ES6 (ECMAScript 2015): Level Up Your JavaScript
A practical guide to the essential features introduced in ES6 that revolutionized modern JavaScript development, making code cleaner, more powerful, and easier to write.
This overview summarizes key additions like `let`/`const`, arrow functions, template literals, destructuring, classes, modules, and Promises, which are fundamental for contemporary JS projects.
Key takeaways highlight how adopting ES6 features improves code readability, maintainability, and developer productivity, enabling more robust and efficient applications.
1. What is ES6 (ECMAScript 2015) & Why Is It Important?
This section introduces ECMAScript 2015, commonly known as ES6, and explains its significance in the evolution of the JavaScript language.
Objectively, ES6 was a major update to the ECMAScript standard (the specification JavaScript is based on), finalized in June 2015. It introduced a wealth of new syntax and features designed to address common pain points and make JavaScript development more efficient and powerful.
Delving deeper, prior to ES6, JavaScript development often relied on workarounds for things like proper variable scoping (using `var`), defining classes, handling asynchronous operations cleanly, and managing code modules. ES6 provided native solutions for these and more.
Further considerations highlight that ES6 features are now widely supported by modern browsers and Node.js environments, making them essential knowledge for any contemporary JavaScript developer. Mastering ES6 allows for writing more readable, concise, and maintainable code.
ECMAScript is the official standard upon which JavaScript is based. ES6 (ECMAScript 2015) represents the 6th major edition of this standard, released in 2015. It marked a significant leap forward for the JavaScript language, introducing fundamental improvements and new capabilities that have become standard practice in modern web development.
Before ES6, JavaScript developers faced challenges with:
- Variable scoping and hoisting issues (primarily with `var`).
- Cumbersome syntax for function expressions, especially callbacks.
- Complex string manipulation and concatenation.
- Lack of native class syntax for object-oriented patterns.
- No built-in module system for organizing code.
- Difficult management of asynchronous operations (often leading to "callback hell").
ES6 addressed these issues and more, introducing features that enhance productivity, readability, and the overall power of the language. Understanding ES6 is crucial for writing modern, efficient, and maintainable JavaScript code used in front-end frameworks (React, Vue, Angular), Node.js back-ends, and general web development.
JavaScript Evolution: Pre-ES6 vs. Modern JS (Conceptual)
(Placeholder: Simple graphic showing a timeline or split screen contrasting older JS practices with ES6+ benefits)
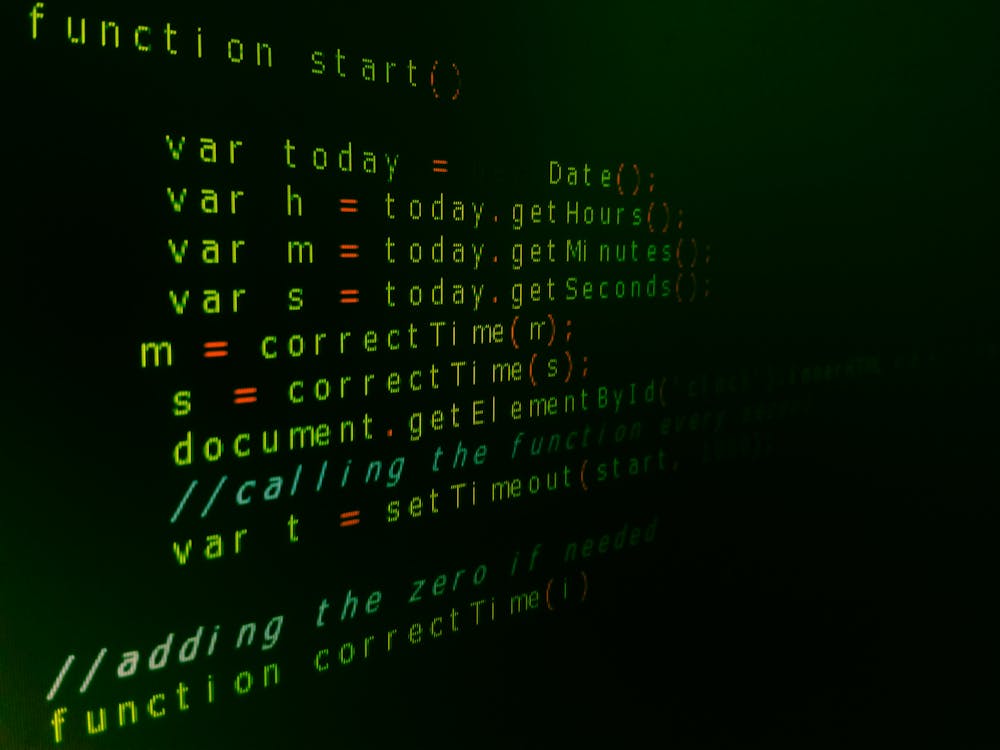
2. Block-Scoped Variables: `let` and `const`
This section introduces two new ways to declare variables in ES6, `let` and `const`, explaining how they improve upon the older `var` keyword.
Objectively, `let` and `const` provide block scoping, meaning the variable is confined to the block (e.g., inside `{...}` like in an `if` statement or `for` loop) where it is defined. This contrasts with `var`, which has function scope or global scope, often leading to unexpected behavior and bugs.
Delving deeper: * `let`: Declares a block-scoped variable whose value *can* be reassigned later. * `const`: Declares a block-scoped variable whose value *cannot* be reassigned after initialization (it's constant). For objects and arrays declared with `const`, the variable itself cannot be reassigned, but the *contents* of the object or array can still be modified.
Further considerations emphasize that using `let` and `const` instead of `var` is now standard practice as it leads to clearer, more predictable code by limiting variable scope and preventing accidental reassignments (with `const`).
One of the most fundamental improvements in ES6 was the introduction of `let` and `const` for variable declarations, largely replacing the older `var` keyword.
- `var` (The Old Way): Variables declared with `var` are function-scoped or globally-scoped. They are also "hoisted" (declarations moved to the top), which could lead to confusing behavior.
- `let` (The New `var`):
- Declares variables that are block-scoped. This means the variable only exists within the nearest set of curly braces `{}` (like inside an `if` block, `for` loop, or just a standalone block).
- Variables declared with `let` can be reassigned later.
- `const` (Constants):
- Also block-scoped.
- Declares variables whose value cannot be reassigned after they are initialized. This helps prevent accidental changes to variables that should remain constant.
- Important: For objects and arrays declared with `const`, the variable itself cannot be reassigned to a different object/array, but the properties of the object or elements of the array *can* still be modified.
Modern JavaScript best practice generally recommends using `const` by default and only using `let` when you know a variable's value needs to change. Avoid using `var` in new code.
// Example: Block Scope with let
if (true) {
let blockVar = "I'm inside";
console.log(blockVar); // Output: I'm inside
}
// console.log(blockVar); // Error: blockVar is not defined outside the block
// Example: const cannot be reassigned
const PI = 3.14159;
// PI = 3.14; // Error: Assignment to constant variable.
// Example: const object properties can be modified
const myObj = { name: "Qwirey" };
myObj.name = "Qwirey Inc."; // This is allowed
console.log(myObj); // Output: { name: 'Qwirey Inc.' }
// myObj = {}; // Error: Assignment to constant variable.
3. Arrow Functions (`=>`)
This section introduces arrow functions, a concise syntax for writing function expressions in ES6, and highlights their key characteristics.
Objectively, arrow functions provide a shorter syntax compared to traditional `function` expressions, especially for simple, single-expression functions.
Delving deeper: * Syntax: `(param1, param2) => { statements }` or `param => expression` (implicit return for single expression). * Conciseness: Particularly useful for callbacks and inline functions. * Lexical `this` Binding: This is a crucial difference. Arrow functions do not have their own `this` context. Instead, they inherit `this` from the surrounding (lexical) scope where they were defined. This avoids common `this` binding issues found with traditional functions, especially within methods or callbacks.
Further considerations include noting that arrow functions are not suitable for all situations (e.g., they cannot be used as constructors, lack their own `arguments` object).
Arrow functions provide a more concise syntax for writing functions in JavaScript, especially useful for anonymous functions and callbacks.
- Concise Syntax:
- Traditional function expression: `const add = function(a, b) { return a + b; };`
- Arrow function equivalent: `const add = (a, b) => { return a + b; };`
- If the function body is a single expression, the `{}` and `return` can be omitted (implicit return): `const add = (a, b) => a + b;`
- If there's only one parameter, the parentheses `()` around it can be omitted: `const square = x => x * x;`
- Lexical `this`: This is the most significant difference from traditional functions. Arrow functions do not bind their own `this` value. Instead, `this` inside an arrow function always refers to the `this` value of the enclosing lexical scope (the scope where the arrow function was defined).
- This solves many common problems where traditional functions lose the intended `this` context (e.g., inside event handlers or `setTimeout` callbacks within object methods).
- Other Differences: Arrow functions cannot be used as constructors (with `new`), do not have their own `arguments` object (use rest parameters instead), and cannot be used as generator functions.
// Traditional function
const numbers = [1, 2, 3];
const squaresTrad = numbers.map(function(n) {
return n * n;
});
console.log(squaresTrad); // Output: [ 1, 4, 9 ]
// Arrow function (concise)
const squaresArrow = numbers.map(n => n * n);
console.log(squaresArrow); // Output: [ 1, 4, 9 ]
// Lexical 'this' example
function Counter() {
this.count = 0;
setInterval(() => {
// 'this' here correctly refers to the Counter instance
this.count++;
console.log(this.count);
}, 1000);
}
// const myCounter = new Counter(); // Uncomment to run
// Without arrow function, 'this' inside setInterval would typically be window/global or undefined.
4. Template Literals (Template Strings)
This section introduces template literals, an enhanced way to work with strings in ES6, offering easier interpolation and multi-line capabilities.
Objectively, template literals are enclosed by backticks (`` ` ``) instead of single ('') or double ("") quotes, enabling embedded expressions and multi-line strings without special characters.
Delving deeper: * String Interpolation: Embedded expressions (variables, function calls) can be included directly within the string using the `${expression}` syntax, making string construction cleaner than traditional concatenation with `+`. * Multi-line Strings: Strings can span multiple lines directly within the backticks without needing concatenation or escape characters like `\n`. * Tagged Templates: An advanced feature allowing functions to parse template literals, enabling custom string processing (e.g., for internationalization or escaping HTML).
Further considerations highlight how template literals significantly improve the readability and maintainability of code involving string manipulation.
Template literals (also known as template strings) provide a more flexible and readable way to create strings compared to traditional single or double quotes.
- Syntax: Use backticks (`` ` ``) instead of quotes (`'` or `"`).
- String Interpolation: Easily embed expressions (variables, function results) directly into strings using the `${...}` placeholder. This is much cleaner than traditional string concatenation using the `+` operator.
- Multi-line Strings: Template literals allow strings to span multiple lines without needing special characters like `\n` or string concatenation for each line. The whitespace and line breaks inside the backticks are preserved.
- Tagged Templates (Advanced): You can prefix a template literal with a function name (a "tag"). This function receives the string parts and expression values, allowing for custom processing of the template literal before the final string is assembled.
// Traditional string concatenation
let firstName = "Qwirey";
let lastName = "User";
let traditionalGreeting = "Hello, " + firstName + " " + lastName + "!";
console.log(traditionalGreeting); // Output: Hello, Qwirey User!
// Template Literal with interpolation
let templateGreeting = `Hello, ${firstName} ${lastName}!`;
console.log(templateGreeting); // Output: Hello, Qwirey User!
// Multi-line string with template literal
let multiLine = `This is line one.
This is line two.
Indentation is preserved.`;
console.log(multiLine);
/* Output:
This is line one.
This is line two.
Indentation is preserved.
*/
// Example with expression embedding
let price = 10;
let tax = 0.05;
let message = `The total price is $${(price * (1 + tax)).toFixed(2)}.`;
console.log(message); // Output: The total price is $10.50.
5. Destructuring Assignment
This section introduces destructuring assignment, a powerful ES6 feature that allows extracting values from arrays or properties from objects into distinct variables concisely.
Objectively, destructuring provides a shorthand syntax for unpacking values from data structures directly into variables.
Delving deeper: * Array Destructuring: Extracts elements from an array based on their position. Allows skipping elements and using rest syntax (`...`) to gather remaining elements. * Object Destructuring: Extracts properties from an object based on their names. Allows assigning to variables with different names, providing default values, and nesting destructuring.
Further considerations highlight how destructuring makes code cleaner and more readable, especially when working with function parameters, configuration objects, or complex data structures.
Destructuring assignment is a convenient syntax that allows you to "unpack" values from arrays or properties from objects into distinct variables.
- Array Destructuring:
- Extracts values based on their position in the array.
- Syntax: `const [var1, var2, ...rest] = array;`
- Allows easily assigning array elements to variables, skipping elements, and gathering remaining elements using the rest syntax (`...`).
- Object Destructuring:
- Extracts values based on property names.
- Syntax: `const { prop1, prop2: newName, prop3 = defaultValue, ...rest } = object;`
- Allows easily assigning object properties to variables (using the same name or a new name), providing default values for properties that might be missing, and gathering remaining properties using the rest syntax (`...`).
- Very useful for extracting options from function arguments or properties from nested objects.
// Array Destructuring
const colors = ["red", "green", "blue", "yellow"];
const [firstColor, secondColor, , fourthColor] = colors;
console.log(firstColor); // Output: red
console.log(secondColor); // Output: green
console.log(fourthColor); // Output: yellow
const [head, ...tail] = colors;
console.log(head); // Output: red
console.log(tail); // Output: [ 'green', 'blue', 'yellow' ]
// Object Destructuring
const person = {
id: 1,
name: "Alice",
age: 30,
city: "Mirabel"
};
const { name, age, city: hometown = "Unknown" } = person;
console.log(name); // Output: Alice
console.log(age); // Output: 30
console.log(hometown); // Output: Mirabel (Uses property value; default ignored)
const { id, ...details } = person;
console.log(id); // Output: 1
console.log(details); // Output: { name: 'Alice', age: 30, city: 'Mirabel' }
// Useful in function parameters
function greet({ name = "Guest", age = "N/A" }) {
console.log(`Hello ${name}, age ${age}.`);
}
greet(person); // Output: Hello Alice, age 30.
greet({}); // Output: Hello Guest, age N/A.
6. Enhanced Function Parameters: Default & Rest
This section covers ES6 enhancements for defining function parameters, specifically default parameters and the rest parameter syntax.
Objectively, these features provide more flexibility and cleaner syntax for handling function arguments.
Delving deeper: * Default Parameters: Allows assigning default values to function parameters directly in the function signature. If an argument for that parameter is not provided (or is `undefined`) when the function is called, the default value is used. This simplifies code that previously required manual checks for undefined parameters. * Rest Parameter (`...`): Allows representing an indefinite number of arguments as an array. Must be the last parameter in the function signature. It gathers all remaining arguments passed to the function into a true array, replacing the older, array-like `arguments` object.
Further considerations highlight how these features make function definitions more expressive and reduce boilerplate code for handling optional or variable arguments.
ES6 introduced convenient ways to handle function parameters:
- Default Parameters:
- Allows you to specify default values for function parameters directly in the function definition.
- If a caller does not provide a value for that parameter (or passes `undefined`), the default value is automatically used.
- Syntax: `function myFunc(param1 = defaultValue1, param2 = defaultValue2) { ... }`
- Eliminates the need for boilerplate code inside the function to check for missing arguments and assign defaults (e.g., `param1 = param1 || defaultValue1;`).
- Rest Parameter (`...`):
- Allows a function to accept an indefinite number of arguments as an array.
- Syntax: `function myFunc(param1, param2, ...restArgs) { ... }`
- The `...restArgs` syntax gathers all remaining arguments passed to the function (beyond `param1` and `param2` in this example) into a real JavaScript array named `restArgs`.
- Must be the last parameter in the function definition.
- Provides a cleaner alternative to the older `arguments` object, which was array-like but not a true array.
// Default Parameters
function greetUser(name = "Guest", message = "Welcome") {
console.log(`${message}, ${name}!`);
}
greetUser("Alice", "Hello"); // Output: Hello, Alice!
greetUser("Bob"); // Output: Welcome, Bob!
greetUser(); // Output: Welcome, Guest!
// Rest Parameter
function sumAll(...numbers) {
// 'numbers' is a real array
return numbers.reduce((total, num) => total + num, 0);
}
console.log(sumAll(1, 2, 3)); // Output: 6
console.log(sumAll(10, 20, 30, 40)); // Output: 100
console.log(sumAll(5)); // Output: 5
7. Spread Operator (`...`)
This section explains the Spread Operator, another powerful ES6 feature using the same `...` syntax as the Rest Parameter, but used in different contexts to expand iterables.
Objectively, the Spread Operator allows an iterable (like an array or string) to be expanded into individual elements or properties, typically where multiple arguments or elements are expected.
Delving deeper into common use cases: * In Function Calls: Spreads array elements as individual arguments to a function (e.g., `Math.max(...numbers)`). * In Array Literals: Creates new arrays by combining existing arrays or adding elements (e.g., `[...arr1, ...arr2, newItem]`). Provides an easy way to clone arrays. * In Object Literals (ES2018+): Creates new objects by copying properties from existing objects, merging objects, or adding new properties (e.g., `{...obj1, ...obj2, newProp: value}`). Provides an easy way to clone or merge objects shallowly.
Further considerations distinguish it from the Rest Parameter: Spread *expands* elements *out* of an iterable, while Rest *gathers* elements *into* an array parameter.
The Spread Operator (`...`) uses the same syntax as the Rest Parameter but performs the opposite function: it *expands* an iterable (like an array, string, or object properties in later versions) into individual components.
- Expanding Arrays into Function Arguments:
- Pass elements of an array as individual arguments to a function.
- Expanding Arrays into Array Literals:
- Easily combine or clone arrays.
- Expanding Strings:
- Spread a string into individual characters (useful for creating an array of characters).
- Expanding Objects into Object Literals (ES2018 and later):
- Copy properties from one or more objects into a new object literal. Useful for merging objects or creating shallow clones. Properties from later objects overwrite earlier ones with the same key.
Key Distinction: Rest Parameter (`...`) collects multiple elements/arguments *into* a single array variable (used in function parameters). Spread Operator (`...`) expands elements *out* of an iterable into individual components (used in function calls, array literals, object literals).
// Spread in function calls
const nums = [1, 5, 2, 8, 3];
console.log(Math.max(...nums)); // Expands nums to Math.max(1, 5, 2, 8, 3) -> Output: 8
// Spread in array literals
const arr1 = [1, 2, 3];
const arr2 = [4, 5, 6];
const combined = [...arr1, 0, ...arr2];
console.log(combined); // Output: [ 1, 2, 3, 0, 4, 5, 6 ]
const clonedArr1 = [...arr1]; // Shallow clone
console.log(clonedArr1); // Output: [ 1, 2, 3 ]
console.log(clonedArr1 === arr1); // Output: false (different array instances)
// Spread in object literals (ES2018+)
const objA = { a: 1, b: 2 };
const objB = { b: 3, c: 4 };
const mergedObj = { ...objA, ...objB, d: 5 };
console.log(mergedObj); // Output: { a: 1, b: 3, c: 4, d: 5 } (objB.b overwrites objA.b)
const clonedObjA = { ...objA }; // Shallow clone
console.log(clonedObjA); // Output: { a: 1, b: 2 }
8. Classes: Cleaner Object-Oriented Syntax
This section introduces ES6 Classes, which provide a clearer and more familiar syntax for creating objects based on prototypes, aligning JavaScript closer to classical object-oriented languages.
Objectively, ES6 classes are primarily "syntactic sugar" over JavaScript's existing prototype-based inheritance mechanism. They do not introduce a new inheritance model but offer a cleaner way to define constructor functions and manage inheritance.
Delving deeper into class syntax: * `class` Keyword: Used to declare a class. * `constructor` Method: A special method for creating and initializing objects created with the class. * Methods: Defined directly within the class body. * `extends` Keyword: Used for creating subclasses that inherit from a parent class. * `super` Keyword: Used to call the parent class's constructor or methods.
Further considerations emphasize that understanding prototypes is still valuable for deeper JS knowledge, but classes offer a much more intuitive syntax for developers familiar with OOP concepts from other languages.
ES6 introduced the `class` syntax, providing a more structured and familiar way (especially for developers coming from languages like Java or C++) to create blueprints for objects and manage inheritance.
It's important to understand that ES6 classes are mainly syntactic sugar over JavaScript's existing prototype-based inheritance. They don't fundamentally change the underlying mechanism but offer a cleaner syntax.
- Class Declaration: Use the `class` keyword followed by the class name.
- `constructor` Method: A special method within a class for creating and initializing objects created with that class. It's called automatically when you use the `new` keyword.
- Methods: Define methods directly within the class body using shorthand syntax (e.g., `methodName() { ... }`). These methods become part of the class's prototype.
- Inheritance (`extends`): Use the `extends` keyword to create a subclass (child class) that inherits properties and methods from a parent class (superclass).
- `super` Keyword: Used within a subclass to call the constructor (`super()`) or methods (`super.methodName()`) of the parent class. `super()` must be called in the subclass constructor before using `this`.
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a noise.`);
}
}
class Dog extends Animal {
constructor(name, breed) {
super(name); // Call the parent constructor
this.breed = breed;
}
speak() { // Overrides parent method
console.log(`${this.name} barks.`);
}
describe() {
console.log(`${this.name} is a ${this.breed}.`);
}
}
const genericAnimal = new Animal("Creature");
genericAnimal.speak(); // Output: Creature makes a noise.
const dog = new Dog("Buddy", "Golden Retriever");
dog.speak(); // Output: Buddy barks.
dog.describe(); // Output: Buddy is a Golden Retriever.
9. Modules: Organizing Your Code
This section explains ES6 Modules, providing a standard, built-in system for organizing JavaScript code into reusable, separate files.
Objectively, ES6 modules allow developers to split code into smaller, manageable files (modules), exporting specific variables, functions, or classes from one module and importing them into others where needed.
Delving deeper into module syntax: * `export` Keyword: Used within a module file to make variables, functions, or classes available for use in other modules. Can be used for named exports (`export const name = ...;`) or a single default export (`export default ...;`). * `import` Keyword: Used in another module file to bring in exported members from a different module. Syntax varies for named imports (`import { name1, name2 } from './module.js';`), default imports (`import myDefault from './module.js';`), and namespace imports (`import * as myModule from './module.js';`).
Further considerations highlight how modules improve code organization, prevent global namespace pollution, manage dependencies explicitly, and are fundamental to building large-scale JavaScript applications. (Note: Module loading behavior depends on the environment - browser vs. Node.js).
Before ES6, JavaScript lacked a standardized native module system, leading to various community solutions (like CommonJS used in Node.js, or AMD). ES6 introduced a built-in system using the `import` and `export` keywords.
Modules allow you to:
- Break down large codebases into smaller, reusable files (modules).
- Encapsulate code: Variables and functions defined within a module are local to that module unless explicitly exported.
- Manage dependencies clearly: Explicitly import the specific functionality needed from other modules.
- Avoid polluting the global namespace.
Key Syntax:
- `export`: Place this keyword before a declaration (variable, function, class) within a module file (`.js` or `.mjs`) to make it available outside.
- Named Exports: `export const PI = 3.14; export function greet() {...}` (Can have multiple).
- Default Export: `export default class User {...}` (Only one per module).
- `import`: Use this keyword at the top of another module file to access exported members.
- Named Imports: `import { PI, greet } from './myMathModule.js';` (Use curly braces).
- Default Import: `import MyUserClass from './userModule.js';` (No curly braces).
- Namespace Import: `import * as MathUtils from './myMathModule.js';` (Imports everything into an object).
Note: How modules are loaded and used depends on the environment. Browsers typically require ``, while Node.js uses `.mjs` file extensions or specific `package.json` settings to enable ES modules.
// --- File: utils.js ---
export const apiKey = "abc123xyz";
export function logMessage(message) {
console.log(`LOG: ${message}`);
}
// --- File: main.js ---
import { apiKey, logMessage } from './utils.js';
// Assuming this runs in an environment supporting ES modules
console.log(`Using API Key: ${apiKey}`); // Output: Using API Key: abc123xyz
logMessage("Application started."); // Output: LOG: Application started.
10. Promises: Handling Asynchronous Operations
This section introduces Promises, an ES6 feature providing a more manageable way to handle asynchronous operations compared to traditional callbacks.
Objectively, a Promise represents the eventual result (either success or failure) of an asynchronous operation. It acts as a placeholder for a value that will be available later.
Delving deeper: * States: A Promise can be in one of three states: *pending* (initial state), *fulfilled* (operation completed successfully), or *rejected* (operation failed). * `.then()` Method: Used to schedule callbacks that execute when the Promise is fulfilled (receives the resolved value). * `.catch()` Method: Used to schedule callbacks that execute when the Promise is rejected (receives the reason for failure). * `.finally()` Method: Used to schedule callbacks that execute regardless of whether the Promise was fulfilled or rejected (useful for cleanup). * Chaining: Promises can be chained together using `.then()`, making complex asynchronous sequences easier to manage and read than nested callbacks ("callback hell").
Further considerations mention that Promises form the foundation for even newer asynchronous patterns like `async/await` (introduced in ES2017), which provide syntactic sugar over Promises for writing asynchronous code that looks more synchronous.
Asynchronous operations (like fetching data from a server, reading a file, or waiting for a timer) are common in JavaScript. Before ES6, these were often handled using callbacks, which could lead to deeply nested and hard-to-read code ("callback hell"). ES6 introduced Promises as a cleaner alternative.
- What is a Promise? A Promise is an object representing the eventual completion (or failure) of an asynchronous operation and its resulting value.
- States: A Promise starts in a *pending* state. It eventually transitions to either:
- *Fulfilled:* The operation completed successfully, and the Promise holds the resulting value.
- *Rejected:* The operation failed, and the Promise holds the reason for the failure (an error).
- Consuming Promises (`.then()`, `.catch()`, `.finally()`):
- Use the `.then(onFulfilled, onRejected)` method to attach callbacks. The first callback (`onFulfilled`) runs if the Promise fulfills, receiving the resolved value. The optional second callback (`onRejected`) runs if it rejects.
- Use the `.catch(onRejected)` method as shorthand for handling only rejections (`.then(undefined, onRejected)`).
- Use the `.finally(onFinally)` method to execute code once the Promise settles, regardless of outcome (useful for cleanup actions).
- Chaining: `.then()` and `.catch()` return new Promises, allowing you to chain asynchronous operations together in a readable sequence.
Promises provide a much better way to manage asynchronous code flow compared to traditional callbacks. They are also the basis for the more recent `async/await` syntax (ES2017), which makes asynchronous code look even more synchronous.
// Example: Using the Fetch API (which returns a Promise)
fetch('https://api.example.com/data') // Returns a Promise
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
return response.json(); // Returns another Promise
})
.then(data => {
console.log('Data received:', data);
})
.catch(error => {
console.error('Fetch failed:', error);
})
.finally(() => {
console.log('Fetch attempt finished.');
});
// Creating a simple Promise
const myPromise = new Promise((resolve, reject) => {
setTimeout(() => {
const success = true; // Simulate success/failure
if (success) {
resolve("Operation successful!"); // Fulfill the promise
} else {
reject("Operation failed!"); // Reject the promise
}
}, 1000);
});
myPromise
.then(message => console.log(message)) // Handles fulfillment
.catch(error => console.error(error)); // Handles rejection
10. Conclusion & Resources
This concluding section summarizes the impact of ES6 features and provides resources for further learning.
Objectively, ES6 (ECMAScript 2015) represented a major modernization of the JavaScript language, introducing features that are now indispensable for efficient, readable, and maintainable code.
Delving deeper, features like `let`/`const`, arrow functions, template literals, destructuring, classes, modules, and Promises address fundamental aspects of programming, making JavaScript development more productive and enjoyable.
Further considerations encourage developers to embrace these features in their projects and utilize authoritative resources like MDN Web Docs to deepen their understanding of modern JavaScript.
Conclusion: Embracing Modern JavaScript with ES6
ECMAScript 2015 (ES6) was a landmark release that fundamentally improved JavaScript development. Features like block-scoped variables (`let`, `const`), concise arrow functions with lexical `this`, powerful template literals, convenient destructuring, cleaner class syntax, native modules, and improved asynchronous handling with Promises are no longer just "new" – they are the foundation of modern JavaScript.
Mastering these ES6 features enables developers to write code that is not only more powerful but also significantly cleaner, more readable, and easier to maintain. As browsers and Node.js widely support ES6 and later versions, understanding these concepts is essential for anyone working with JavaScript today.
Key ES6 & JavaScript Resources
Primary Documentation & Learning:
- MDN Web Docs (Mozilla Developer Network): The definitive resource for JavaScript and web technologies. MDN JavaScript Reference / JavaScript Guide
- javascript.info: A comprehensive modern JavaScript tutorial. javascript.info
- Exploring ES6 (Book by Dr. Axel Rauschmayer): In-depth coverage of ES6 features (available online). exploringjs.com/es6/
Specification:
- ECMA-262 Standard (ECMAScript): The official language specification. tc39.es/ecma262/ (Technical and dense).
Practice & Community:
- freeCodeCamp: Interactive coding challenges and projects. freecodecamp.org
- Codewars / LeetCode: Platforms for practicing coding problems.
- Stack Overflow: Community Q&A for specific programming questions.
References (Placeholder)
Links to specific MDN pages for each feature could be included here.
- MDN: let, const
- MDN: Arrow functions
- MDN: Template literals
- MDN: Destructuring assignment
- MDN: Default parameters, Rest parameters
- MDN: Spread syntax
- MDN: Classes
- MDN: JavaScript modules
- MDN: Promise
ES6 Features Overview
(Placeholder: Simple graphic listing key ES6 features covered)
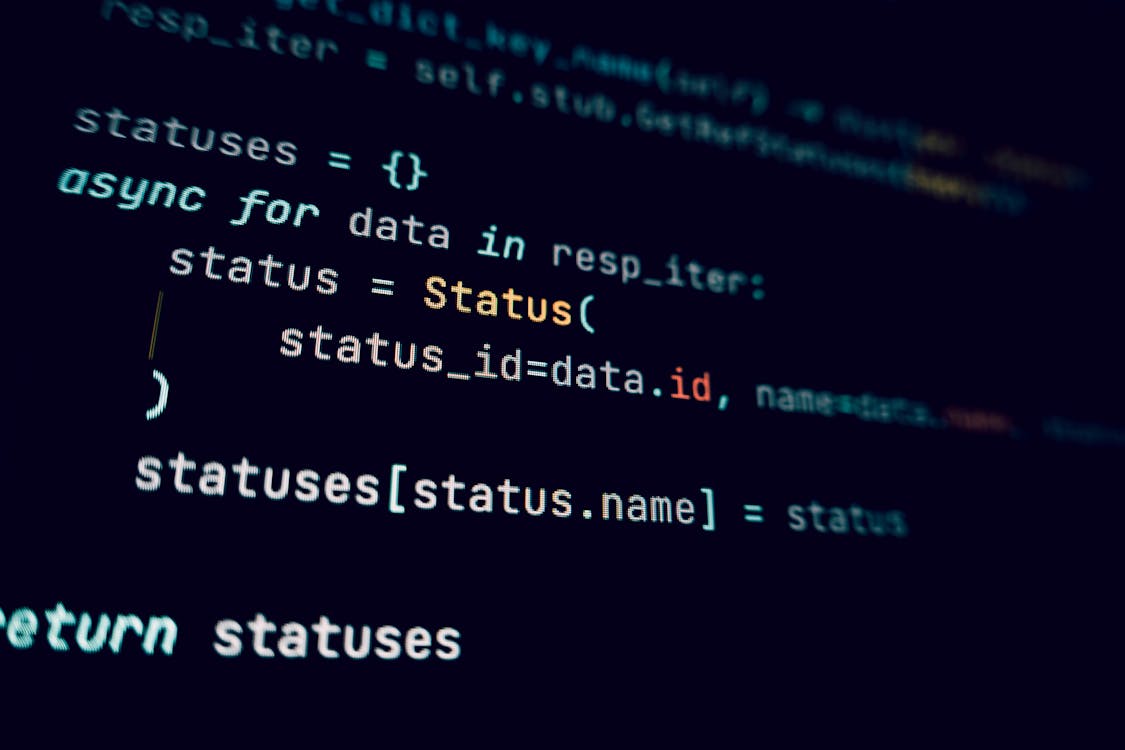