Choosing Your Front-End Weapon: React, Vue, Angular, Svelte & More
Navigate the vibrant world of modern JavaScript front-end frameworks. Understand the key players and core concepts for building dynamic and efficient user interfaces.
This guide provides an overview of leading frameworks like React, Vue.js, Angular, and Svelte, exploring their architectures (components, state management, rendering), ecosystems, and trade-offs.
Key takeaways focus on understanding component-based development, reactivity, and how these frameworks help manage complexity and improve developer productivity in building sophisticated web applications.
1. What Are Front-End Frameworks & Why Use Them?
This section defines front-end frameworks in the context of JavaScript web development and explains the problems they solve compared to using vanilla JavaScript or older libraries.
Objectively, a front-end framework provides a foundational structure, pre-written code components, and established patterns for building user interfaces (UIs) for web applications. They primarily use JavaScript, along with HTML and CSS.
Delving deeper, as web applications became more complex than static pages, managing UI state, handling user interactions, updating the view efficiently, and organizing code became challenging with just vanilla JS or libraries like jQuery. Frameworks emerged to address these challenges.
Further considerations highlight the key benefits:
- Structure & Organization: Enforce conventions and patterns (like component-based architecture) for building maintainable codebases.
- Efficiency & Productivity: Provide reusable components, utility functions, and abstractions that speed up development.
- Maintainability: Structured code is easier to understand, debug, and update over time, especially in teams.
- Performance: Many frameworks employ techniques like Virtual DOM or compile-time optimizations to efficiently update the UI in response to data changes.
- Community & Ecosystem: Popular frameworks have large communities, extensive documentation, and rich ecosystems of supporting libraries and tools.
While you *can* build web UIs with just HTML, CSS, and vanilla JavaScript, it quickly becomes complex and difficult to manage as applications grow. Front-end frameworks (like React, Vue, Angular, Svelte) provide a structured approach and powerful tools to simplify this process.
Think of a framework as a scaffold or blueprint for building your user interface. It offers:
- Structure and Conventions: Guidelines and patterns on how to organize your code, making it more predictable and maintainable, especially when working in teams.
- Reusable Components: Encourages breaking down the UI into smaller, self-contained, reusable pieces (Components), making development faster and code cleaner.
- State Management: Tools and patterns for managing the data (state) of your application and automatically updating the UI when that data changes (Reactivity).
- Efficient Rendering: Techniques (like the Virtual DOM in React/Vue or compile-time updates in Svelte) to update only the necessary parts of the webpage when data changes, improving performance.
- Routing: Built-in or companion libraries to handle navigation between different "pages" or views within a Single Page Application (SPA).
- Ecosystem & Community: Access to a vast collection of third-party libraries, tools, tutorials, and community support.
Using a framework significantly boosts productivity, promotes best practices, and helps manage the complexity inherent in building modern, interactive web applications.
Framework Benefits (Conceptual)
(Placeholder: Graphic showing Structure, Efficiency, Components, Performance icons)
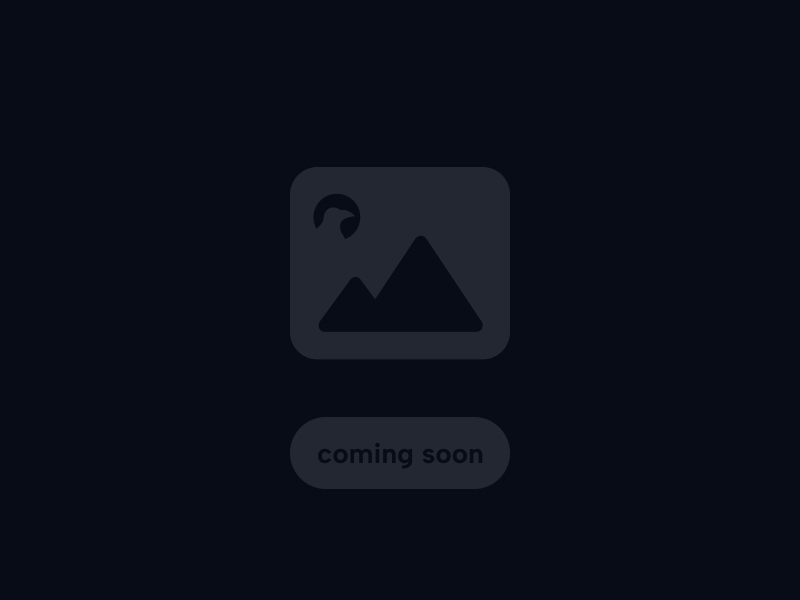
2. Core Concepts Common to Front-End Frameworks
This section explains fundamental concepts shared by most modern JavaScript front-end frameworks, forming the basis of how they work.
Objectively, while syntax and implementation details differ, concepts like component-based architecture, state management, and reactivity are central to frameworks like React, Vue, and Angular.
Delving deeper into core concepts:
- Component-Based Architecture: Breaking the UI down into small, independent, reusable pieces called components (e.g., a button, a user profile card, a navigation bar). Each component manages its own logic and presentation. Components can be nested to build complex UIs.
- State: The data that describes the current condition of a component or application (e.g., user input, fetched data, UI status like 'loading').
- Reactivity: The mechanism by which the framework automatically updates the UI (the DOM) whenever the underlying state changes. This eliminates manual DOM manipulation for data updates.
- Props (Properties): How data is passed *down* from a parent component to a child component. Props are typically read-only within the child.
- Templating / Rendering: How the framework defines the structure of components (using HTML-like syntax like JSX in React, templates in Vue/Angular) and renders them to the actual DOM. Some use a Virtual DOM (an in-memory representation) to efficiently calculate and apply updates (React, Vue), while others compile templates directly to imperative DOM updates (Svelte).
- Routing: Managing navigation between different views or "pages" within a Single Page Application (SPA) without requiring full page reloads. Often handled by companion libraries.
Further considerations mention that understanding these core ideas makes it easier to learn and compare different frameworks.
While each framework has its unique syntax and features, most modern JavaScript front-end frameworks share several core concepts:
- Component-Based Architecture: This is fundamental. The UI is built by combining small, independent, and reusable pieces called Components. Think of LEGO bricks – each component (like a button, header, form input, user list) has its own HTML structure, CSS styling, and JavaScript logic. You compose these components together to create complex interfaces.
- State: Represents the data that drives your application's UI at any given moment. It could be user input in a form, data fetched from an API, whether a modal is open, etc. When the state changes, the UI should ideally update to reflect that change.
- Reactivity: The "magic" that links state changes to UI updates. Frameworks provide mechanisms so that when you update a piece of state data, the parts of the UI that depend on that data automatically re-render to show the new information, without you needing to manually manipulate the DOM.
- Props (Properties): A way for parent components to pass data *down* to their child components. This allows for configuring and customizing reusable components. Props are generally treated as immutable (read-only) by the child component.
- Templating / Rendering: How you define what a component looks like (its HTML structure) and how the framework translates that definition into actual elements on the webpage (DOM manipulation).
- Some use JSX (React) - an HTML-like syntax extension within JavaScript.
- Others use HTML-based template syntax with special directives (Vue, Angular).
- Some frameworks use a Virtual DOM (React, Vue) as an intermediate step to efficiently calculate the minimum required changes to the actual DOM. Others, like Svelte, compile components into highly optimized vanilla JavaScript that updates the DOM directly.
- Routing: In Single Page Applications (SPAs), routing allows users to navigate between different views or sections of the application without triggering a full page refresh from the server. This is usually handled by dedicated routing libraries associated with the framework (e.g., React Router, Vue Router, Angular Router).
Grasping these concepts provides a solid foundation for understanding how different frameworks approach building user interfaces.
Component Tree Example (Conceptual)
(Placeholder: Simple tree diagram showing App component branching into Header, Main, Footer, etc.)
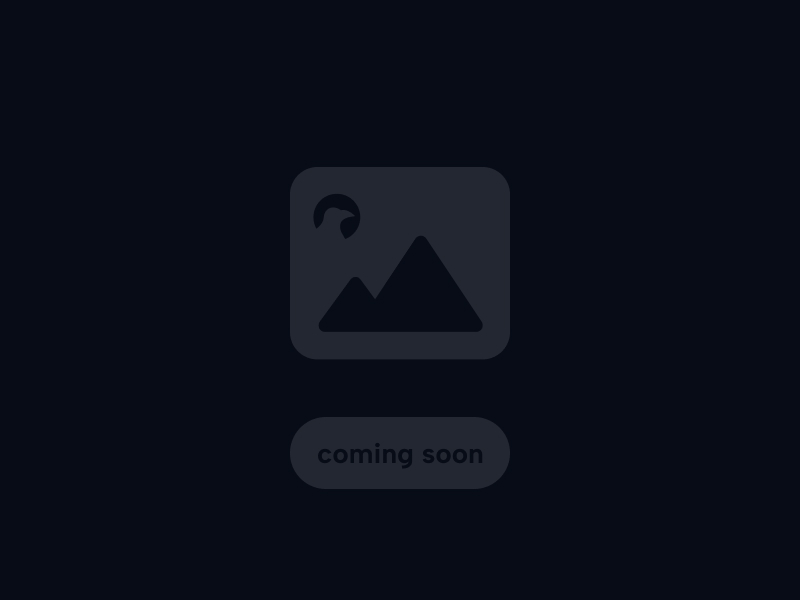
(Source: UI Architecture Concept)
3. React.js Overview
This section provides a high-level overview of React.js, one of the most popular front-end libraries (often referred to as a framework) for building user interfaces.
Objectively, React is developed and maintained by Meta (Facebook) and has a vast community and ecosystem. It focuses primarily on the "View" layer of an application.
Delving deeper into key features:
- Component-Based: Encourages building UIs from reusable components (functional components with Hooks, or older class components).
- JSX (JavaScript XML): A syntax extension allowing you to write HTML-like structures directly within your JavaScript code, making component rendering intuitive.
- Virtual DOM: Uses an in-memory representation of the UI to efficiently determine and apply only the necessary changes to the actual browser DOM, improving performance.
- Hooks (e.g., `useState`, `useEffect`): Introduced in React 16.8, allowing functional components to manage state and lifecycle features previously only available in class components.
- Unidirectional Data Flow: Data typically flows down from parent to child components via props, making state management more predictable.
Further considerations mention React's flexibility (often requiring additional libraries for routing or complex state management like Redux or Zustand), its large ecosystem (including frameworks like Next.js for server-side rendering/static site generation), and its significant presence in the job market.
React (often called React.js or ReactJS) is a JavaScript library (though often used like a framework) developed by Meta (Facebook) for building user interfaces, particularly Single Page Applications.
- Core Focus: Primarily concerned with the View layer – efficiently rendering UI components and updating them when data changes.
- Component Model: Everything is a component. UIs are built by composing small, reusable functional components (using Hooks) or class components (less common in new code).
- JSX: Uses JSX, an XML-like syntax extension that lets you write HTML structures within your JavaScript code. While optional, it's the standard way to define component markup in React.
// Simple React Functional Component with JSX function Welcome(props) { return <h1>Hello, {props.name}</h1>; }
- Virtual DOM: React maintains a lightweight representation of the actual DOM in memory (the Virtual DOM). When state changes, React updates the Virtual DOM first, compares it with the previous version ("diffing"), and then efficiently updates only the changed parts of the real browser DOM.
- Hooks (`useState`, `useEffect`, etc.): Modern React development heavily relies on Hooks, which are functions that let you "hook into" React state and lifecycle features from functional components. `useState` manages component state, `useEffect` handles side effects (like data fetching).
- Unidirectional Data Flow: Data generally flows down from parent components to child components via `props`. Managing state that needs to be shared across many components often requires state management libraries or React's Context API.
- Ecosystem: React itself is relatively focused. Functionality like routing (`React Router`) or advanced state management (`Redux`, `Zustand`, `Jotai`) are handled by separate, popular libraries. Frameworks like Next.js (for server-side rendering, static sites) and Remix are built on top of React.
Pros: Large community and ecosystem, high performance (Virtual DOM), component reusability, widely used in industry (good job market).
Cons: Can require choosing and learning additional libraries for a complete solution, JSX has a slight learning curve for beginners.
4. Vue.js Overview
This section provides a high-level overview of Vue.js, another popular progressive JavaScript framework for building user interfaces.
Objectively, Vue was created by Evan You and is known for its approachability, clear documentation, and gentle learning curve, making it popular among beginners and experienced developers alike.
Delving deeper into key features:
- Progressive Framework: Can be adopted incrementally – use it for small parts of an existing application or build complex SPAs from scratch.
- Component-Based: Uses components as building blocks, similar to React and Angular.
- Approachable Template Syntax: Uses HTML-based templates with directives (like `v-bind`, `v-on`, `v-for`, `v-if`) to declaratively bind data to the DOM, often considered easier to grasp initially than JSX.
- Reactivity System: Automatically tracks dependencies and updates the DOM efficiently when underlying JavaScript data changes. Uses a Virtual DOM similar to React (in Vue 2 & 3).
- Single File Components (.vue files): Encourages organizing a component's template, script (logic), and styles within a single `.vue` file.
Further considerations mention Vue's excellent documentation, its well-integrated ecosystem for routing (`Vue Router`) and state management (`Pinia` - recommended, or older `Vuex`), and frameworks built on it like Nuxt.js (similar to Next.js for React).
Vue.js is an approachable, progressive JavaScript framework for building user interfaces. Created by Evan You, it aims to be adoptable incrementally.
- Progressive Nature: You can start using Vue for just a small part of your UI or scale it up to build sophisticated Single Page Applications.
- Component Model: Also based on reusable components. Components can be defined using an Options API or the newer Composition API (more flexible for complex components).
- HTML-based Templates: Vue uses an intuitive HTML-based template syntax with special attributes called directives (prefixed with `v-`) to bind data, handle events, loop through lists, and conditionally render elements. This often feels familiar to developers comfortable with HTML.
<!-- Simple Vue Template Example --> <div id="app"> <p>{{ message }}</p> <input v-model="message"> <!-- Two-way data binding --> <button @click="reverseMessage">Reverse Message</button> </div> <script> // Corresponding Vue instance logic (simplified) const { createApp } = Vue; createApp({ data() { return { message: 'Hello Vue!' } }, methods: { reverseMessage() { this.message = this.message.split('').reverse().join(''); } } }).mount('#app'); </script>
- Reactivity: Vue's core feature is its highly optimized reactivity system. When you modify data properties, Vue automatically detects the change and efficiently updates the relevant parts of the DOM.
- Single File Components (.vue): A common pattern where a component's template (``), logic (`