, Vue, Angular) or server-side JS (Node.js). Reliable resources like MDN are crucial for continued learning.
Conclusion: Your JavaScript Journey Begins!
Congratulations! You've taken your first steps into the world of JavaScript, the language that powers interactive web experiences. We've covered the essential fundamentals: how to store data with variables (`let`, `const`), the basic data types, how to perform operations and make decisions (`if`/`else`, loops), how to create reusable code blocks with functions, and how to manage collections of data with objects and arrays. We also had a brief look at how JavaScript interacts with HTML via the DOM.
This foundation is crucial. Like learning any language, practice is essential. Try modifying the examples, experimenting in your browser console, and working on small challenges.
Where to Go Next?
Deepen Your Fundamentals:
- MDN Web Docs (Mozilla): The absolute best resource. Explore their JavaScript Guide and Reference.
- javascript.info: Excellent, structured tutorials covering basics to advanced topics.
- freeCodeCamp / Codecademy: Interactive platforms to practice coding exercises.
Explore Modern JavaScript (ES6+):
- Learn about features like Arrow Functions, Destructuring, Promises, `async/await`, etc. (Check out our ES6 Features guide if available!)
Dive into the DOM:
- Learn more advanced DOM manipulation techniques for building interactive interfaces.
Consider Frameworks/Libraries or Node.js:
- Once comfortable with fundamentals, explore popular front-end tools like React, Vue, or Angular, or server-side JavaScript with Node.js.
References (Placeholder)
Links to key MDN beginner pages could be included here.
- MDN JavaScript Guide: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide
- (Placeholder for other key beginner resources)
JS Fundamentals Recap
(Placeholder: Simple graphic listing key topics: Variables, Types, Operators, Control Flow, Functions, DOM...)
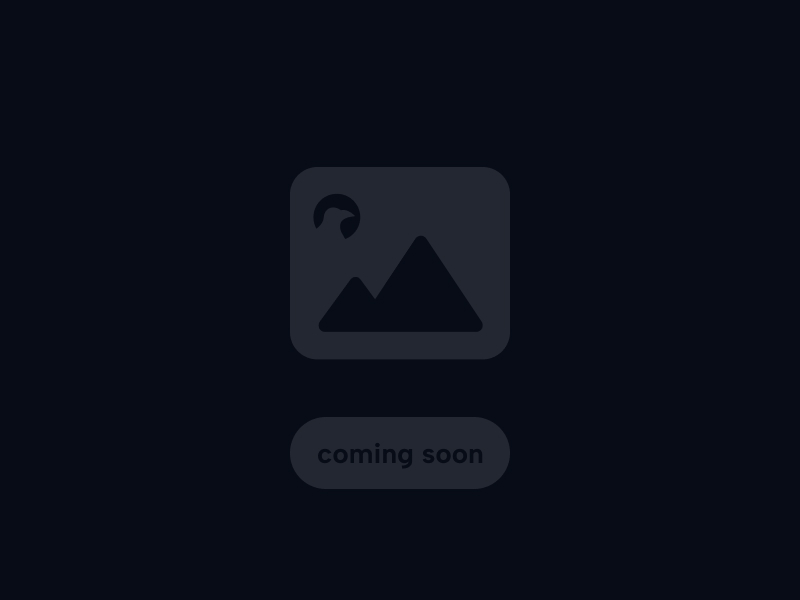