Git Version Control: Essential Skills for JavaScript Developers
Master Git, the indispensable tool for managing code changes, collaborating effectively, and maintaining sanity in modern JavaScript projects.
This overview introduces Git as a distributed version control system, crucial for tracking project history, experimenting with features via branching, and working seamlessly in development teams.
Key takeaways emphasize Git's role in preventing code loss, enabling parallel development, facilitating code reviews (Pull Requests), and managing project dependencies correctly (especially with `.gitignore`).
1. What is Version Control & Why Git?
This section defines version control and explains why Git has become the industry standard, especially relevant for JavaScript development.
Objectively, Version Control Systems (VCS) are software tools that help developers manage changes to source code over time. They track modifications, allow reverting to previous versions, and facilitate collaboration.
Delving deeper, Git is a distributed version control system (DVCS). This means every developer has a full copy of the project history locally, enabling offline work and providing redundancy. Git is known for its speed, powerful branching capabilities, and non-linear workflow support.
Further considerations highlight Git's importance for JavaScript projects (both front-end and Node.js back-end): managing complex codebases, coordinating team efforts, experimenting with new features safely (branching), integrating with CI/CD pipelines, and easily rolling back changes if bugs are introduced.
Imagine working on a JavaScript project – maybe a React app or a Node.js server. As you add features, fix bugs, or refactor code, the project evolves. What happens if you introduce a bug and need to go back to a previous working state? What if multiple developers are working on the same project simultaneously?
This is where Version Control Systems (VCS) come in. A VCS tracks every change made to your codebase, allowing you to:
- View the history of changes.
- Revert files (or the entire project) back to a previous state.
- Compare changes over time.
- Create isolated environments (branches) to work on new features without affecting the main codebase.
- Collaborate effectively with other developers by merging changes together.
Git is the most widely used Distributed Version Control System (DVCS) today. Key advantages include:
- Speed and Efficiency: Git operations are generally very fast.
- Distributed Nature: Every developer working on the project typically has a full copy (clone) of the entire repository history on their local machine. This allows for offline work and provides inherent backups.
- Powerful Branching and Merging: Git makes it easy to create branches for features or bug fixes and later merge them back into the main codebase, supporting complex workflows.
- Strong Community & Ecosystem: Vast community support, extensive documentation, and integration with popular platforms like GitHub, GitLab, and Bitbucket.
For any serious JavaScript project, whether solo or team-based, using Git is considered an essential best practice.
Version Control Concept (Conceptual)
(Placeholder: Diagram showing a timeline with different versions/snapshots of code)
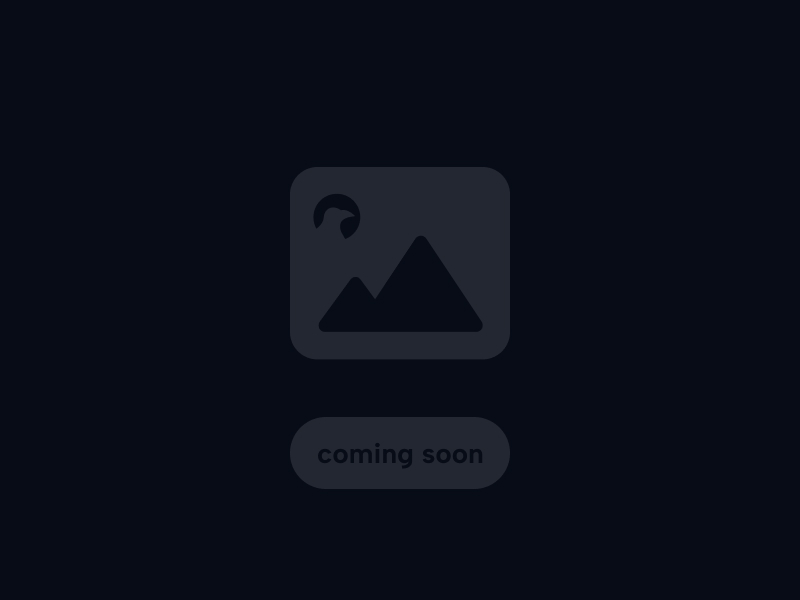
2. Setting Up Git & Basic Configuration
This section covers the initial steps of installing Git and performing essential one-time configuration.
Objectively, Git needs to be installed on your system before you can use it. Installers are available for Windows, macOS, and Linux from the official Git website.
Delving deeper: * Installation: Download from git-scm.com/downloads and follow the installation instructions for your OS. Many development environments or OS package managers (like Homebrew on macOS, apt on Debian/Ubuntu) also provide easy ways to install Git. * Verification: Open your terminal or command prompt and type `git --version` to confirm installation. * Initial Configuration (`git config`): After installation, configure your user name and email address. Git uses this information to identify who made each commit. This is typically done globally (once per machine). * `git config --global user.name "Your Name"` * `git config --global user.email "your.email@example.com"` * Other Useful Config: Setting a default text editor, enabling color output, etc. (`git config --global core.editor "code --wait"`, `git config --global color.ui auto`).
Further considerations mention checking existing configuration with `git config --list`.
Before you can start using Git, you need to install it and set up some basic configuration.
- Install Git:
- Go to the official Git website: https://git-scm.com/downloads
- Download the installer appropriate for your operating system (Windows, macOS, Linux) and follow the installation steps. Accept the default settings during installation unless you have specific reasons to change them.
- Alternatively, use a package manager if you prefer (e.g., `brew install git` on macOS, `sudo apt update && sudo apt install git` on Debian/Ubuntu).
- Verify Installation:
- Open your terminal (Git Bash on Windows, Terminal on macOS/Linux).
- Run the command:
git --version
- You should see the installed Git version printed.
- Configure Your Identity: This is a crucial one-time setup. Git embeds this information into every commit you make.
- Set your name:
git config --global user.name "Your Full Name"
- Set your email address:
(*Use the email address associated with your GitHub/GitLab account if applicable.*)git config --global user.email "your_email@example.com"
- The `--global` flag means this configuration applies to all Git repositories on your system for your user account.
- Set your name:
- Optional Recommended Configurations:
- Set your preferred text editor (e.g., VS Code):
git config --global core.editor "code --wait"
- Enable helpful color output:
git config --global color.ui auto
- Set the default branch name to `main` (modern standard):
git config --global init.defaultBranch main
- Set your preferred text editor (e.g., VS Code):
- Check Configuration: View your global settings:
git config --global --list
With Git installed and configured, you're ready to start using it in your projects.
3. Core Git Concepts: Repository, Staging Area, Commit
This section explains the fundamental building blocks and areas within a Git workflow.
Objectively, understanding these core concepts is essential for using Git effectively.
Delving deeper: * Repository (Repo): The heart of a Git project. It's a directory (often hidden, named `.git`) within your project folder that stores all the project's history, metadata, and object database (snapshots of your files). Can be local or remote. * Working Directory: Your actual project folder containing the current versions of your files that you actively modify. * Staging Area (Index): An intermediate area where you prepare changes before committing them. You selectively add (`git add`) modified files from your Working Directory to the Staging Area. This allows you to craft specific, logical commits rather than committing all changes at once. * Commit: A snapshot of the Staging Area at a particular point in time, saved permanently to the Repository's history. Each commit has a unique ID (SHA-1 hash), an author, a timestamp, and a commit message describing the changes. Commits form the project's timeline. * HEAD: A pointer that usually refers to the tip of the currently checked-out branch, indicating the commit your Working Directory is based on.
Further considerations involve visualizing the flow: Modify files (Working Directory) -> Stage changes (`git add`) -> Commit snapshot (`git commit`).
To use Git effectively, you need to understand its main components:
- Repository (Repo): This is the database where Git stores all the history and metadata for your project. When you initialize Git in a project folder (`git init`), it creates a hidden sub-directory named `.git` – this is your local repository. It contains all the commits (snapshots) and information about branches, tags, etc.
- Working Directory: This is simply your project folder – the files and directories you see and edit directly on your computer's file system. These are the current versions of your project files.
- Staging Area (Index): This is a crucial intermediate step between your Working Directory and the Repository. Before you permanently save changes (commit), you first add specific modified files from your Working Directory to the Staging Area using the `git add` command. Think of it as preparing or "staging" the exact changes you want to include in your next snapshot (commit). This allows you to create focused, meaningful commits.
- Commit: A commit is a permanent snapshot of the state of your Staging Area at a specific point in time. When you run `git commit`, Git takes everything in the Staging Area, creates a snapshot, and stores it in the Repository history. Each commit has:
- A unique identifier (an SHA-1 hash).
- Information about the author and timestamp.
- A link to its parent commit(s), forming the project history.
- A commit message explaining the changes made in that snapshot.
- HEAD: This is usually a pointer indicating which commit you are currently working on top of. Most often, `HEAD` points to the latest commit of the branch you have checked out.
The basic workflow involves modifying files in your Working Directory, selectively adding those changes to the Staging Area, and then committing the staged changes to create a new snapshot in your Repository history.
Git Workflow Areas (Conceptual Diagram)
(Placeholder: Diagram showing flow: Working Directory -> `git add` -> Staging Area -> `git commit` -> Repository (.git))
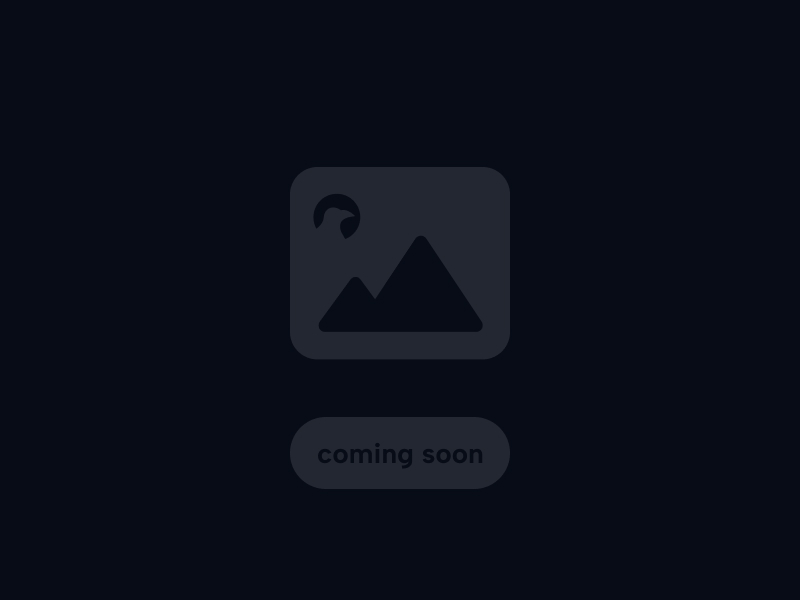
4. Essential Local Workflow Commands
This section introduces the fundamental Git commands used for managing changes within your local repository.
Objectively, these commands form the core day-to-day workflow for tracking changes in a project.
Delving deeper into essential commands:
* `git init`: Initializes a new, empty Git repository in the current directory (creates the `.git` folder). Use this once per project.
* `git status`: Shows the current state of your Working Directory and Staging Area – which files are modified, staged, or untracked. Use this frequently!
* `git add
Further considerations involve emphasizing the cycle: modify -> `git status` -> `git add` -> `git status` -> `git commit` -> repeat.
These are the commands you'll use most often when working on your project locally:
- `git init`
- Purpose: Creates a new Git repository in your current project folder. Run this only once when starting a new project with Git.
# Navigate to your project folder first cd my-javascript-project git init
- `git status`
- Purpose: Shows the status of your files – which are modified but not staged, which are staged but not committed, and which are untracked by Git. Use this command frequently!
git status
- `git add
` - Purpose: Takes the changes from your Working Directory and places them into the Staging Area, preparing them for the next commit.
- `git add file.js`: Stages changes in `file.js`.
- `git add src/`: Stages all changes within the `src` directory.
- `git add .`: Stages all modified and new files in the current directory and subdirectories (*use with caution, ensure you want to stage everything*).
# Example: Stage a specific file git add script.js # Example: Stage all changes in the current directory git add .
- `git commit -m "Commit message"`
- Purpose: Takes everything currently in the Staging Area, creates a permanent snapshot (commit) in your repository history, and attaches your message describing the changes.
- Write clear, concise, and informative commit messages (e.g., "feat: Add login component", "fix: Correct calculation error").
git commit -m "feat: Implement user authentication"
- `git log`
- Purpose: Shows the history of commits made in the repository, starting with the most recent. Displays commit hash (ID), author, date, and message.
- Useful flags: `--oneline` (concise view), `--graph` (shows branch structure), `-p` (shows changes introduced by each commit).
git log git log --oneline --graph
- `git diff`
- Purpose: Shows differences between states.
- `git diff`: Shows changes in the Working Directory not yet staged.
- `git diff --staged` (or `--cached`): Shows changes staged but not yet committed.
- `git diff
`: Shows differences between two commits.
git diff git diff --staged
The typical local cycle is: Edit files -> `git status` -> `git add` -> `git status` -> `git commit`.
5. Branching & Merging: Parallel Development
This section explains Git's powerful branching and merging capabilities, essential for managing feature development, bug fixes, and experimentation without disrupting the main codebase.
Objectively, a branch in Git is essentially a movable pointer to a specific commit. Creating a branch allows you to diverge from the main line of development and work on something in isolation.
Delving deeper:
* `git branch
Further considerations highlight that branching is fundamental for workflows like Feature Branching (develop each new feature on its own branch) common in JS projects, keeping the `main` branch stable.
Branching is one of Git's most powerful features. It allows you to create independent lines of development within your repository.
- Why Branch?
- Work on new features without affecting the stable main codebase (`main` or `master` branch).
- Fix bugs in isolation without mixing in unfinished feature code.
- Experiment with new ideas without risk to the primary project history.
- Facilitate parallel development by team members on different features.
- Creating Branches:
- `git branch
`: Creates a new branch based on your current commit, but doesn't switch to it.
git branch new-feature
- `git branch
- Switching Branches:
- `git checkout
` or (newer, preferred) `git switch `: Switches your HEAD and Working Directory to the specified branch.
git switch new-feature # Or: git checkout new-feature
- `git checkout
- Create and Switch:
- `git checkout -b
` or (newer, preferred) `git switch -c `: A shortcut to create a new branch and immediately switch to it.
git switch -c another-feature # Or: git checkout -b another-feature
- `git checkout -b
- Merging Branches: Once work on a branch is complete, you typically merge it back into your main development line (e.g., `main`).
- First, switch back to the branch you want to merge into: `git switch main`
- Then, run the merge command: `git merge
`
# Assuming work is done on 'new-feature' branch git switch main git merge new-feature
- Merge Conflicts: If changes were made to the same lines of the same file on both branches since they diverged, Git cannot automatically decide which change to keep. This results in a merge conflict. Git will mark the conflicting sections in the file(s), and you must manually edit the file(s) to resolve the conflict, then `git add` the resolved file(s) and run `git commit` to finalize the merge.
Branching is fundamental to modern development workflows, allowing for safe experimentation and organized feature development, especially crucial in collaborative JavaScript projects.
Git Branching & Merging (Conceptual Diagram)
(Placeholder: Diagram showing main line, a feature branch diverging, commits on both, then merging back)
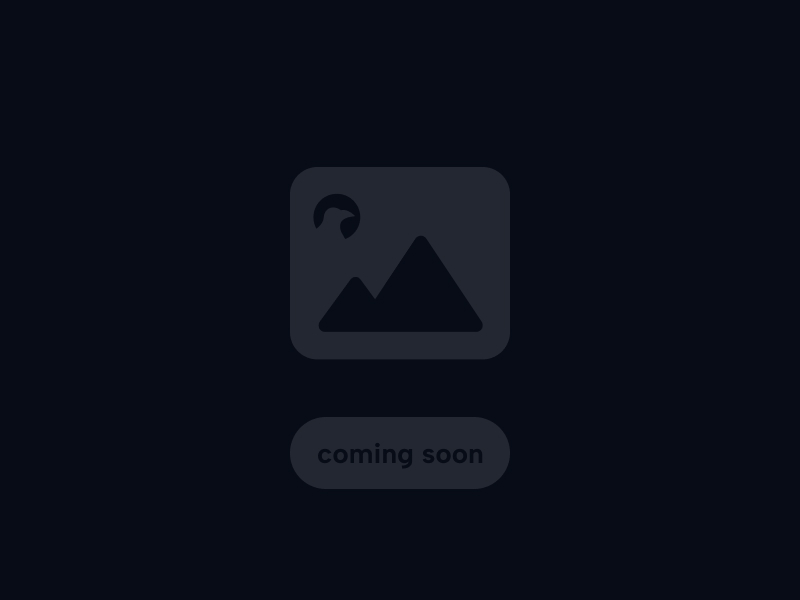
6. Working with Remotes (GitHub, GitLab, Bitbucket)
This section explains how to interact with remote Git repositories hosted on platforms like GitHub, GitLab, or Bitbucket, enabling collaboration and backup.
Objectively, a remote in Git is simply a reference to another repository, usually hosted on a server. Platforms like GitHub provide hosting for these remote repositories.
Delving deeper into key remote operations:
* `git remote add
Further considerations emphasize that remotes are essential for team collaboration, allowing developers to share code, review changes, and maintain a central project history.
Git is distributed, meaning you have a full local repository. However, to collaborate with others or back up your work, you interact with remote repositories, typically hosted on platforms like GitHub, GitLab, or Bitbucket.
- Remote: A bookmark or reference in your local repository pointing to another repository, usually on a server. The default name for the primary remote repository you clone from or push to is conventionally `origin`.
- `git clone
`: - Downloads an entire existing repository from a remote URL to your local machine, creating a new directory. It automatically sets up the `origin` remote pointing back to the source URL. This is the standard way to get started with an existing project.
git clone https://github.com/some-user/some-repo.git cd some-repo
- `git remote add
`: - Adds a new remote connection to your local repository. You give it a short name (like `origin`) and specify its URL. Usually only needed if you initialized locally (`git init`) and want to connect to a remote later.
# Example after 'git init' git remote add origin https://github.com/your-user/your-repo.git
- `git push
`: - Uploads your local commits from the specified local branch to the corresponding branch on the specified remote. This shares your work.
- Often shortened to `git push` if the upstream branch is configured (e.g., `git push -u origin main` on the first push sets this up).
# Push local 'main' branch commits to the 'origin' remote git push origin main
- `git pull
`: - Downloads changes from the specified remote branch and immediately attempts to merge them into your currently checked-out local branch. It's a combination of `git fetch` and `git merge`. Use this to get updates from collaborators.
- Often shortened to `git pull` if the upstream is configured.
# Update local 'main' branch with changes from 'origin/main' git switch main git pull origin main
- `git fetch
`: - Downloads new data (commits, branches) from the remote but does not automatically merge changes into your local working branches. It updates your remote-tracking branches (e.g., `origin/main`). This allows you to see what others have done before deciding how/when to merge.
git fetch origin # Now you can inspect changes, e.g., git log origin/main # Then merge manually if desired: git merge origin/main
Working with remotes is fundamental for collaboration and backing up your code history.
7. Pull Requests / Merge Requests: Collaboration Workflow
This section explains the concept of Pull Requests (common on GitHub/Bitbucket) or Merge Requests (GitLab terminology), a core mechanism for collaborative code review and integration.
Objectively, a Pull Request (PR) or Merge Request (MR) is not a core Git feature itself, but rather a feature provided by hosting platforms built *around* Git. It's a formal proposal to merge changes from one branch (e.g., a feature branch) into another (e.g., the `main` branch).
Delving deeper into the PR/MR workflow: 1. A developer works on a feature or fix in a separate branch (`feature-branch`). 2. They push this branch to the remote repository (`git push origin feature-branch`). 3. On the hosting platform (GitHub/GitLab), they initiate a PR/MR, targeting the `main` branch as the destination. 4. This creates a dedicated space for discussion and code review. Other team members can comment on the code, suggest changes, and discuss the implementation. 5. Automated checks (like tests or linters via CI/CD pipelines) can run against the proposed changes. 6. Once approved (and conflicts resolved, if any), a project maintainer merges the PR/MR, incorporating the changes from the feature branch into the target branch (`main`).
Further considerations highlight how PRs/MRs promote code quality, knowledge sharing, and controlled integration of changes, making them standard practice in team-based JavaScript development.
While `git merge` handles combining branches locally or directly on a remote, Pull Requests (PRs) on GitHub/Bitbucket or Merge Requests (MRs) on GitLab provide a structured workflow for proposing, reviewing, and merging code changes, especially in collaborative environments.
The typical PR/MR workflow looks like this:
- Create a Feature Branch: Create a new branch locally for the feature or bug fix you're working on (`git switch -c my-feature`).
- Make Changes & Commit: Write your code and make one or more commits on your feature branch (`git add .`, `git commit -m "..."`).
- Push the Branch: Push your feature branch to the remote repository (`git push origin my-feature`).
- Open a Pull/Merge Request: Go to the hosting platform (GitHub, GitLab, etc.). You'll usually see a prompt to create a PR/MR for your recently pushed branch. Create the PR/MR, specifying your feature branch as the source and the branch you want to merge into (e.g., `main`) as the target.
- Code Review & Discussion: The PR/MR provides a dedicated page where:
- Team members can review the code changes (diffs).
- Comments and suggestions can be made inline.
- Discussions about the implementation can take place.
- Automated checks (Continuous Integration tests, linters) can run and report status.
- Address Feedback: If reviewers request changes, make further commits on your local feature branch and push them again. The PR/MR automatically updates.
- Approval & Merging: Once the changes are approved and pass any required checks, a maintainer (or sometimes the author) merges the PR/MR via the platform's interface. This integrates the feature branch commits into the target branch (`main`).
- Clean Up (Optional): After merging, the feature branch can often be deleted from the remote and locally.
This workflow is standard practice in open-source and professional JavaScript development, promoting code quality, collaboration, and a traceable history of changes.
Pull Request / Merge Request Workflow (Conceptual)
(Placeholder: Flowchart: Branch -> Push -> Create PR/MR -> Review -> Approve -> Merge -> Main)
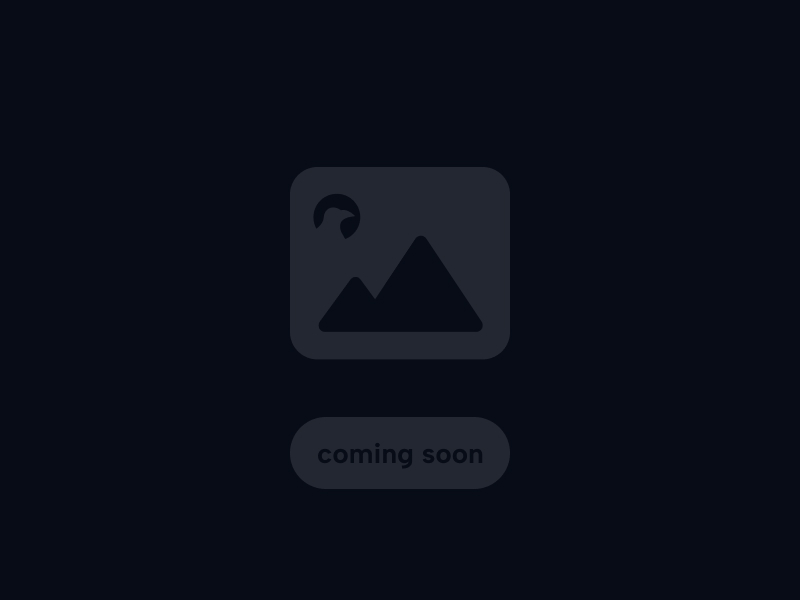
8. `.gitignore` for JavaScript Projects
This section explains the purpose and importance of the `.gitignore` file, particularly for typical JavaScript/Node.js projects.
Objectively, a `.gitignore` file is a plain text file placed in the root of a Git repository that tells Git which files or directories it should intentionally ignore and not track.
Delving deeper, ignored files won't show up in `git status` as untracked, and `git add .` will not stage them. This is crucial for keeping the repository clean and avoiding committing unnecessary or sensitive files.
Further considerations highlight common entries for JavaScript projects: * `node_modules/`: Essential! This directory contains downloaded dependencies and can be huge; it should always be ignored as dependencies can be reinstalled using `npm install` based on `package.json` / `package-lock.json`. * Build Output: Directories containing bundled/transpiled code (e.g., `dist/`, `build/`, `public/`). * Log Files: (`*.log`). * Environment Variables: Files containing sensitive configuration or API keys (e.g., `.env`). * IDE/Editor Configuration: Specific configuration files or directories generated by editors (e.g., `.vscode/`, `.idea/`). * OS-Specific Files: System files like `.DS_Store` (macOS) or `Thumbs.db` (Windows).
Using a well-maintained `.gitignore` tailored for Node.js/JavaScript is a fundamental best practice.
When working with Git, you often have files or directories in your project folder that you *don't* want to track or commit to the repository history. These might include:
- Dependencies downloaded by package managers (like the huge `node_modules` folder).
- Compiled or bundled code output (e.g., `dist`, `build` folders).
- Log files (`.log`).
- Operating system files (`.DS_Store`, `Thumbs.db`).
- IDE or editor configuration files (`.vscode/`, `.idea/`).
- Files containing sensitive information like API keys or passwords (`.env`).
To tell Git to ignore these, you create a file named exactly `.gitignore` (note the leading dot) in the root directory of your repository. Each line in this file specifies a pattern for files or directories to ignore.
Why is this crucial for JavaScript projects?
- `node_modules/`: This directory contains all third-party packages installed via NPM. It can be very large and contains hundreds or thousands of files. Committing it bloats the repository unnecessarily. Anyone cloning the repo can simply run `npm install` to regenerate this folder based on `package.json` and `package-lock.json`. Always ignore `node_modules/`.
- Build Artifacts: Front-end projects often have build steps that generate optimized code in folders like `dist` or `build`. This generated code shouldn't typically be version controlled; only the source code should.
- Security: Accidentally committing files like `.env` containing sensitive credentials is a major security risk.
Example `.gitignore` for a typical Node.js / Front-end JS project:
# Dependencies
node_modules/
npm-debug.log*
yarn-error.log*
# Build output
build/
dist/
public/ # If generated by build process
# Logs
logs/
*.log
# Environment variables
.env
.env.*
!.env.example # Exclude example env file if needed
# OS generated files
.DS_Store
Thumbs.db
# IDE / Editor directories
.idea/
.vscode/
*.suo
*.ntvs*
*.njsproj
*.sln
*.sw? # Vim swap files
# Test output
coverage/
You can find standard `.gitignore` templates for Node.js and various frameworks online (e.g., gitignore.io or GitHub's templates).
9. Common Git Workflows & Best Practices
This section introduces common strategies (workflows) for using Git branches effectively in development, along with general best practices.
Objectively, having a defined workflow helps teams manage development, releases, and fixes in an organized manner.
Delving deeper into common workflows: * Feature Branch Workflow: A simple and popular model. Developers create a new branch for each feature or bug fix off the main branch (`main`). Work is done on the feature branch, and once complete and reviewed (via PR/MR), it's merged back into `main`. `main` always represents stable, production-ready code. * Gitflow Workflow: A more complex workflow involving multiple long-running branches (`main` for production releases, `develop` for integration) and supporting branches (feature, release, hotfix). Suitable for projects with scheduled releases but can be overkill for simpler projects or continuous deployment models. * GitHub Flow / GitLab Flow: Variations often simplifying Gitflow, typically using a single `main` branch and feature branches with deployment directly from `main` or specific release branches/tags.
Further considerations cover best practices: * Write clear, concise, and descriptive commit messages (follow conventions like Conventional Commits if possible). * Commit small, logical changes frequently. * Pull changes from the remote often (`git pull` or `git fetch`/`git merge`) to stay updated and minimize merge conflicts. * Use branches extensively for new work. * Review code via Pull/Merge Requests.
While you can use Git branches however you like, adopting a standard workflow helps keep development organized, especially in teams.
Common Workflows:
- Feature Branch Workflow (Popular & Simple):
- The `main` branch always contains production-ready code.
- To work on a new feature or bug fix, create a new branch *from* `main` (e.g., `git switch -c feature/user-login`).
- Do all your work and commits on this feature branch.
- When the feature is complete, open a Pull Request (PR) / Merge Request (MR) to merge your branch back into `main`.
- After review and approval, merge the PR/MR into `main`.
- Optionally delete the feature branch.
- Gitflow Workflow (More Complex):
- Uses two main long-lived branches: `main` (for tagged production releases) and `develop` (for integrating completed features).
- Uses supporting branches: `feature/*` (branched from `develop`), `release/*` (for preparing releases, branched from `develop`), `hotfix/*` (for urgent production fixes, branched from `main`).
- Can be overly complex for many projects, especially those using continuous delivery.
- GitHub Flow / GitLab Flow: Simpler variations often focusing on feature branches merged directly into `main` (GitHub Flow) or incorporating environment/release branches (GitLab Flow).
General Best Practices:
- Commit Frequently: Make small, logical commits often. It's easier to understand history and revert changes if needed.
- Write Good Commit Messages: Use the imperative mood (e.g., "Fix login bug" not "Fixed login bug"). Explain *what* the change does and *why*. Consider conventions like Conventional Commits.
- Pull Often: Regularly update your local branches (`git pull` or `Workspace`/`merge`) with changes from the remote to avoid large merge conflicts later.
- Branch Liberally: Don't hesitate to create branches even for small changes. It keeps your main line clean.
- Use Pull/Merge Requests: Leverage code reviews for better quality and knowledge sharing.
- Use `.gitignore`: Keep your repository clean from unnecessary files.
Feature Branch Workflow (Conceptual Diagram)
(Placeholder: Diagram showing Main branch, Feature branch off Main, commits, PR/Merge back to Main)
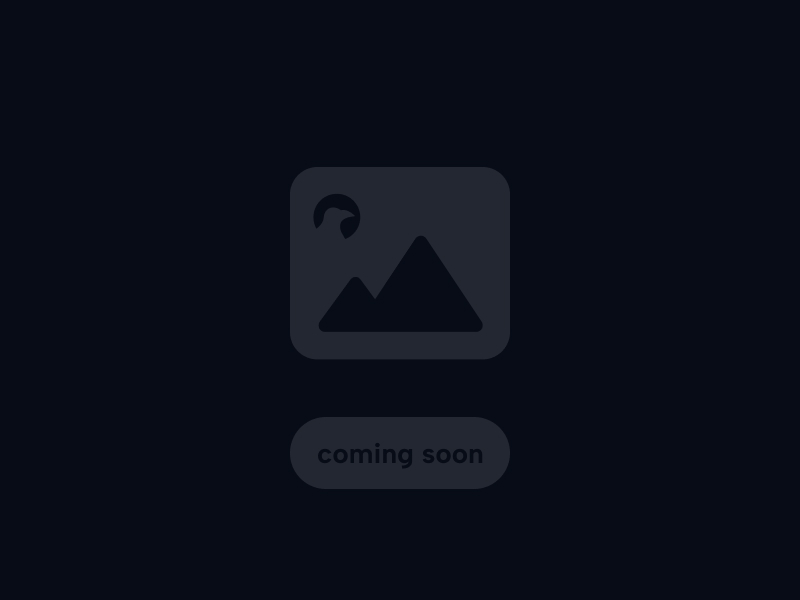
10. Conclusion & Resources
This concluding section summarizes the importance of Git for JavaScript developers and provides resources for continued learning.
Objectively, Git is an essential tool in the modern software development landscape, providing robust version control critical for managing JavaScript projects of any size, both individually and collaboratively.
Delving deeper, mastering the core concepts (repo, staging, commit), essential commands (`add`, `commit`, `status`, `log`), branching/merging, and remote interactions (push, pull, clone, PRs/MRs) empowers developers to work efficiently and safely.
Further considerations emphasize that Git proficiency comes with practice. Developers are encouraged to use Git consistently, explore different workflows, and consult official documentation and community resources to deepen their skills.
Conclusion: Git - Your Safety Net and Collaboration Hub
For JavaScript developers today, Git isn't just a tool – it's a fundamental part of the workflow. It acts as a safety net, allowing you to track every change, revert mistakes, and experiment without fear. It's also the backbone of collaboration, enabling teams to work together on complex codebases efficiently using features like branching and Pull/Merge Requests.
Understanding the core concepts and mastering the essential commands covered in this guide will significantly boost your productivity and confidence as a developer. Remember to use `.gitignore` effectively, adopt a consistent workflow, write meaningful commit messages, and leverage remote repositories for backup and collaboration. Practice is key – use Git in all your projects, big or small!
Git Learning Resources
Official & Foundational:
- Git Official Website & Documentation: git-scm.com (Reference, Download)
- Pro Git Book (Free Online): git-scm.com/book/en/v2 (Comprehensive Guide)
Hosting Platforms & Guides:
- GitHub Guides: guides.github.com
- GitLab Docs: docs.gitlab.com
- Bitbucket Git Tutorials (by Atlassian): atlassian.com/git/tutorials
Interactive Learning:
- Learn Git Branching: learngitbranching.js.org (Visual and interactive)
- Codecademy / Udacity / Coursera / Udemy often have Git courses.
Tools & Utilities:
- gitignore.io / topal.com/developers/gitignore: Generate `.gitignore` files easily.
- Git GUIs (SourceTree, GitKraken, GitHub Desktop): Visual interfaces for Git (though learning commands is recommended).
References (Placeholder)
Links to specific sections of Pro Git or Git command documentation could go here.
- Pro Git, Chapter 1: Getting Started
- Pro Git, Chapter 2: Git Basics
- Pro Git, Chapter 3: Git Branching
- Git Command Reference
Git Essentials Overview
(Placeholder: Simple graphic listing key topics: VCS, Basics, Branch/Merge, Remote, PRs, .gitignore)
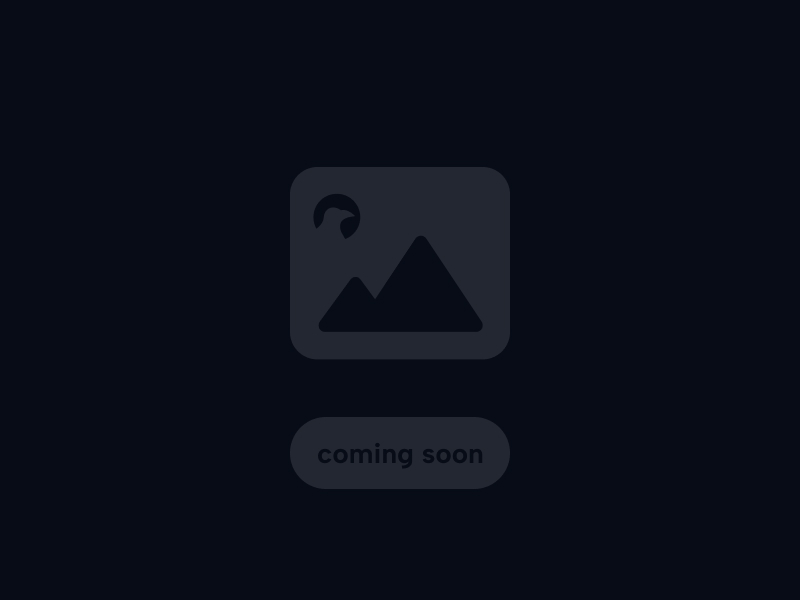